Java最短路径算法时间复杂度与优化方向
发布时间: 2024-08-29 23:33:27 阅读量: 52 订阅数: 27 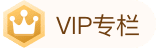
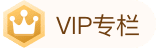
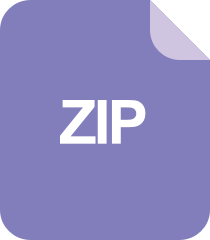
毕业设计 最短路径算法.zip
# 1. 最短路径问题概述
## 1.1 定义与背景
最短路径问题是图论中的一个经典问题,主要关注在加权图中找到两个顶点之间的最短路径。这个问题不仅在理论计算机科学领域有着广泛的研究,也是许多实际应用的基石,如导航系统、网络路由和交通规划等。
## 1.2 实际意义
解决最短路径问题对于优化资源分配、减少运输成本和提高效率至关重要。在现实世界中,最短路径算法能够帮助我们更好地理解复杂网络中的动态,进而做出更快速和有效的决策。
## 1.3 算法发展
随着时间的推移,多种最短路径算法相继被提出,它们各自有不同的适用场景、复杂度和优化方向。从经典的Dijkstra算法到现代的A*算法,每一项创新都在推动着该领域的发展。
在本文的后续章节中,我们将深入探讨这些算法的理论基础、实现细节、实践应用以及优化策略,希望能为读者提供最短路径问题的全面理解。
# 2. 经典最短路径算法理论
## 2.1 Dijkstra算法
### 2.1.1 基本原理与步骤
Dijkstra算法是一种用于图的单源最短路径问题的算法,能够找到从单一源点到其他所有节点的最短路径。该算法于1956年由荷兰计算机科学家Edsger W. Dijkstra提出,是计算机网络中用于路由算法的基础。
算法基本步骤如下:
1. 初始化源点的距离为0,其他所有节点的距离为无穷大,并将所有节点标记为未访问。
2. 创建一个包含所有节点的未访问集合。
3. 从未访问集合中选择距离源点最近的节点,将其标记为已访问。
4. 更新当前节点的邻居节点的距离。如果从源点经过当前节点到达邻居节点的距离更短,则更新该距离。
5. 重复步骤3和步骤4,直到所有节点都被访问。
### 2.1.2 时间复杂度分析
Dijkstra算法的时间复杂度依赖于所使用的数据结构。如果使用优先队列(如二叉堆),时间复杂度可以优化至O((V+E)logV),其中V是节点数,E是边数。这是因为每次从优先队列中取出最小元素的操作代价是O(logV),而更新所有节点的优先级的操作代价是O(E)。因此,总的时间复杂度是两者乘积的对数。
## 2.2 Bellman-Ford算法
### 2.2.1 算法描述与特性
Bellman-Ford算法用于在包含负权边的图中寻找从单个源点到所有其他节点的最短路径。该算法比Dijkstra算法更为通用,但是它的时间复杂度较高,为O(VE)。
算法的基本步骤如下:
1. 初始化源点的距离为0,其他所有节点的距离为无穷大。
2. 对所有的边进行V-1次松弛操作(Relaxation),即遍历每条边,尝试通过这条边减小到其邻接点的距离。
3. 检查是否存在负权回路。通过再次尝试松弛操作,如果某条边仍然可以减小距离,则存在负权回路。
### 2.2.2 时间复杂度及限制
Bellman-Ford算法的时间复杂度为O(VE),这是因为每条边可能会被松弛V-1次,而图中有E条边。由于需要多次遍历所有边,所以对于大型稀疏图,该算法可能不如Dijkstra算法高效。
该算法的限制在于它不适用于包含负权回路的图,因为它不能正确计算出负权回路中的路径长度。如果在步骤3中检测到负权回路,则算法会报告这一点并停止执行。
## 2.3 Floyd-Warshall算法
### 2.3.1 算法原理与步骤
Floyd-Warshall算法是一个用于多源最短路径问题的算法,能够找出图中任意两点间的最短路径。与Dijkstra算法和Bellman-Ford算法相比,Floyd-Warshall算法可以处理包含负权边的图,但不能处理包含负权回路的图。
算法的基本步骤如下:
1. 初始化一个距离矩阵D,其中D[i][j]表示从节点i到节点j的边的权重,如果i和j之间没有直接的边,则设置为无穷大。
2. 对每一个中间节点k,对距离矩阵D进行更新。如果通过中间节点k从i到j的路径比直接的i到j的路径更短,则更新D[i][j]。
3. 重复步骤2,直到所有的中间节点都被考虑过。
### 2.3.2 时间复杂度及适用场景
Floyd-Warshall算法的时间复杂度是O(V^3),这使得它在处理大型图时效率较低。然而,它的优势在于它能够处理包含多个源点的最短路径问题,并且实现相对简单。
适用场景包括:
- 图的规模较小且需要计算所有节点对之间的最短路径时。
- 需要频繁查询任意两点间的最短路径时。
- 图中边的权重可能会变化时,因为Floyd-Warshall算法可以很容易地重新计算整个图的最短路径。
在下一章节中,我们将探讨这些算法在实践中的具体实现和优化策略,以便更好地应用于各种实际问题中。
# 3. 最短路径算法实践应用
在这一章节中,我们将深入探讨最短路径算法在实际编程中的应用,以及如何通过代码实现和优化这些算法。本章重点关注三个经典算法——Dijkstra算法、Bellman-Ford算法和Floyd-Warshall算法,并通过Java语言实现这些算法,同时探讨在实际场景中可能遇到的挑战和优化策略。
## 3.1 Dijkstra算法实现与优化
### 3.1.1 Java实现细节
Dijkstra算法是解决单源最短路径问题的常用算法。以下是Dijkstra算法的基本Java实现:
```java
import java.util.Arrays;
import java.util.PriorityQueue;
public class DijkstraAlgorithm {
private static final int NO_PARENT = -1;
public static void dijkstra(int[][] adjacencyMatrix, int startVertex) {
int nVertices = adjacencyMatrix[0].length;
// shortestDistances[i] will hold the shortest distance from start to i
int[] shortestDistances = new int[nVertices];
// added[i] will be true if vertex i is included in shortest path tree
boolean[] added = new boolean[nVertices];
// Initialize all distances as INFINITE and added[] as false
for (int vertexIndex = 0; vertexIndex < nVertices; vertexIndex++) {
shortestDistances[vertexIndex] = Integer.MAX_VALUE;
added[vertexIndex] = false;
}
// Distance of start vertex from itself is always 0
shortestDistances[startVertex] = 0;
// Parent array to store shortest path tree
int[] parents = new int[nVertices];
// The starting vertex does not have a parent
parents[startVertex] = NO_PARENT;
// Find shortest path for all vertices
for (int i = 1; i < nVertices; i++) {
// Pick the minimum distance vertex from the set of vertices not yet processed
