Achieving Precise Image Alignment: A Comprehensive Analysis of OpenCV Image Registration Algorithms, from SIFT to ORB
发布时间: 2024-09-15 10:36:47 阅读量: 31 订阅数: 28 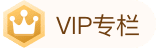
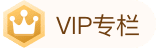
# Image Registration Overview
Image registration is a computer vision technique that aligns two or more images to overlap them spatially. Image registration is crucial in many applications such as image stitching, image rectification, and medical imaging.
Image registration algorithms typically rely on feature point detection and matching. Feature points are regions in an image with unique and repeatable patterns. By detecting and matching these feature points, algorithms can determine the geometric transformation between two images, thus achieving image registration.
The performance of image registration algorithms is influenced by various factors, including image quality, the robustness of feature point detection algorithms, and the efficiency of matching algorithms. When selecting an image registration algorithm, these factors and the requirements of specific applications must be considered.
# Feature-based Image Registration Algorithms
### Scale-Invariant Feature Transform (SIFT)
#### SIFT Algorithm Principle
SIFT (Scale-Invariant Feature Transform) is a feature-based image registration algorithm that is robust to changes in image scale, rotation, and lighting. The principles of the SIFT algorithm mainly include the following steps:
- **Image Pyramid Construction:** Scale the image to different levels to form an image pyramid, which allows the detection of feature points at various scales.
- **Feature Point Detection:** Use the Difference of Gaussian operator to detect extremas in each level of the image as candidate feature points.
- **Feature Point Localization:** Subpixel-level accurate positioning of candidate feature points to improve their accuracy.
- **Feature Point Orientation Assignment:** Calculate the gradients around each feature point and assign the dominant orientation based on the gradient direction.
- **Feature Point Description:** Compute the gradient histogram in the area around the feature point to form a feature point descriptor.
#### SIFT Algorithm Implementation
```python
import cv2
def sift(image1, image2):
# Initialize SIFT feature detector
sift = cv2.SIFT_create()
# Detect feature points
keypoints1, descriptors1 = sift.detectAndCompute(image1, None)
keypoints2, descriptors2 = sift.detectAndCompute(image2, None)
# Feature point matching
bf = cv2.BFMatcher()
matches = bf.knnMatch(descriptors1, descriptors2, k=2)
# Filter matches
good_matches = []
for m, n in matches:
if m.distance < 0.75 * n.distance:
good_matches.append(m)
# Draw matches
result = cv2.drawMatches(image1, keypoints1, image2, keypoints2, good_matches, None)
return result
```
**Parameter Explanation:**
- `image1` and `image2`: The two images to be registered.
- `k`: The number of nearest neighbor matches in the matching algorithm.
**Code Logic Analysis:**
1. Initialize the SIFT feature detector.
2. Use SIFT to detect feature points and descriptors in both images.
3. Perform feature point matching using the Brute Force Matcher (BFMatcher).
4. Filter matches to remove erroneous matches.
5. Draw matches for visualizing the registration results.
### Oriented FAST and Rotated BRIEF (ORB)
#### ORB Algorithm Principle
ORB (Oriented FAST and Rotated BRIEF) is a fast and robust feature point detection and description algorithm that is based on the FAST feature point detector and the BRIEF descriptor. The principles of the ORB algorithm mainly include the following steps:
- **Feature Point Detection:** Use the FAST algorithm to detect feature points in the image.
- **Feature Point Description:** Use the BRIEF algorithm to calculate the binary pattern of the pixels around the feature points to form feature point descriptors.
- **Feature Point Matching:** Use the Hamming distance to calculate the similarity between feature point descriptors for matching.
#### ORB Algorithm Implementation
```python
import cv2
def orb(image1, image2):
# Initialize ORB feature detector
orb = cv2.ORB_create()
# Detect feature points
keypoints1, descriptors1 = orb.detectAndCompute(image1, None)
keypoints2, descriptors2 = orb.detectAndCompute(image2, None)
# Feature point matching
bf = cv2.BFMatcher(cv2.NORM_HAMMING, crossCheck=True)
matches = bf.match(descriptors1, descriptors2)
# Draw matches
result = cv2.drawMatches(image1, keypoints1, image2, keypoints2, mat
```
0
0
相关推荐
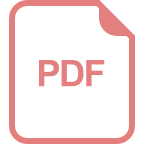
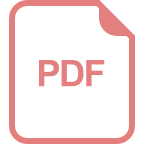
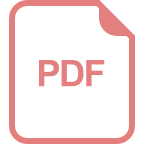





