网络流与最大流最小割定理:Java最短路径问题应用剖析
发布时间: 2024-08-29 23:24:36 阅读量: 61 订阅数: 24 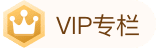
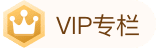
.png)
# 1. 网络流与最大流最小割定理基础
网络流是图论和网络分析中一个极其重要的概念,它是研究网络中数据流动效率以及资源分配问题的基础工具。在这一章节中,我们将探索网络流模型的基础知识和最大流最小割定理的基本概念。
## 1.1 网络流基本概念
网络流是指在一个有向图中,从源点到汇点的流,这些流必须满足流守恒和容量限制两个基本条件。流守恒指的是网络中任意一个非源点、非汇点的节点,流入该节点的流量之和等于流出该节点的流量之和。容量限制则表示每条边上的流量不能超过这条边的容量上限。
## 1.2 最大流问题
最大流问题要求我们在满足流量守恒和容量限制的前提下,找到从源点出发到汇点的流量最大的可能路径。解决这个问题可以通过多种算法,如Ford-Fulkerson方法、Edmonds-Karp算法和Dinic算法等。每种算法都有其特定的适用场景和效率。
## 1.3 最小割定理
最小割定理是网络流理论的核心定理之一,它指出一个网络的最大流值等于其最小割容量。最小割是指在某个网络中,将源点和汇点分隔开来的边的最小容量和。这一原理是网络流算法优化的基础,它提供了一种评判和指导最大流算法性能的新视角。
# 2. 图论中的最短路径算法理论
### 2.1 最短路径问题概述
#### 定义与重要性
最短路径问题是在一个加权图中寻找两个顶点之间权值总和最小的路径。这个问题在交通规划、网络通信、物流配送等多个领域有着广泛的应用。例如,在公路网络中,我们可能希望找到从城市A到城市B的最短行驶路线,以节省时间和燃油。在计算机网络中,寻找数据包从源地址到目的地址的最短路径是实现高效路由的关键。
最短路径问题的求解对于优化网络资源分配、降低运营成本具有重要意义。此外,最短路径算法在路径规划、游戏设计、社交网络分析等领域也扮演着重要角色。
#### 算法分类与应用场景
最短路径算法主要分为两类:单源最短路径算法和多源最短路径算法。单源最短路径算法用于计算图中某个顶点到其他所有顶点的最短路径,而多源最短路径算法则计算图中任意两个顶点之间的最短路径。
单源最短路径算法例如Dijkstra算法和Bellman-Ford算法,在很多领域内都有应用,比如在社交网络中分析用户间的最短关系链。多源最短路径算法如Floyd-Warshall算法和Johnson算法,它们在构建路由表或进行全局网络分析时非常有用。
### 2.2 单源最短路径算法
#### Dijkstra算法原理与实现
Dijkstra算法是一种基于贪心策略的单源最短路径算法,适用于没有负权边的加权图。算法从源点开始,逐步扩展最短路径树,直到覆盖所有顶点。
算法的基本步骤如下:
1. 初始化源点到所有顶点的距离为无穷大,源点自身为0。
2. 创建一个未处理的顶点集合,初始时仅包含源点。
3. 当未处理的顶点集合非空时,选择一个距离源点最近的顶点u,并从集合中移除。
4. 更新顶点u的每个邻居v的距离,如果从源点经过顶点u到达顶点v的路径更短,则更新距离值。
5. 重复步骤3和4,直到所有顶点都被处理。
以下是Dijkstra算法的伪代码实现:
```pseudo
function dijkstra(graph, source):
dist[source] ← 0 // 初始化源点距离
for each vertex v in graph: // 初始化所有顶点
if v ≠ source
dist[v] ← INFINITY // 最大值表示无穷大
prev[v] ← UNDEFINED // 前驱节点设置为未定义
Q.add_with_priority(v, dist[v]) // 优先队列
while Q is not empty: // 主循环
u ← Q.extract_min() // 弹出最小距离顶点
for each neighbor v of u: // 遍历u的邻接顶点
alt ← dist[u] + length(u, v)
if alt < dist[v]: // 如果找到更短路径
dist[v] ← alt // 更新距离
prev[v] ← u // 更新前驱节点
return dist[], prev[]
```
在Java中,可以使用`PriorityQueue`实现上述算法,以保持未处理顶点集合的最小优先级队列特性。
#### Bellman-Ford算法原理与实现
Bellman-Ford算法同样适用于没有负权环的加权图,并能处理负权边的情况。相比Dijkstra算法,Bellman-Ford算法在每次迭代中会考虑所有边,逐步缩短路径长度。
算法步骤如下:
1. 初始化源点到其他所有顶点的距离为无穷大,源点自身为0。
2. 对所有边进行`|V|-1`次松弛操作(每次迭代检查所有边)。
3. 检查是否存在负权环,再次对所有边进行松弛操作,如果没有距离减少,则不存在负权环。
以下是Bellman-Ford算法的伪代码实现:
```pseudo
function bellman_ford(graph, source):
dist[source] ← 0 // 初始化源点距离
for each vertex v in graph: // 初始化其他所有顶点
if v ≠ source
dist[v] ← INFINITY // 最大值表示无穷大
for i from 1 to |V|-1: // 进行|V|-1次松弛操作
for each edge (u, v) in graph.edges: // 遍历每条边