// u is always equal to startVertex in first iteration
int nearestVertex = -1;
int shortestDistance = Integer.MAX_VALUE;
for (int vertexIndex = 0; vertexIndex < nVertices; vertexIndex++) {
if (!added[vertexIndex] && shortestDistances[vertexIndex] < shortestDistance) {
nearestVertex = vertexIndex;
shortestDistance = shortestDistances[vertexIndex];
}
}
// Mark the picked vertex as processed
added[nearestVertex] = true;
// Update dist value of the adjacent vertices of the picked vertex
for (int vertexIndex = 0; vertexIndex < nVertices; vertexIndex++) {
int edgeDistance = adjacencyMatrix[nearestVertex][vertexIndex];
if (edgeDistance > 0 && ((shortestDistance + edgeDistance) < shortestDistances[vertexIndex])) {
parents[vertexIndex] = nearestVertex;
shortestDistances[vertexIndex] = shortestDistance + edgeDistance;
}
}
}
printSolution(startVertex, shortestDistances, parents);
}
// A utility function to print the constructed distances array and shortest paths
private static void printSolution(int startVertex, int[] distances, int[] parents) {
int nVertices = distances.length;
System.out.print("Vertex\t Distance\tPath");
for (int vertexIndex = 0; vertexIndex < nVertices; vertexIndex++) {
if (vertexIndex != startVertex) {
System.out.print("\n" + startVertex + " -> ");
System.out.print(vertexIndex + " \t\t ");
System.out.print(distances[vertexIndex] + "\t\t");
printPath(vertexIndex, parents);
}
}
}
// Function to print shortest path from source to currentVertex using parents array
private static void printPath(int currentVertex, int[] parents) {
if (currentVertex == NO_PARENT) {
return;
}
printPath(parents[currentVertex], parents);
System.out.print(currentVertex + " ");
}
public static void main(String[] args) {
int[][] adjacencyMatrix = {
{ 0, 4, 0, 0, 0, 0, 0, 8, 0 },
{ 4, 0, 8, 0, 0, 0, 0, 11, 0 },
{ 0, 8, 0, 7, 0, 4, 0, 0, 2 },
{ 0, 0, 7, 0, 9, 14, 0, 0, 0 },
{ 0, 0, 0, 9, 0, 10, 0, 0, 0 },
{ 0, 0, 4, 14, 10, 0, 2, 0, 0 },
{ 0, 0, 0, 0, 0, 2, 0, 1, 6 },
{ 8, 11, 0, 0, 0, 0, 1, 0, 7 },
{ 0, 0, 2, 0, 0, 0, 6, 7, 0 }
};
dijkstra(adjacencyMatrix, 0);
}
}
```
### 3.1.2 优化策略与实例
Dijkstra算法的时间复杂度为O(V^2),使用优先队列可以将其降低到O((V+E)logV),其中V是顶点数,E是边数。以下是使用Java优先队列优化后的实现:
```java
import java.util.PriorityQueue;
class Vertex {
int vertexNumber;
int distance;
boolean visited;
Vertex parent;
public Vertex(int v, int dist) {
vertexNumber = v;
distance = dist;
visited = false;
}
public void setDistance(int dist) {
distance = dist;
}
public int getDistance() {
return distance;
}
public int getVertexNumber() {
return vertexNumber;
}
public void setVertexNumber(int vertexNumber) {
this.vertexNumber = vertexNumber;
}
public boolean isVisited() {
return visited;
}
public void setVisited(boolean visited) {
this.visited = visited;
}
public Vertex getParent() {
return parent;
}
public void setParent(Vertex parent) {
this.parent = parent;
}
}
public class DijkstraOptimized {
private void dijkstra(int[][] adjacencyMatrix, int startVertex) {
int nVertices = adjacencyMatrix[0].length;
Vertex[] vertices = new Vertex[nVertices];
// Initialize vertices
for (int i = 0; i < nVertices; i++) {
vertices[i] = new Vertex(i, Integer.MAX_VALUE);
}
vertices[startVertex].setDistance(0);
```
0
0
相关推荐
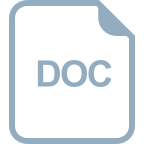
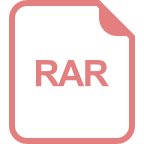
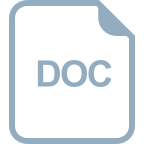
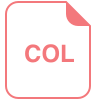
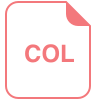
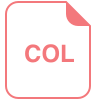
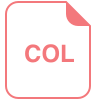
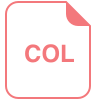