Exploring Cutting-edge Fields: Innovative Applications of Monte Carlo Simulation in MATLAB
发布时间: 2024-09-15 10:17:59 阅读量: 22 订阅数: 23 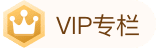
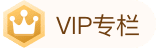
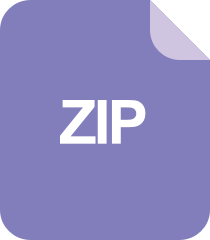
Exploring-Data-Analysis:探索性数据分析期末报告,text clustering with KmeansGMMNMF
# 1. Introduction to Monte Carlo Simulation**
Monte Carlo simulation is a powerful numerical method used to solve complex problems where analytical solutions are difficult or costly to obtain. It is based on random sampling and probability theory, approximating the expected value or distribution of a problem by simulating a large number of random events.
In MATLAB, Monte Carlo simulation is implemented using built-in functions and toolboxes, including `rand()` and `randn()` for generating random numbers, and `integral()` and `sum()` for integration and summation. These functions provide flexibility and scalability, making Monte Carlo simulation a powerful tool for solving a variety of problems.
# 2.1 Foundations of Probability Theory and Statistics
### Foundations of Probability Theory
Probability theory is the foundation of the Monte Carlo method, providing a mathematical framework for describing uncertainty and random events.
**Basic Concepts:**
***Event:** A set of possible occurrences.
***Probability:** The likelihood of an event occurring, ranging between 0 and 1.
***Random Variable:** A variable that can take on different values, determined by a probability distribution.
**Probability Distributions:**
***mon probability distributions include:
* Normal distribution
* Uniform distribution
* Exponential distribution
* Binomial distribution
### Foundations of Statistics
Statistics provide a set of tools for extracting meaningful information from data. The Monte Carlo method uses statistical techniques to estimate probabilities and uncertainties.
**Basic Concepts:**
***Sample:** A set of data extracted from a population.
***Sample Mean:** The average of all values in a sample.
***Sample Variance:** The degree of dispersion of values within a sample.
***Confidence Interval:** A range that contains the population mean with a known probability.
### The Connection Between Monte Carlo Method, Probability Theory, and Statistics
The Monte Carlo method utilizes principles of probability theory and statistics to simulate random events. By generating a large number of random samples, the Monte Carlo method can estimate probabilities, integrals, and sums.
# 3. Practical Applications of Monte Carlo Simulation in MATLAB
### 3.1 Random Number Generation and Distribution Fitting
In MATLAB, ***mon random number generators include:
- `rand()`: Generates uniformly distributed random numbers.
- `randn()`: Generates normally distributed random numbers.
- `randsample()`: Randomly samples from a given set of data.
**Code Block:**
```matlab
% Generates 100 uniformly distributed random numbers
rand_nums = rand(100, 1);
% Generates 100 normally distributed random numbers
norm_nums = randn(100, 1);
% Randomly samples 10 numbers from 1 to 100
sample_nums = randsample(1:100, 10);
```
**Logical Analysis:**
* The `rand()` function generates a uniformly distributed random number between 0 and 1.
* The `randn()` function generates a normally distributed random number with a mean of 0 and a standard deviation of 1.
* The `randsample()` function randomly selects a specified number of samples from a given set, with or without replacement.
**Parameter Explanation:**
* Both `rand()` and `randn()` functions take no parameters.
* The `randsample()` function takes three parameters: the set, the number of samples to draw, and whether to sample with replacement.
### 3.2 Monte Carlo Estimation of Integrals and Sums
The Monte Carlo method can be used to estimate integrals and sums. For integration, the Monte Carlo method divides the integration region into many subregions, randomly samples points within these subregions, and calculates the function values at these points. The estimated value of the integral is the average of these function values multiplied by the area of the subregion.
**Code Block:**
```matlab
% Defines the integration function
f = @(x) x.^2;
% Integration interval
a = 0;
b = 1;
% Number of subregions
n = 10000;
% Randomly sample points
x = a + (b - a) * rand(n, 1);
% Calculate function values
y = f(x);
% Estimate the integral
integral_estimate = mean(y) * (b - a) / n;
```
**Logical Analysis:**
* The code defines an integration function `f(x) = x^2`, with the integration interval [0, 1].
* It randomly sa
0
0
相关推荐
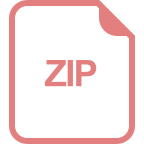
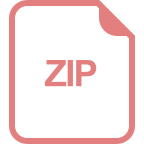
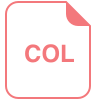
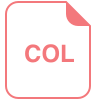
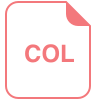
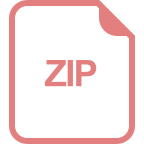
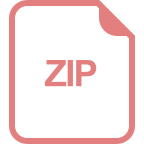