Performance Optimization: Accelerating Monte Carlo Simulations in MATLAB
发布时间: 2024-09-15 10:13:32 阅读量: 24 订阅数: 28 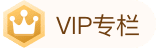
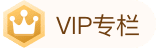
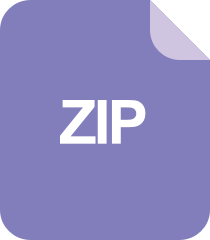
Particle Swarm Optimization:粒子群优化动画-matlab开发
# Performance Optimization: Accelerating Monte Carlo Simulations in MATLAB
## 1. Theoretical Foundations of Monte Carlo Simulation
Monte Carlo simulation is a numerical method based on probability and random numbers to solve complex problems. Its core idea is to approximate the expected value or other statistical measures of a target function through extensive random sampling.
The basic steps involved in Monte Carlo simulation are:
***Random Number Generation:** Generate random numbers according to given probability distributions to represent uncertainties in the model.
***Sampling and Statistics:** Sample from the random numbers and compute the target function values. Then perform statistical analysis on the sampling results to obtain an approximate distribution of the target function.
## 2. Implementation of Monte Carlo Simulation in MATLAB
### 2.1 Basic Steps of Monte Carlo Simulation
Monte Carlo simulation is a numerical method based on random number generation and statistical analysis. The basic steps include:
#### 2.1.1 Random Number Generation
Monte Carlo simulation requires generating a large number of random numbers to simulate real-world randomness. MATLAB provides various functions for generating random numbers, including:
```matlab
randn() % Generates random numbers from a standard normal distribution
rand() % Generates random numbers from a uniform distribution
normrnd(mu, sigma) % Generates random numbers from a normal distribution, with mu as the mean and sigma as the standard deviation
```
#### 2.1.2 Sampling and Statistics
Monte Carlo simulation estimates the value of a target function through sampling and statistical analysis of random numbers. Sampling involves selecting representative samples from the random numbers, while statistical analysis refers to the statistical calculations performed on the samples, such as mean, variance, etc.
### 2.2 Functions and Tools for Monte Carlo Simulation in MATLAB
MATLAB offers a comprehensive set of functions and tools to support Monte Carlo simulation, including:
#### 2.2.1 randn, rand, and normrnd Functions
As mentioned above, these functions are used to generate random numbers from different distributions.
#### 2.2.2 hist and histogram Functions
These functions are used to plot histograms of random numbers to help analyze their distribution.
```matlab
% Generating 1000 normally distributed random numbers
data = normrnd(0, 1, 1000, 1);
% Plotting histogram
histogram(data);
xlabel('Value');
ylabel('Frequency');
title('Histogram of Normally Distributed Random Numbers');
```
**Code Logic Analysis:**
* `normrnd(0, 1, 1000, 1)` generates 1000 normally distributed random numbers with a mean of 0 and a standard deviation of 1.
* `histogram(data)` plots the histogram of the random numbers in `data`.
* `xlabel('Value')` and `ylabel('Frequency')` set the axis labels.
* `title('Histogram of Normally Distributed Random Numbers')` sets the title of the chart.
## 3. Performance Optimization of Monte Carlo Simulation in MATLAB
### 3.1 Vectorization and Parallelization
#### 3.1.1 Vectorized Operations
Vectorized operations involve converting loop operations into vector operations to improve code efficiency. In MATLAB, vectorized functions can be used for this purpose. For instance, the following code uses a loop to compute the probability density function of a normal distribution:
```matlab
x = linspace(-3, 3, 1000);
mu = 0;
sigma = 1;
pdf = zeros(size(x));
for i = 1:length(x)
pdf(i) = 1 / (sigma * sqrt(2 * pi)) * exp(-(x(i) - mu)^2 / (2 * sigma^2));
end
```
The loop can be replaced with the vectorized function `normpdf`:
```matlab
pdf = normpdf(x, mu, sigma);
```
Vectorized operations can significantly enhance code efficiency by avoiding the overhead of loops.
#### 3.1.2 Parallel Computing
Parallel computing involves utilizing multiple processors or cores to execute tasks simultan
0
0
相关推荐







