OpenCV Deep Learning Practical Guide: From Image Classification to Object Detection, Building AI Applications
发布时间: 2024-09-15 10:31:14 阅读量: 7 订阅数: 12 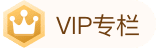
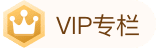
# 1. Introduction to OpenCV Deep Learning
OpenCV (Open Source Computer Vision Library) is a powerful open-source library for computer vision, widely used for image and video processing, machine learning, and deep learning applications. In the realm of deep learning, OpenCV offers a rich set of functions and modules that make it easy for developers to construct and deploy deep learning models.
The OpenCV deep learning module integrates popular deep learning frameworks such as TensorFlow, PyTorch, and Caffe. It provides a collection of pre-trained models for common tasks like image classification, object detection, and semantic segmentation. Additionally, OpenCV offers tools for data preprocessing, model training, and evaluation, streamlining the deep learning development workflow.
# 2. Image Classification in Practice
### 2.1 Image Preprocessing and Data Augmentation
#### 2.1.1 Image Scaling and Cropping
Image scaling and cropping are common techniques in image preprocessing used to adjust images to the size and aspect ratio required for model training.
```python
import cv2
# Load image
image = cv2.imread("image.jpg")
# Scale image
scaled_image = cv2.resize(image, (224, 224))
# Crop image
cropped_image = cv2.resize(image, (224, 224), interpolation=cv2.INTER_AREA)
```
**Parameter Explanation:**
- `cv2.resize()`: Used for scaling the image. The first parameter is the original image, and the second is the target size.
- `interpolation`: Specifies the scaling algorithm. `cv2.INTER_AREA` is used for downscaling, while `cv2.INTER_CUBIC` is for upscaling.
**Code Logic Analysis:**
1. `cv2.imread()` reads the image and stores it in the `image` variable.
2. `cv2.resize()` scales the image to `(224, 224)` size and stores it in the `scaled_image` variable.
3. `cv2.resize()` crops the image to `(224, 224)` size and stores it in the `cropped_image` variable.
#### 2.1.2 Data Augmentation Techniques
Data augmentation is a technique that generates more training samples by transforming the original data. It helps prevent overfitting of the model and improves its generalization ability.
**Common data augmentation techniques include:**
- **Random Cropping**: Randomly crop out regions of different sizes and positions from the image.
- **Random Flipping**: Horizontally or vertically flip the image.
- **Random Rotation**: Rotate the image by a random angle.
- **Color Jittering**: Adjust the brightness, contrast, and saturation of the image.
```python
import cv2
import numpy as np
# Load image
image = cv2.imread("image.jpg")
# Random cropping
random_crop = cv2.resize(image[np.random.randint(0, image.shape[0] - 224), np.random.randint(0, image.shape[1] - 224):], (224, 224))
# Random flipping
random_flip = cv2.flip(image, 1)
# Random rotation
random_rotation = cv2.rotate(image, cv2.ROTATE_90_CLOCKWISE)
# Color jittering
random_color = cv2.cvtColor(image, cv2.COLOR_BGR2HSV)
random_color[:, :, 1] = random_color[:, :, 1] * (0.8 + np.random.rand(1))
random_color[:, :, 2] = random_color[:, :, 2] * (0.8 + np.random.rand(1))
random_color = cv2.cvtColor(random_color, cv2.COLOR_HSV2BGR)
```
**Parameter Explanation:**
- `np.random.randint()`: Generates a random integer used for random cropping and flipping.
- `cv2.rotate()`: Rotates the image. `cv2.ROTATE_90_CLOCKWISE` represents a 90-degree clockwise rotation.
- `cv2.cvtColor()`: Converts the image's color space. `cv2.COLOR_BGR2HSV` converts from BGR to HSV color space, while `cv2.COLOR_HSV2BGR` converts from HSV to BGR.
**Code Logic Analysis:**
1. `cv2.imread()` reads the image and stores it in the `image` variable.
2. `np.random.randint()` generates two random integers used for random cropping. `cv2.resize()` scales the cropped image to `(224, 224)` size and stores it in the `random_crop` variable.
3. `cv2.flip()` horizontally flips the image and stores it in the `random_flip` variable.
4. `cv2.rotate()` rotates the image 90 degrees clockwise and stores it in the
0
0
相关推荐
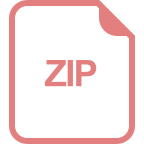
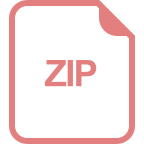
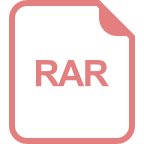





