Boosting OpenCV Application Performance: Detailed Tips for Optimizing OpenCV Performance, from Code Optimization to Parallel Processing
发布时间: 2024-09-15 10:47:51 阅读量: 27 订阅数: 28 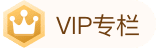
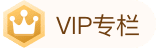
# 1. Overview of OpenCV Performance Optimization**
OpenCV (Open Source Computer Vision Library) is a powerful computer vision library extensively used in image processing, video analysis, and machine learning fields. However, as application scenarios become more complex, performance optimization of OpenCV programs becomes crucial.
This guide will delve into all aspects of OpenCV performance optimization, including code optimization techniques, parallel processing, hardware acceleration, performance analysis, and tuning strategies. By following these best practices, developers can significantly improve the performance of OpenCV programs, meeting requirements such as real-time processing and high throughput.
# 2. OpenCV Code Optimization Techniques
### 2.1 Data Structure Optimization
#### 2.1.1 Avoiding Unnecessary Copies
**Optimization Goal:** Reduce memory allocation and copy operations for improved program efficiency.
**Optimization Methods:**
- **Using References or Pointers:** Pass references or pointers to avoid copying entire objects.
- **Using Shared Memory:** Store data in shared memory to avoid multiple copies.
- **Using Views:** Create views of data without creating copies.
**Code Example:**
```cpp
// Avoid unnecessary copying
cv::Mat image1 = cv::imread("image.jpg");
cv::Mat image2 = image1.clone(); // A copy of the image
// Using a reference to avoid copying
cv::Mat& image2 = image1; // Referencing the image
```
**Logical Analysis:**
In the first example, `image2` is a copy of `image1`, requiring new memory allocation and data copying. In the second example, `image2` references `image1`, avoiding unnecessary copying.
#### 2.1.2 Using the Right Containers
**Optimization Goal:** Select appropriate container structures to enhance data access efficiency.
**Optimization Methods:**
- **Using Vectors:** Store contiguous data elements for fast random access.
- **Using Lists:** Store non-contiguous data elements for fast insertion and deletion.
- **Using Hash Tables:** Store key-value pairs for quick lookup operations.
**Code Example:**
```cpp
// Using a vector to store contiguous data
std::vector<int> numbers = {1, 2, 3, 4, 5};
// Using a list to store non-contiguous data
std::list<std::string> names = {"John", "Mary", "Bob"};
// Using a hash table to store key-value pairs
std::unordered_map<std::string, int> ages = {{"John", 25}, {"Mary", 30}, {"Bob", 35}};
```
**Logical Analysis:**
`std::vector` is used for storing contiguous integers, `std::list` for non-contiguous strings, and `std::unordered_map` for key-value pairs. These container structures provide optimal data access efficiency.
### 2.2 Algorithm Optimization
#### 2.2.1 Choosing Efficient Algorithms
**Optimization Goal:** Select algorithms with better time and space complexity to improve program performance.
**Optimization Methods:**
- **Using Quick Sort:** Time complexity of O(n log n) and space complexity of O(log n).
- **Using Hash Table Lookups:** Time complexity of O(1) and space complexity of O(n).
- **Using Binary Search:** Time complexity of O(log n) and space complexity of O(1).
**Code Example:**
```cpp
// Using quick sort
std::vector<int> numbers = {1, 5, 2, 4, 3};
std::sort(numbers.begin(), numbers.end());
// Using hash table lookups
std::unordered_map<std::string, int> ages = {{"John", 25}, {"Mary", 30}, {"Bob", 35}};
int age = ages["John"];
// Using binary search
std::vector<int> numbers = {1, 2, 3, 4, 5};
int index = std::binary_search(numbers.begin(), numbers.end(), 3);
```
**Logical Analysis:**
`std::sort` employs the quick sort algorithm, `std::unordered_map` uses hash table lookups, and `std::binary_search` uses the binary search algorithm, providing superior time and space complexity.
#### 2.2.2 Reducing Unnecessary Computations
**Optimization Goal:** Avoid redundant calculations to improve program efficiency.
**Optimization Methods:**
- **Using Cachin
0
0
相关推荐
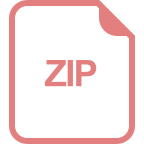
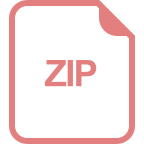
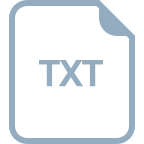
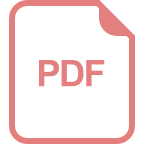
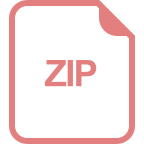
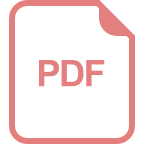
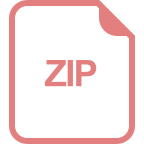
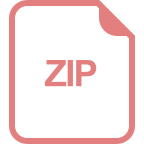
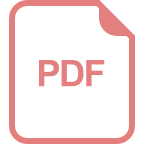