Extended Monte Carlo Simulation: Integration with Other Tools in MATLAB
发布时间: 2024-09-15 10:16:30 阅读量: 24 订阅数: 30 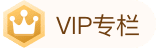
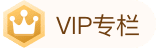
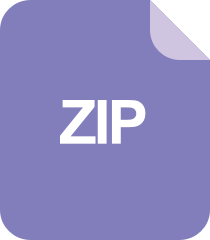
Heston Simulation using Monte Carlo:Heston Simulation using Monte Carlo-matlab开发
# Extended Monte Carlo Simulation: Integration with Other Tools in MATLAB
## 1. Basics of Monte Carlo Simulation
Monte Carlo simulation is a numerical technique based on random sampling to solve complex problems that are often difficult to solve using analytical methods. The core idea is to estimate the distribution or expected value of an unknown quantity by generating a large number of random samples and calculating the output value for each sample.
In Monte Carlo simulation, random samples are generated according to known probability distributions. By repeatedly sampling these samples, we can obtain an approximate value of the target distribution. The advantage of this method is that it does not require any assumptions about the target distribution and can be applied to a variety of problems.
## 2. Extended Monte Carlo Simulation in MATLAB
### 2.1 Parallel Computing in MATLAB
MATLAB provides extensive parallel computing features that can significantly improve the efficiency of Monte Carlo simulations.
#### 2.1.1 Multicore Parallelization
MATLAB supports multicore parallelization, allowing tasks to be executed simultaneously on multiple cores within a single computer. This can be achieved by using `parfor` loops, which distribute tasks to different cores.
```matlab
% Create an array of 1,000,000 random numbers
n = 1000000;
x = rand(n, 1);
% Use a parallel for loop to compute the sine value of each random number
tic;
parfor i = 1:n
y(i) = sin(x(i));
end
toc;
```
**Logical Analysis:**
This code uses a `parfor` loop to parallelize the computation of the `sin` function across multiple cores. The `tic` and `toc` functions are used to measure the time taken for the parallelized computation.
**Parameter Description:**
* `n`: The number of random numbers
* `x`: Array of random numbers
* `y`: Array of sine values
#### 2.1.2 GPU Acceleration
MATLAB also supports GPU acceleration, allowing computations to be executed on a graphics processing unit (GPU). GPUs have a large number of parallel processing units, which can significantly increase the speed of computation.
```matlab
% Create an array of 1,000,000 random numbers
n = 1000000;
x = rand(n, 1);
% Transfer the array of random numbers to the GPU using GPUarray
x_gpu = gpuArray(x);
% Compute the sine value of each random number on the GPU
tic;
y_gpu = sin(x_gpu);
toc;
% Transfer results back to the CPU
y = gather(y_gpu);
```
**Logical Analysis:**
This code uses the `gpuArray` function to transfer the array of random numbers to the GPU, and then computes the sine values using the `sin` function on the GPU. The `gather` function is used to transfer the results back to the CPU.
**Parameter Description:**
* `n`: The number of random numbers
* `x`: Array of random numbers
* `x_gpu`: Array of random numbers on the GPU
* `y_gpu`: Array of sine values on the GPU
* `y`: Array of sine values on the CPU
### 2.2 Distributed Computing in MATLAB
MATLAB also offers distributed computing capabilities that allow tasks to be executed in parallel across multiple computers. This is particularly useful for large-scale Monte Carlo simulations that require substantial computational resources.
#### 2.2.1 Cloud Computing Platforms
MATLAB supports integration with cloud computing platforms such as AWS and Azure, allowing distributed computing to be executed in the cloud. This provides scalable computing resources on demand, which can be easily expanded or reduced according to needs.
```matlab
% Create a MATLAB job
job = createJob('myJob');
% Add tasks to the job
addTask(job, @myFunction, 10);
% Submit the job to the cloud
submit(job);
% Wait for the job to complete
waitFor(job);
% Get the job results
results = getAllOutputArguments(job);
```
**Logical Analysis:**
This code uses the `createJob` function to create a MATLAB job, and then uses the `addTask` function to add tasks to the job. The `submit` function submits the job to the cloud, the `waitFor` function waits for the job to complete, and the `getAllOutputArguments` function retrieves the job results.
**Parameter Description:**
* `job`: MATLAB job object
* `myFunction`: Function handle for the task to be executed
* `results`: Results of the job
#### 2.2.2 Distributed Computing Toolbox
MATLAB also provides a Distributed Computing Toolbox, which allows distributed computing to be executed on local computer clusters or cloud computing platforms. This toolbox provides advanced functions for creating and managing distributed computing jobs.
```matlab
% Create a distributed computing pool
pool = parpool;
% Execute tasks on the distributed computing pool
spmd
% Execute tasks
end
% Delete the distributed computing pool
delete(pool);
```
**Logical Analysis:**
This code uses the `parpool` function to create a distributed computing pool, and then uses the `spmd` block to execute tasks in parallel across all workers in the pool. The `delete` function deletes the
0
0
相关推荐







