Performance and Accuracy Evaluation: Algorithm Comparison of Monte Carlo Simulation in MATLAB
发布时间: 2024-09-15 10:19:59 阅读量: 19 订阅数: 23 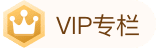
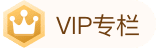
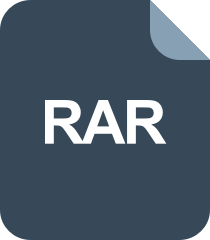
Monte-Carlo-Simulation-Area-of-the-Shape.rar_monte carlo methods
# Introduction to Monte Carlo Simulation
Monte Carlo Simulation is a numerical method based on random numbers to solve complex problems, especially those that are difficult to analyze or compute integrals and optimization problems. It approximates the expected value or other statistical measures of the target function by generating a large number of random samples and conducting statistical analysis on them.
The principle of Monte Carlo Simulation is based on the law of large numbers, which states that as the number of samples increases, the sample mean will converge to the expected value of the target function. Therefore, by generating a sufficient number of random samples, we can obtain an approximate value of the expected value of the target function.
# Implementation of Monte Carlo Simulation Algorithm in MATLAB
### 2.1 Pseudo-random Number Generation
#### 2.1.1 Random Number Generators
MATLAB offers various random number generators to produce pseudo-random numbers. Pseudo-random numbers are a series of numbers generated by algorithms that appear to be random, ***mon random number generators in MATLAB include:
- `rand()`: Generates pseudo-random numbers from a uniform distribution.
- `randn()`: Generates pseudo-random numbers from a normal distribution.
- `randperm()`: Generates a random permutation.
```matlab
% Generate 10 pseudo-random numbers from a uniform distribution
rand_numbers = rand(1, 10);
% Generate 10 pseudo-random numbers from a normal distribution
normal_numbers = randn(1, 10);
% Generate a random permutation of 1-10
random_permutation = randperm(10);
```
#### 2.1.2 Random Number Distributions
MATLAB also ***mon random number distributions include:
- Uniform distribution: `unifrnd()`
- Normal distribution: `normrnd()`
- Exponential distribution: `exprnd()`
- Poisson distribution: `poissrnd()`
```matlab
% Generate 10 pseudo-random numbers uniformly distributed between 0 and 1
uniform_numbers = unifrnd(0, 1, 1, 10);
% Generate 10 pseudo-random numbers from a normal distribution with mean 0 and standard deviation 1
normal_numbers = normrnd(0, 1, 1, 10);
% Generate 10 pseudo-random numbers from an exponential distribution with mean 1
exponential_numbers = exprnd(1, 1, 10);
```
### 2.2 Integration Computation
#### 2.2.1 Basic Principle
Monte Carlo integration is an integration method based on random sampling. The integral of a function f(x) defined on the interval [a, b] can be computed using the following formula:
```matlab
∫[a, b] f(x) dx ≈ (b - a) * (1/N) * Σ[i=1:N] f(x_i)
```
Where N is the number of random samples, and x_i are the sample points randomly drawn from the interval [a, b].
#### 2.2.2 Algorithm Steps
The steps of the Monte Carlo integration algorithm in MATLAB are as follows:
1. Define the integration interval [a, b] and the integrand function f(x).
2. Randomly generate N sample points x_i.
3. Calculate the function value f(x_i) for each sample point.
4. Calculate the approximate integral value:
```matlab
integral_approx = (b - a) * (1/N) * sum(f(x_i));
```
```matlab
% Define the integration interval and the integrand function
a = 0;
b = 1;
f = @(x) x.^2;
% Randomly generate 1000 sample points
N = 1000;
x_i = a + (b - a) * rand(1, N);
% Calculate the approximate integral value
integral_approx = (b - a) * (1/N) * sum(f(x_i));
% Output the approximate integral value
disp(integral_approx);
```
### 2.3 Solving Optimization Problems
#### 2.3.1 Optimization Algorithms
The Monte Carlo simulation algorithm can be used to solve optimization problems, ***mon optimization algorithms include:
- Random search: Randomly generate sample points and evaluate the objective function values, choosing the sample point with the largest objective function value.
- Simulated annealing: Start from a random initial point, gradually decrease the temperature, and accept or reject new sample points according to the Metropolis-Hastings criterion.
- Genetic algorithm: Simulate the process of biological evolution, generating new sample points through selection, crossover, and mutation operations.
```matlab
% Define the objective function
objective_function = @(x) -x.^2 + 2*x;
% Random search optimization
N = 1000;
max_value = -inf;
for i = 1:N
x = a + (b - a) * rand();
value = objective_function(x);
if value > max_value
max_value = value;
x_opt = x;
end
end
% Output the optimal solution
disp(['Optimal solution: ', num2str(x_opt)]);
disp(['Optimal value: ', num2str(max_value)]);
```
# Performance Evaluation of the Monte Carlo Simulation Algorithm
### 3.1 Algorithm Efficiency Analysis
#### 3.1.1 Time Complexity
The time complexity of the Monte Carlo Simulation algorithm mainly depends on the number of simulations and the computational complexity of each simulation step. For the integral calculation problem, each simulation step involves function evaluation, with a complexity of O(1). For the optimization problem solution, each simulation step involves the calculation of the objective function, with a complexity of O(n), where n is the number o
0
0
相关推荐
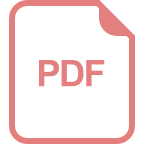
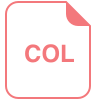
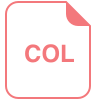
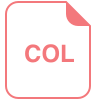
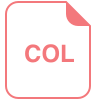
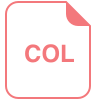
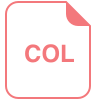
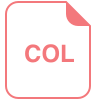