Monte Carlo Simulation for Risk Analysis in MATLAB: A Case Study Analysis
发布时间: 2024-09-15 09:59:37 阅读量: 19 订阅数: 21 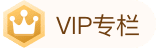
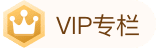
# Monte Carlo Simulation in MATLAB for Risk Analysis: A Practical Case Study
## 1. Foundations of Monte Carlo Simulation
Monte Carlo simulation is a numerical technique based on probability theory, used to solve complex problems. The core idea is to approximate solutions to integrals or other intractable expressions through random sampling.
**Random Number Generation**
A key step in Monte Carlo simulation is generating random numbers. Pseudo-random number generators are algorithms that produce sequences of numbers that appear random but are in fact generated by deterministic algorithms. MATLAB provides several pseudo-random number generators, such as `rand` and `randn`.
**Probability Distributions**
In Monte Carlo simulation, random numbers often need to follow specific probability distributions. MATLAB provides various functions to generate common probability distributions, such as normal, uniform, and Poisson distributions.
## 2. Monte Carlo Simulation in MATLAB
### 2.1 Random Number Generation and Distribution Functions
#### 2.1.1 Pseudo-Random Number Generators
MATLAB offers a variety of pseudo-random number gene***monly used pseudo-random number generators in MATLAB include:
- `rand`: Generates uniformly distributed random numbers.
- `randn`: Generates normally distributed random numbers with a mean of 0 and a standard deviation of 1.
- `randperm`: Generates a random permutation.
```matlab
% Generating 10 uniformly distributed random numbers
rand_numbers = rand(1, 10);
% Generating 10 normally distributed random numbers
normal_numbers = randn(1, 10);
% Generating a random permutation of 10 elements
random_permutation = randperm(10);
```
#### 2.1.2 Implementation of Common Probability Distributions
MATLAB provides functions to generate random numbers from various probability distributions, including:
- `unifrnd`: Generates uniformly distributed random numbers.
- `normrnd`: Generates normally distributed random numbers.
- `exprnd`: Generates exponentially distributed random numbers.
```matlab
% Generating 10 uniformly distributed random numbers in the range [0, 1]
uniform_numbers = unifrnd(0, 1, 1, 10);
% Generating 10 normally distributed random numbers with a mean of 0 and standard deviation of 1
normal_numbers = normrnd(0, 1, 1, 10);
% Generating 10 exponentially distributed random numbers with a parameter of 1
exponential_numbers = exprnd(1, 1, 10);
```
### 2.2 Simulation Process and Results Analysis
#### 2.2.1 Simulation Algorithm Flow
The Monte Carlo simulation algorithm flow is as follows:
1. **Define input parameters:** Determine the parameters to be randomized in the model and their probability distributions.
2. **Generate random samples:** Use pseudo-random number generators and probability distribution functions to generate random samples.
3. **Compute model output:** Calculate the model output for each random sample.
4. **Statistical analysis:** Perform statistical analysis on the simulation output, such as calculating the mean, standard deviation, and distribution.
5. **Repeat steps 2-4:** Repeat the above steps multiple times to obtain reliable results.
#### 2.2.2 Visualization and Statistical Analysis of Results
Simulation results can be visualized and analyzed in various ways, including:
- **Histograms:** Display the distribution of simulation outputs.
- **Box plots:** Show the median, quartiles, and extreme values of simulation outputs.
- **Scatter plots:** Show the relationship between simulation inputs and outputs.
- **Statistics:** Calculate statistical measures of simulation outputs, such as mean, standard deviation, and confidence intervals.
```matlab
% Generating 1000 normally distributed random numbers with a mean of 0 and a standard deviation of 1
normal_numbers = normrnd(0, 1, 1000, 1);
% Plotting a histogram
histogram(normal_numbers);
title('Histogram of Normally Distributed Random Numbers');
% Plotting a box plot
boxplot(normal_numbers);
title('Box
```
0
0
相关推荐
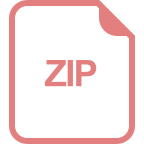
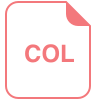
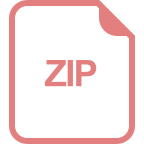
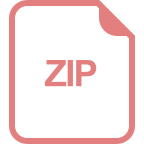
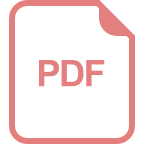
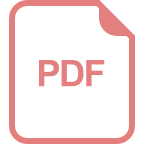
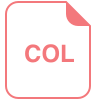
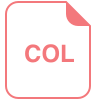