Visual Representation of Outcomes: Visualization of Monte Carlo Simulations in MATLAB
发布时间: 2024-09-15 10:14:34 阅读量: 19 订阅数: 23 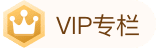
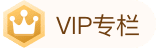
**1. Introduction to Monte Carlo Simulation**
Monte Carlo simulation is a numerical method based on probability theory used to solve complex problems. It estimates the solution to a problem by generating a large number of random samples and performing calculations on these samples. The advantage of Monte Carlo simulation is that it can handle high-dimensional, nonlinear problems and provide probabilistic distribution information about the problem.
In MATLAB, Monte Carlo simulation is commonly used to solve the following types of problems:
* Probability distribution sampling
* Numerical integration
* Risk assessment
**2. Monte Carlo Simulation Theory in MATLAB**
### 2.1 Principles and Methods of Monte Carlo Simulation
Monte Carlo simulation is a numerical method based on probability theory that approximates solutions to complex problems by generating a large number of random samples and calculating their results. The basic principle is as follows:
- **Random Number Generation:** Generate a sequence of random numbers that follow a specific probability distribution.
- **Probability Distribution Sampling:** Draw samples from the sequence of random numbers based on the given probability distribution in the problem.
- **Compute Results:** Calculate each sample to obtain a result value.
- **Statistical Analysis:** Perform statistical analysis on all result values to obtain an approximate solution to the problem.
### 2.1.1 Random Number Generation
MATLAB provides various random number generation functions, such as `rand`, `randn`, `unifrnd`, etc. These functions can generate random numbers that follow different probability distributions. For example:
```matlab
% Generate 10 random numbers from a standard normal distribution
r = randn(10, 1);
```
### 2.1.2 Probability Distribution Sampling
Probability distribution sampling refers to drawing samples from a given probability distribution. MATLAB provides the `random` function for probability distribution sampling. The syntax for this function is:
```matlab
random(Distribution, Parameter1, Parameter2, ..., ParameterN)
```
Where:
- `Distribution`: The type of probability distribution, such as `'normal'`, `'uniform'`, etc.
- `Parameter1`, `Parameter2`, ..., `ParameterN`: Parameters of the probability distribution.
For example, to draw 10 samples from a normal distribution:
```matlab
% Normal distribution parameters: mean 0, standard deviation 1
mu = 0;
sigma = 1;
samples = random('normal', mu, sigma, 10, 1);
```
### 2.2 Error Analysis in Monte Carlo Simulation
The errors in Monte Carlo simulation are mainly divided into two types:
### 2.2.1 Statistical Error
Statistical errors are due to random sampling. As the number of samples increases, statistical errors will decrease. Statistical errors can be measured by the standard deviation.
### 2.2.2 Systematic Error
Systematic errors are due to flaws in the model or algorithm. Systematic errors do not decrease as the number of samples increases. Systematic errors can be reduced by improving the model or algorithm.
**3. Monte Carlo Simulation Practice in MATLAB**
### 3.1 Probability Distribution Sampling Functions
MATLAB provides various functions for generating random numbers from various probability distributions. These functions allow users to specify the parameters of the distribution and generate random samples that conform to that distribution.
#### 3.1.1 Normal Distribution Sampling
The normal distribution sampling function `normrnd` is used to generate random numbers that follow a normal distribution. This function takes two parameters: mean and standard deviation.
```matlab
% Normal distribution sampling
mu = 0; % Mean
sigma = 1; % Standard deviation
n = 1000; % Sample size
x = normrnd(mu, sigma, n, 1); % Generate random samples
```
#### 3.1.2 Uniform Distribution Sampling
The uniform distribution sampling function `unifrnd` is used to generate random numbers that follow a uniform distribution. This function takes two parameters: minimum and maximum values.
```matlab
% Uniform distribution sampling
a = 0; % Minimum value
b = 1; % Maximum value
n = 1000; % Sample size
x = unifrnd(a, b, n, 1); % Generate random samples
```
### 3.2 Integral
0
0
相关推荐
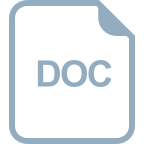
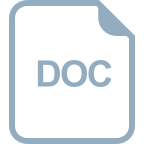
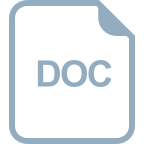
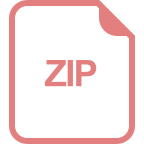
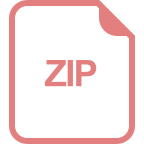
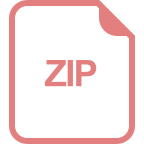
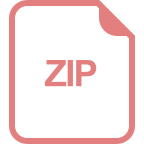
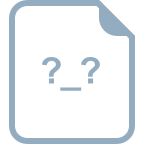
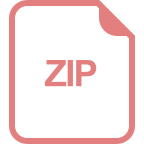