Introduction and Advanced: Teaching Resources for Monte Carlo Simulation in MATLAB
发布时间: 2024-09-15 10:23:22 阅读量: 6 订阅数: 10 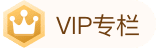
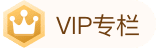
# Introduction and Advancement: Teaching Resources for Monte Carlo Simulation in MATLAB
## 1. Introduction to Monte Carlo Simulation
Monte Carlo simulation is a numerical simulation technique based on probability and randomness used to solve complex or intractable problems. It generates a large number of random samples and estimates the unknown quantities based on the results of the samples, thereby obtaining an approximate solution to the problem.
The advantages of Monte Carlo simulation include:
***Broad Applicability:** It can be used to solve a variety of problems, including integration, optimization, risk assessment, and financial modeling.
***Ease of Implementation:** Approximate solutions can be obtained by simply generating random numbers and performing straightforward calculations.
***Accuracy:** As the number of samples increases, the accuracy of the approximate solutions also improves.
## 2. Theory of Monte Carlo Simulation in MATLAB
### 2.1 The Basics of Monte Carlo Method
The Monte Carlo method is a numerical method based on random numbers for solving complex problems. It estimates the results by generating a large number of random samples and calculating their average. The principle of this method is that if the sample size is large enough, the average of the samples will be close to the true solution of the problem.
### 2.2 Probability Distributions and Random Number Generation
In Monte Carlo simulation, probability di***mon probability distributions include normal distribution, uniform distribution, exponential distribution, and Poisson distribution.
MATLAB provides various functions to generate random numbers, including:
```matlab
% Generating normally distributed random numbers
x = randn(1, 1000);
% Generating uniformly distributed random numbers
y = rand(1, 1000);
% Generating exponentially distributed random numbers
z = exprnd(1, 1, 1000);
```
### 2.3 Monte Carlo Integration and Optimization
Monte Carlo integration is a method that uses random numbers to estimate the value of an integral. It estimates the integral by generating a large number of random points and calculating the function values within the integral interval.
Monte Carlo optimization is a method that uses random numbers to search for the optimal value of a function. It estimates the optimal value by generating a large number of random points and calculating their function values.
**Code Block: Monte Carlo Integration**
```matlab
% Defining the integration function
f = @(x) sin(x);
% Integration interval
a = 0;
b = pi;
% Sample size
n = 10000;
% Generating random samples
x = a + (b - a) * rand(n, 1);
% Calculating function values
y = f(x);
% Estimating the integral value
integral = (b - a) * mean(y);
```
**Logical Analysis:**
This code uses Monte Carlo integration to estimate the integral value of the function `f(x) = sin(x)` over the interval `[0, pi]`. It first defines the integration function `f`, then generates `n` random samples `x`. Next, it calculates the function values `y` for these samples and uses the average to estimate the integral value.
**Code Block: Monte Carlo Optimization**
```matlab
% Defining the objective function
f = @(x) x^2 + 2*x + 1;
% Search range
lower = -5;
upper = 5;
% Sample size
n = 10000;
% Generating random samples
x = lower + (upper - lower) * rand(n, 1);
% Calculating function values
y = f(x);
% Finding the minimum function value
[min_value, min_index] = min(y);
% Optimal value
optimal_value = x(min_index);
```
**Logical Analysis:**
This code uses Monte Carlo optimization to search for the minimum value of the function `f(x) = x^2 + 2x + 1`. It first defines the objective function `f`, then generates `n` random samples `x`. Next, it calculates the function values `y` for these samples and finds the minimum function value `min_value` and the corresponding optimal value `optimal_value`.
# 3. Practice of Monte Carlo Simulation in MATLAB
### 3.1 Implementation of Monte Carlo Integration
Monte Carlo integration is the application of Monte Carlo method in numerical integration. It estimates the integral value by generating random samples and calculating their average.
**Steps:**
1. **Generating random samples:** Generate a set of random samples based on the integration interval and probability distribution.
2. **Calculating function values:** Calculate the value of the integrand for each random sample.
3. **Calculating the average:** Sum all function values and divide b
0
0
相关推荐
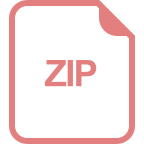
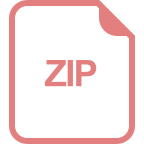