if dist[u] + length(u, v) < dist[v]
dist[v] ← dist[u] + length(u, v)
for each edge (u, v) in graph.edges: // 检查负权环
if dist[u] + length(u, v) < dist[v]
error "Graph contains a negative-weight cycle"
return dist[]
```
在Java实现中,可以通过双重循环实现上述步骤,并在第二次循环中检测负权环。
### 2.3 多源最短路径算法
#### Floyd-Warshall算法原理与实现
Floyd-Warshall算法是一种动态规划算法,用于计算图中所有顶点对之间的最短路径。算法初始化一个距离矩阵,并逐步更新矩阵中的元素,直到找到所有顶点对之间的最短路径。
算法步骤如下:
1. 初始化距离矩阵,对角线元素为0,表示顶点到自身的距离为0,其他为无穷大或两个顶点间的实际距离。
2. 对每个顶点k,作为中间顶点,更新距离矩阵中的元素`dist[i][j]`,如果通过顶点k的路径比直接路径更短,则更新`dist[i][j]`。
以下是Floyd-Warshall算法的伪代码实现:
```pseudo
function floyd_warshall(graph):
dist[][] ← INFINITY // 初始化距离矩阵
for each vertex v in graph: // 初始化对角线元素
dist[v][v] ← 0
for each edge (u, v) in graph.edges: // 初始化其他元素
dist[u][v] ← length(u, v)
for k from 1 to |V|: // 通过顶点k更新距离
for i from 1 to |V|:
for j from 1 to |V|:
if dist[i][k] + dist[k][j] < dist[i][j]
dist[i][j] ← dist[i][k] + dist[k][j]
return dist[]
```
在Java实现时,可以通过三层嵌套循环来实现距离矩阵的动态规划更新。
#### Johnson算法原理与实现
Johnson算法结合了Dijkstra算法和Bellman-Ford算法的优点,适用于包含负权边但没有负权环的图。它先通过添加一个虚拟源点和一条连接所有顶点的边,并为每条边分配一个初始权重,然后使用Bellman-Ford算法找到最短路径,接着重新调整边的权重,最后用Dijkstra算法找到每个顶点到其他顶点的最短路径。
Johnson算法步骤如下:
1. 向图中添加一个虚拟源点s,并连接所有其他顶点,边的权重设置为0。
2. 使用Bellman-Ford算法计算从s到所有顶点的最短路径,以确定实际的边权重。
3. 重新计算每条边的权重,使得每条边的权重都是正的,同时保持所有路径长度不变。
4. 对于每个顶点v,使用Dijkstra算法计算从v到所有其他顶点的最短路径。
以下是Johnson算法的伪代码实现:
```pseudo
function johnson(graph):
s ← new vertex() // 添加虚拟源点
add edge (s, v) with weight 0 for all v in graph
h[] ← bellman_ford(graph, s) // 计算初始权重
for each edge (u, v) in graph.edges:
w(u, v) ← w(u, v) + h[u] - h[v] // 重新计算权重
for each vertex v in graph: // 使用Dijkstra算法
dist[v] ← dijkstra(graph, v)
remove vertex s and all edges to/from s // 移除虚拟源点和相关边
return dist[]
```
在Java中,首先使用Bellman-Ford算法计算每个顶点的潜在权重,然后根据这些权重调整每条边的权重,最后对每个顶点运行Dijkstra算法。
### 小结
最短路径算法是图论中的经典问题,不仅理论基础扎实,而且在实际应用中具有广泛的需求。本章节介绍了最短路径问题的定义、重要性以及算法分类。详细阐述了两类主要算法——单源最短路径算法和多源最短路径算法,并分别对Dijkstra算法、Bellman-Ford算法、Floyd-Warshall算法和Johnson算法的原理和实现进行了详细分析。这些算法为解决现实世界中的各种路径优化问题提供了有力的工具。
# 3. Java实现最短路径算法实践
## 3.1 Java环境下图的表示与操作
### 3.1.1 邻接矩阵与邻接表
在图论中,图可以通过多种数据结构来表示,其中最常用的两种数据结构
0
0
相关推荐
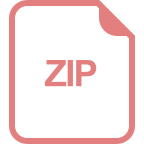
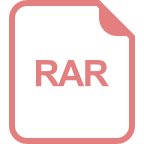
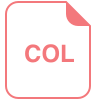
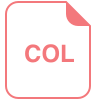
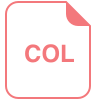
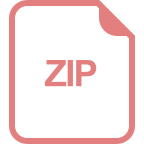
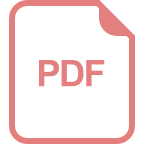
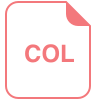
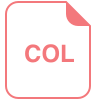