Object Recognition in Images: A Detailed Explanation of OpenCV Image Recognition Algorithms, from Feature Extraction to Deep Learning
发布时间: 2024-09-15 10:35:56 阅读量: 40 订阅数: 39 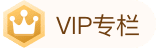
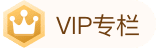
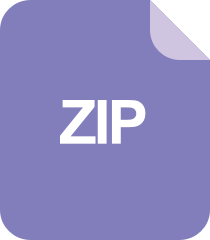
Object Detection and Recognition Using Deep Learning in OpenCV [Chapter 1 and 2]

# 1. Overview of Image Recognition
Image recognition is a significant technology in the field of computer vision, aiming to enable computers to "see" and "understand" images as humans do. It involves extracting meaningful information from images, such as objects, faces, and scenes. Image recognition plays a crucial role in various applications, including security, medicine, industrial automation, and entertainment.
Image recognition algorithms are typically divided into two categories: traditional algorithms and deep learning algorithms. Traditional algorithms rely on manually designed features and rules, whereas deep learning algorithms utilize neural networks to automatically learn features from data. In recent years, deep learning algorithms have made significant advancements in image recognition tasks and have become the dominant technology in this field.
# 2. Basics of OpenCV Image Recognition Algorithms
### 2.1 Basics of Image Processing
Image processing is the foundation of computer vision, performing various operations on images to enhance image quality and extract valuable information for subsequent image recognition algorithms. OpenCV provides a wealth of image processing functions, covering image enhancement, image segmentation, and image feature extraction.
#### 2.1.1 Image Enhancement
Image enhancement aims to improve the visual effects and readability of images, making them more suitable for subsequent processing. OpenCV offers a variety of image enhancement techniques, including:
- **Histogram Equalization:** Adjusts the histogram of the image to distribute different gray levels more evenly, enhancing image contrast.
- **Gamma Correction:** Adjusts the gamma value of the image to alter its brightness and contrast.
- **Sharpening:** Enhances the contrast of edges and details in the image, making it clearer.
#### 2.1.2 Image Segmentation
Image segmentation breaks an image into sub-regions with different features for easier analysis and recognition. OpenCV provides several image segmentation algorithms, including:
- **Thresholding:** Segments the image into a binary image based on pixel gray values.
- **Region Growing:** Starts from a seed point and aggregates pixels with similar features into a region.
- **Watershed Algorithm:** Treats the image as terrain, assigning pixels to different basins, thus achieving segmentation.
#### 2.1.3 Image Feature Extraction
Image feature extraction extracts valuable information from images to provide a basis for subsequent image recognition algorithms. OpenCV offers various image feature extractors, including:
- **Edge Detection:** Detects edges and contours in an image.
- **Corner Detection:** Detects corners and interest points in an image.
- **Histogram:** Statistics of the distribution of different gray levels in the image.
### 2.2 Basics of Machine Learning
Machine learning is the core of image recognition algorithms, enabling computers to learn from data and make predictions and decisions. OpenCV integrates various machine learning algorithms, covering supervised learning, unsupervised learning, feature selection, and dimensionality reduction.
#### 2.2.1 Supervised Learning and Unsupervised Learning
- **Supervised Learning:** Learns from labeled data to build models to predict labels for new data.
- **Unsupervised Learning:** Learns from unlabeled data to discover potential structures and patterns.
#### 2.2.2 Feature Selection and Dimensionality Reduction
- **Feature Selection:** Selects the most discriminative and relevant features from raw data, reducing computation and improving model performance.
- **Dimensionality Reduction:** Projects high-dimensional data into a low-dimensional space, reducing data redundancy and improving computational efficiency.
#### 2.2.3 Classification and Regression
- **Classification:** Assigns data points to predefined categories.
- **Regression:** Predicts continuous values, such as the size or position of objects in an image.
# 3. Practical Application of OpenCV Image Recognition Algorithms
### 3.1 Traditional Image Recognition Algorithms
Traditional image recognition algorithms are manual feature engineering algorithms based on image processing and machine learning technologies. These algorithms typically include steps such as image enhancement, image segmentation, image feature extraction, and classification or regression.
#### 3.1.1 Template Matching
Template matching is an image recognition algorithm that matches based on the sub-region of an image and a template image. The template image is typically a known image of the target object. The algorithm detects the target object by sliding the template image over the input image and calculating the similarity between the template image and sub-regions of the input image. The sub-region with the highest similarity is considered the matching area for the target object.
```python
import cv2
# Load template image and input image
template = cv2.imread('template.jpg', cv2.IMREAD_GRAYSCALE)
image = cv2.imread('input.jpg', cv2.IMREAD_GRAYSCALE)
# Template Matching
result = cv2.matchTemplate(image, template, cv2.TM_CCOEFF_NORMED)
# Find the matching region
min_val, max_val, min_loc, max_loc = cv2.minMaxLoc(result)
top_left = max_loc
# Draw the matching region
cv2.rectangle(image, top_left, (top_left[0] + template.shape[1], top_left[1] + template.shape[0]), (0, 255, 0), 2)
# Display the resu
```
0
0