Demystifying Object Detection: An In-depth Analysis of OpenCV Object Detection Algorithms, from Haar Cascades to YOLO
发布时间: 2024-09-15 10:34:41 阅读量: 22 订阅数: 24 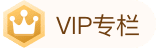
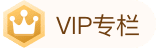
# Demystifying Object Detection: A Comprehensive Analysis of OpenCV Object Detection Algorithms, from Haar Cascades to YOLO
# 1. Object Detection Overview
Object detection is a crucial task in computer vision, aiming to localize and identify interesting objects within images or videos. Object detection algorithms typically encompass two steps:
1. **Feature Extraction:** Extract features representing targets from images, such as shape, texture, and color.
2. **Classification and Localization:** Classify the extracted features into specific target categories and determine the position of the objects within the image.
# 2. Traditional Object Detection Algorithms
### 2.1 Haar Cascade Classifiers
#### 2.1.1 Theoretical Basis
The Haar cascade classifier is a machine learning algorithm based on Haar features for object detection. Haar features are the differences in adjacent rectangular regions in an image and can effectively capture edge and texture information in images.
The Haar cascade classifier detects objects through a series of weak classifiers trained to recognize a target. Each weak classifier uses a single Haar feature to classify image regions as either objects or non-objects based on the value of the feature. These weak classifiers are cascaded to form a strong classifier, capable of more accurately detecting targets.
#### 2.1.2 Practical Application
Haar cascade classifiers are widely used in face detection, pedestrian detection, and other fields. Their advantages include:
- Fast training speed
- Fast detection speed
- Strong robustness, insensitive to changes in lighting and poses
### 2.2 HOG Pedestrian Detector
#### 2.2.1 Theoretical Basis
The Histogram of Oriented Gradients (HOG) pedestrian detector is a machine learning algorithm based on gradient histograms for pedestrian detection. The gradient histogram describes the distribution of gradient directions and magnitudes at each pixel in the image.
The HOG pedestrian detector calculates the gradient histogram of each local area in the image and uses a Support Vector Machine (SVM) for classification of these histograms. SVM is a binary classification algorithm that can classify local areas as pedestrians or non-pedestrians.
#### 2.2.2 Practical Application
HOG pedestrian detectors are widely used in pedestrian detection, vehicle detection, and other fields. Their advantages include:
- High detection accuracy
- Strong robustness, insensitive to changes in lighting and poses
- High computational efficiency
**Code Block:**
```python
import cv2
# Load HOG pedestrian detector
hog = cv2.HOGDescriptor()
hog.setSVMDetector(cv2.HOGDescriptor_getDefaultPeopleDetector())
# Load image
image = cv2.imread('image.jpg')
# Detect pedestrians
(rects, weights) = hog.detectMultiScale(image, winStride=(4, 4), padding=(8, 8), scale=1.05)
# Draw detection boxes
for (x, y, w, h) in rects:
cv2.rectangle(image, (x, y), (x + w, y + h), (0, 255, 0), 2)
# Display image
cv2.imshow('Image', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**Logical Analysis:**
* `cv2.HOGDescriptor()`: Create a HOG descriptor object.
* `hog.setSVMDetector()`: Load the default pedestrian detector.
* `cv2.imread()`: Load the image.
* `hog.detectMultiScale()`: Use the HOG descriptor to detect pedestrians.
* `cv2.rectangle()`: Draw the detection box.
* `cv2.imshow()`: Display the image.
* `cv2.waitKey()`: Wait for user input.
* `cv2.destroyAllWindows()`: Destroy all windows.
**Parameter Explanation:**
* `winStride`: The stride of the sliding window.
* `padding`: Padding around the image.
* `scale`: The image scaling factor.
# 3. Deep Learning-Based Object Detection Algorithms**
**3.1 R-CNN Series Algorithms**
**3.1.1 Theoretical Basis**
The R-CNN (Region-based Convolutional Neural Networks) series algorithms are pioneering work in deep learning-based object detection algorithms. They divide the object detection problem into two steps:
1. **Region Proposal:** Generate candidate target regions using selective search or other algorithms.
2. **Feature Extraction and Classification:** Extract features from each candidate region and classify the region using a convolutional neural network.
The advantage of the R-CNN algorithm is that it can leverage the powerful feature extraction capabilities of deep neural networks, thereby improving the accuracy of object detection. However, it also has some disadvantages, including:
* High c
0
0
相关推荐
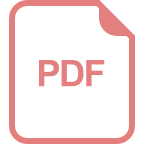
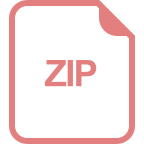
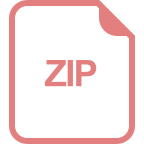
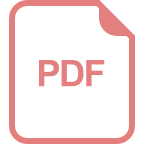
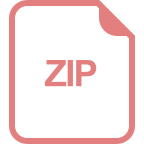
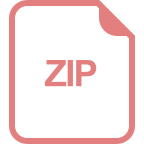
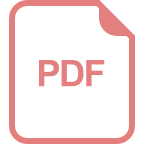
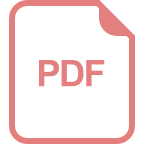
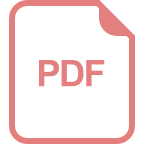