Building Cross-Platform OpenCV Applications: A Comprehensive Guide to Cross-Platform Development with OpenCV, from Windows to Linux
发布时间: 2024-09-15 10:46:57 阅读量: 30 订阅数: 32 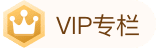
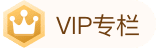
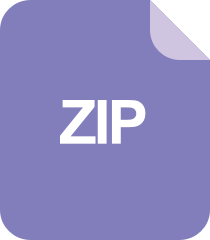
Cross-Platform-Dense-SLAM:由Keller等人提出的密集SLAM的跨平台实现。 使用C ++,OpenGL和OpenCV
# 1. Overview of OpenCV and Introduction to Cross-Platform Development
OpenCV (Open Source Computer Vision Library) is an open-source computer vision library that offers a variety of image processing and computer vision algorithms. It supports cross-platform development, allowing developers to build and run applications on different operating systems.
Cross-platform development is a development strategy that enables applications to run on multiple operating systems without requiring specialized development for each operating system. This saves development time and cost and ensures consistent behavior of applications across different platforms. OpenCV supports cross-platform development by providing cross-platform APIs and libraries.
# 2. Setting Up a Cross-Platform OpenCV Development Environment
### 2.1 Setting Up the Windows Environment
#### 2.1.1 Installing Visual Studio
- Download and install Visual Studio 2022 or a higher version.
- Ensure that the C++ workload for desktop development is installed.
#### 2.1.2 Installing OpenCV
- Download the latest version of OpenCV and choose the installer for Windows.
- Run the installer and follow the prompts.
- Make sure to add OpenCV to the system path.
#### 2.1.3 Configuring Environment Variables
- Open the control panel and search for "environment variables."
- Add the following variable in "System variables":
```
Variable name: OPENCV_DIR
Variable value: OpenCV installation directory
```
- Add the following variable in "User variables":
```
Variable name: Path
Variable value: %OPENCV_DIR%\bin;%Path%
```
### 2.2 Setting Up the Linux Environment
#### 2.2.1 Installing Dependencies
- Update the package manager:
```
sudo apt update
```
- Install necessary dependencies:
```
sudo apt install build-essential cmake pkg-config libgtk2.0-dev libavcodec-dev libavformat-dev libswscale-dev
```
#### 2.2.2 Installing OpenCV
- Download the latest version of OpenCV and choose the installer for Linux.
- Unpack the installation package and enter the unpacked directory:
```
tar -xzvf opencv-latest.tar.gz
cd opencv-latest
```
- Compile and install OpenCV:
```
mkdir build
cd build
cmake -D CMAKE_BUILD_TYPE=Release -D CMAKE_INSTALL_PREFIX=/usr/local ..
make
sudo make install
```
#### 2.2.3 Configuring Environment Variables
- Open the terminal and add the following variables:
```
export OPENCV_DIR=/usr/local/share/OpenCV
export LD_LIBRARY_PATH=$OPENCV_DIR/lib:$LD_LIBRARY_PATH
```
### 2.3 Selecting and Configuring a Cross-Platform IDE
#### 2.3.1 CLion
- CLion is a cross-platform C/C++ IDE that supports OpenCV development.
- Install CLion and install the OpenCV plugin.
- Create a new OpenCV project and configure the following settings:
```
CMakeLists.txt:
cmake_minimum_required(VERSION 3.18)
project(opencv_project)
find_package(OpenCV REQUIRED)
target_link_libraries(opencv_project ${OpenCV_LIBRARIES})
```
#### 2.3.2 Visual Studio Code
- Visual Studio Code is a free and open-source code editor that supports OpenCV development.
- Install Visual Studio Code and install the C/C++ extension.
- Install the OpenCV extension and configure the following settings:
```
settings.json:
{
"C_Cpp.default.includePath": ["${env:OPENCV_DIR}/include"]
}
```
#### 2.3.3 CMake
- CMake is a cross-platform build system that can be used to configure and build OpenCV projects.
- Create a CMakeLists.txt file and configure the following settings:
```
cmake_minimum_required(VERSION 3.18)
project(opencv_project)
find_package(OpenCV REQUIRED)
target_link_libraries(opencv_project ${OpenCV_LIBRARIES})
```
- Use the following commands to build the project:
```
cmake .
make
```
# 3. OpenCV Cross-Platform Development in Practice
### 3.1 Developing Cross-Platform Image Processing Applications
#### 3.1.1 Image Reading and Display
**Reading an Image**
```cpp
cv::Mat image = cv::imread("image.jpg");
```
**Parameter Explanation:**
* `image.jpg`: The path to the image file to be read.
**Logical Analysis:**
The `cv::imread` function reads the specified image file and stores it in a `cv::Mat` object.
**Displaying an Image**
```cpp
cv::imshow("Image", image);
cv::waitKey(0);
```
**Parameter Explanation:**
* `Image`: The title of the image window.
* `image
0
0
相关推荐
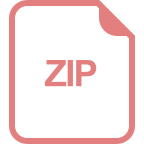
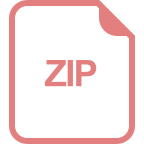
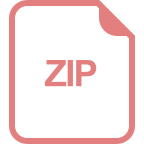
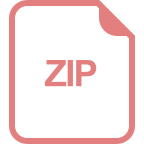
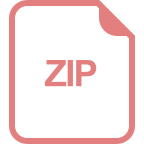
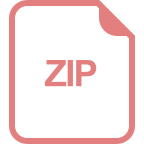
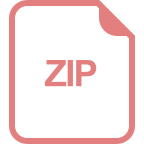
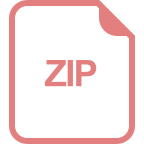