Make OpenCV Omnipresent: A Detailed Guide to OpenCV Mobile Development, from iOS to Android
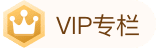
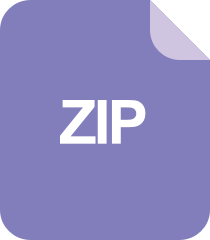
opencv-tutorial-a-guide-to-learn-opencv
1. Overview of OpenCV Mobile Development
1.1 Introduction to OpenCV
OpenCV (Open Source Computer Vision Library) is an open-source library that provides a wide array of algorithms and functions for image processing, computer vision, and machine learning. It is extensively applied in various fields, including mobile device development.
1.2 Advantages of OpenCV Mobile Development
*Cross-Platform Support: OpenCV supports multiple mobile platforms, such as iOS and Android, allowing developers to build cross-platform applications with ease. *Powerful Functionality: OpenCV offers a wealth of algorithms for image processing, computer vision, and machine learning, enabling developers to create robust mobile applications. *Community Support: OpenCV has a vast community that provides documentation, tutorials, and examples to help developers get up to speed quickly.
2. Fundamentals of OpenCV Mobile Development
2.1 Integration and Configuration of OpenCV Library
2.1.1 iOS Platform Integration
Steps:
- Add the OpenCV library to your Xcode project:
- Open your Xcode project, select “File” > “Add Files” > “Add Files to [Project Name]”.
- Navigate to the location of the OpenCV library, select the “libopencv_ios.a” file, and click “Add”.
- Add Header Files:
- In your project build settings, go to “Build Settings” > “Header Search Paths”, and add the path to the OpenCV header files.
- Link the Library:
- In your project build settings, go to “Build Settings” > “Linking” > “Other Linker Flags”, and add the “-lopencv_ios” flag.
Code Snippet:
- // Import OpenCV header file in AppDelegate.swift
- import OpenCV
- // Use OpenCV function in ViewController.swift
- let image = Mat(rows: 100, cols: 100, type: CvType.CV_8UC3)
Logical Analysis:
import OpenCV
imports the OpenCV header file.let image = Mat(...)
creates a new Mat object to store image data.
2.1.2 Android Platform Integration
Steps:
- Add the OpenCV library to your Android Studio project:
- Right-click on the project and select “File” > “Project Structure”.
- In the “Project” tab, select “Dependencies” > “+” > “Library dependency”.
- Search for “OpenCV”, choose the “opencv” library, and click “OK”.
- Add Header Files:
- In the “app” module of your project, create a folder named “jni”.
- Copy OpenCV header files (e.g., opencv2/opencv.hpp) into the “jni” folder.
- Modify CMakeLists.txt ***
*** “jni” folder, open the CMakeLists.txt file.
- Add the following lines:
- find_library(OPENCV_LIBRARY opencv)
- target_link_libraries(opencv_java ${OPENCV_LIBRARY})
- Add the following lines:
Code Snippet:
- // Import OpenCV header file in MainActivity.java
- import org.opencv.android.OpenCVLoader;
- // Load OpenCV library in the onCreate() method
- if (!OpenCVLoader.initDebug()) {
- Log.e("MainActivity", "OpenCV initialization failed!");
- }
- // Use OpenCV function
- Mat image = new Mat(100, 100, CvType.CV_8UC3);
Logical Analysis:
import org.opencv.android.OpenCVLoader
imports the OpenCV header file.OpenCVLoader.initDebug()
initializes the OpenCV library.Mat image = new Mat(...)
creates a new Mat object to store image data.
2.2 Setting Up the Mobile Development Environment
2.2.1 Configuration of Xcode and Android Studio
Xcode:
- Install Xcode version 13 or higher.
- Install iOS SDK version 15 or higher.
- Install OpenCV version 4.5 or higher.
Android Studio:
- Install Android Studio version 4.2 or higher.
- Install Android SDK version 30 or higher.
- Install OpenCV version 4.5 or higher.
2.2.2 Selection of OpenCV Library Versions
The choice of OpenCV version depends on the target platform and development environment:
Platform | OpenCV Version |
---|---|
iOS | OpenCV 4.5.5 or higher versions |
Android | OpenCV 4.5.5 or higher versions |
Table: Selection of OpenCV Library Versions
Mermaid Flowchart:
graph LR
subgraph iOS
Xcode 13+
iOS SDK 15+
OpenCV 4.5+
end
subgraph Android
Android Studio 4.2+
Android SDK 30+
OpenCV 4.5+
end
Flowchart Analysis:
- Both iOS and Android platforms require specific software and OpenCV versions to be installed for mobile development.
- The flowchart illustrates the dependencies for each platform.
3. OpenCV Mobile Development in Practice
3
相关推荐







