Optimization of Multi-threaded Drawing in QT: Avoiding Color Rendering Blockage
发布时间: 2024-09-15 11:06:59 阅读量: 10 订阅数: 16 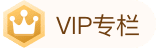
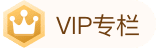
### 1. Understanding the Basics of Multithreaded Drawing in Qt
#### 1.1 Overview of Multithreaded Drawing in Qt
Multithreaded drawing in Qt refers to the process of performing drawing operations in separate threads to improve drawing performance and responsiveness. By leveraging the advantages of multicore processors, multiple drawing tasks can be handled simultaneously, thus preventing blocking in the main thread during drawing operations.
#### 1.2 Why Does Color Rendering Cause Blocking?
Color rendering can cause blocking because performing color calculations and drawing operations in the main thread consumes a significant amount of computational resources and time. When the drawing tasks are too heavy, the main thread cannot execute other operations while waiting for the drawing to complete, resulting in界面卡顿 (界面 freezing) and response delays.
#### 1.3 Advantages and Challenges of Multithreaded Drawing
The advantage of multithreaded drawing lies in its ability to enhance drawing performance and smoothness, making the interface more responsive. However, multithreaded drawing also faces challenges such as thread synchronization, resource competition, and data consistency, requiring careful design and handling.
This concludes the first chapter. If you need to continue with other chapters or have other requirements, please let me know.
# 2. Basic Steps to Implement Multithreaded Drawing
In this chapter, we will introduce the basic steps to implement multithreaded drawing, which will help you better understand how to perform drawing operations using multithreading in Qt.
#### 2.1 Creating a Drawing Thread
In Qt, creating a drawing thread typically involves inheriting from the `QThread` class and overriding its `run()` method to perform drawing operations. Here is a simple example code:
```python
import sys
from PyQt5.QtWidgets import QApplication, QWidget
from PyQt5.QtCore import Qt, QThread
class DrawingThread(QThread):
def run(self):
# Perform drawing operations
pass
# Create an instance of the drawing thread
thread = DrawingThread()
thread.start()
```
#### 2.2 Data Preparation and Transfer
In multithreaded drawing, sometimes data needs to be prepared in the main thread and then passed to the drawing thread for rendering. Data transfer can be accomplished via the signal-slot mechanism.
```python
class DrawingThread(QThread):
data_ready = pyqtSignal(object)
def run(self):
# Perform drawing operations, using self.data_ready signal to receive data
pass
# Create an instance of the drawing thread
thread = DrawingThread()
# Prepare data and pass it to the drawing thread
data = prepare_data()
thread.data_ready.connect(data)
thread.start()
```
#### 2.3 Synchronization and Communication Between Drawing Threads
In multithreaded drawing, different drawing threads may need to synchronize and communicate to ensure the smooth progress of drawing operations. The `QMutex` and `QWaitCondition` classes can be used to achieve synchronization and communication between threads.
```python
from PyQt5.QtCore import QMutex, QWaitCondition
class DrawingThread(QThread):
mutex = QMutex()
wait_condition = QWaitCondition()
def run(self):
self.mutex.lock()
self.wait_condition.wait(self.mutex)
# Drawing operation
self.mutex.unlock()
# Create multiple drawing threads
thread1 = DrawingThread()
thread2 = DrawingThread()
# Start the threads
thread1.start()
thread2.start()
# Send signals to wake up the drawing threads
thread1.wait_condition.wakeAll()
```
With these steps, you can better understand how to implement basic multithreaded drawing operations and ensure data transfer and synchronization communication between threads.
# 3. Solutions to Optimize Color Rendering and Avoid Blocking
During the process of multithreaded drawing, color rendering is often one of the main causes of blocking. To improve drawing efficiency, we can adopt the following optimization solutions to avoid color rendering blocking:
#### 3.1 Asynchronous Loading of Color Resources
Befo
0
0
相关推荐
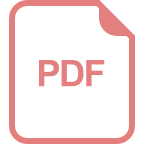
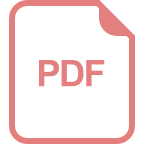
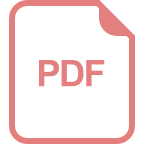





