Debugging Monte Carlo Simulations: Avoiding Common Errors in MATLAB
发布时间: 2024-09-15 10:12:05 阅读量: 31 订阅数: 28 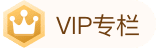
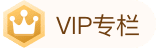
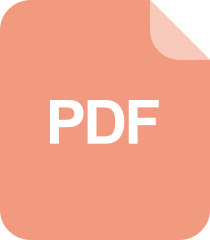
Linux Debugging(五): coredump 分析入門1
# Introduction to Monte Carlo Simulation
Monte Carlo simulation is a numerical technique based on random sampling to solve complex problems where deterministic methods may not be effective. It approximates results by generating a vast number of random samples and performing calculations based on these samples.
Monte Carlo simulation is particularly suitable for the following scenarios:
- Problems involving uncertainty or randomness.
- No analytical solution exists or the computational cost is prohibitive.
- Probability analysis is required for the output results.
# Theoretical Foundations of Monte Carlo Simulation in MATLAB
### 2.1 Probability Distributions and Random Number Generation
The core of Monte Carlo simulation is the use of random numbers to approximate probabili***mon distributions include normal, uniform, exponential, and Poisson distributions.
In MATLAB, the `rand` function can be used to generate uniformly distributed random numbers, and the `randn` function for normally distributed numbers. For other distributions, a probability distribution object can be created using the `makedist` function, followed by generating random numbers with the `random` function.
```matlab
% Generate uniformly distributed random numbers
rand_num = rand(1, 10);
% Generate normally distributed random numbers
randn_num = randn(1, 10);
% Create a Poisson distribution object
poisson_dist = makedist('Poisson', 5);
% Generate Poisson distributed random numbers
poisson_num = random(poisson_dist, 1, 10);
```
### 2.2 Monte Carlo Integration and Sampling
Monte Carlo integration is a method that uses random numbers to approximate the integral of a function. The integral represents the area under a function over a given interval; Monte Carlo integration estimates this area by randomly sampling points within the interval.
In MATLAB, the `integral` function can be used to calculate the integral. For Monte Carlo integration, the `montecarlo` function can be utilized for approximation.
```matlab
% Calculate the integral of a normal distribution
integral(@(x) normpdf(x, 0, 1), -inf, inf)
% Approximate the integral of a normal distribution using Monte Carlo
montecarlo(@(x) normpdf(x, 0, 1), 10000)
```
Monte Carlo sampling is a method that uses random numbers to generate samples from a probability distribution. In MATLAB, samples can be generated using the `random` function or the `datasample` function.
```matlab
% Generate samples from a normal distribution
samples = random('Normal', 0, 1, 1000, 1);
% Generate samples from a uniform distribution
samples = datasample(1:10, 10, 'Replace', false);
```
### 2.3 Error Analysis and Convergence
The error in Monte Carlo simulation consists of two parts: sampling error and statistical error. Sampling error arises from random sampling, while statistical error results from a limited number of samples.
In MATLAB, the `std` function can be used to calculate the standard deviation to estimate the error. Convergence refers to the increasing accuracy of Monte Carlo simulation results as the sample size grows.
```matlab
% Calculate the standard deviation of Monte Carlo integration
std_dev = std(montecarlo(@(x) normpdf(x, 0, 1), 10000));
% Plot a convergence graph
sample_sizes = 100:100:10000;
errors = zeros(size(sample_sizes));
for i = 1:length(sample_sizes)
errors(i) = std(montecarlo(@(x) normpdf(x, 0, 1), sample_sizes(i)));
end
plot(sample_sizes, errors);
xlabel('Sample Size');
ylabel('Standard Deviation');
```
# Basic Syntax and Functions
In MATLAB, Monte Carlo simulation can be implemented using built-in functions and custom code. Here are some commonly used basic syntax and functions:
**Random Number Generation:**
* `rand`: Generate uniformly distributed random numbers.
* `randn`: Generate normally distributed random numbers.
* `randsample`: Randomly draw elements from a given set.
**Distribution Sampling:**
* `normrnd`: Generate random numbers from a normal distribution.
* `unifrnd`: Generate random numbers from a uniform distribution.
* `exprnd`: Generate random numbers from an exponential distribution.
**Integral Calculation:**
* `integral`: Calculate the integral.
* `trapz`: Calculate the integral using the trape
0
0
相关推荐







