Advanced Features of QT Graphics: Implementing Multi-View Display with Graphics View Framework
发布时间: 2024-09-15 11:05:16 阅读量: 22 订阅数: 27 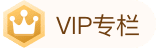
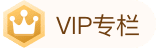
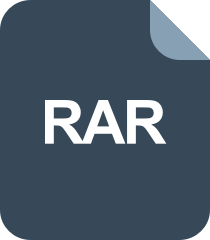
Deep Learning with Python: A Hands-on Introduction
# 1. Introduction to the Graphics View Framework
- **1.1 Overview of the Graphics View Framework**
- **1.2 Advantages and Application Scenarios of the Graphics View Framework**
- **1.3 Introduction to the Components of the Graphics View Framework**
# 2. A Review of QT Drawing Fundamentals
### 2.1 Basic Concepts of QT Drawing
In QT, drawing is achieved through a drawing engine and mainly involves the following concepts:
- **QPainter**: A class used to perform drawing operations, capable of drawing various graphical elements on components such as QWidget or QGraphicsView.
- **QPen**: A pen class defining the edges of drawn graphics, with properties such as color, line style, and width that can be set.
- **QBrush**: A brush class defining the filling of graphic areas, with attributes like color, gradients, and textures that can be set.
- **QColor**: A color class used to define RGB values or predefined colors for colors.
### 2.2 Introduction to QT-Related Drawing Class Libraries
QT offers a rich set of drawing class libraries, including:
- **QPainter**: A class that performs drawing operations, providing functionality to draw basic shapes, text, images, and more.
- **QPaintDevice**: A base class for drawing devices, such as QWidget, QPixmap, etc., providing a drawing context.
- **QPen**: A pen class used for setting properties when drawing lines.
- **QBrush**: A brush class for setting attributes when filling areas.
- **QFont**: A font class used for setting fonts when drawing text.
- **QColor**: A color class used to represent color values.
### 2.3 Analysis of a QT Drawing Example
Let's understand these concepts through a simple QT drawing example:
```python
import sys
from PyQt5.QtWidgets import QApplication, QWidget
from PyQt5.QtGui import QPainter, QPen, QBrush
from PyQt5.QtCore import Qt
class MyWidget(QWidget):
def __init__(self):
super().__init__()
def paintEvent(self, event):
painter = QPainter(self)
painter.setPen(QPen(Qt.blue, 3, Qt.SolidLine)) # Set the pen
painter.setBrush(QBrush(Qt.red, Qt.SolidPattern)) # Set the brush
painter.drawRect(50, 50, 200, 200) # Draw a rectangle
if __name__ == '__main__':
app = QApplication(sys.argv)
widget = MyWidget()
widget.resize(300, 300)
widget.show()
sys.exit(app.exec_())
```
Code Analysis:
- Create a custom window class MyWidget, inheriting from QWidget.
- Override the paintEvent method to perform drawing operations.
- Set pen and brush properties, then draw a rectangle with a blue border and red fill.
- Finally, display the window and run the application.
Through the above code, we can achieve a simple drawing effect. In actual development, more complex drawing functions can be implemented by inheriting from QWidget or QGraphicsView.
This is just a simple example; QT's drawing capabilities are very powerful and can meet various complex scenarios for drawing needs.
# 3. Analysis of Multi-View Display Requirements
- **3.1 Definition and Usage of Multi-View Display**
In many applications, it is necessary to display multiple views simultaneously, allowing users to conveniently view and compare different data or information. Multi-view display can enhance user experience, help users quickly obtain the information they need, and improve work efficiency. For example, in CAD software, users may need to simultaneously view design drawings from different perspectives; in map applications, users may need to display different map layers at the same time.
- **3.2 Design Considerations for Multi-View Display**
- **Interface Layout**: How to reasonably arrange multiple views so that users can easily view and operate.
- **View Interaction**: How views interact with each other, whether features like synchronized scrolling and zooming are needed.
- **Performance Optimization**: Displaying multiple views simultaneously may affect system performance, and methods must be considered to optimize display efficiency.
- **User Experience**: Multi-view display should be in line with user habits, with simple and convenient operations and clear and intuitive interfaces.
- **Scalability**: Consider future functional expansion and changes in requirements, and design should be flexible and scalable.
- **3.3 Comparison of Advantages and Disadvantages of Multi-View Display**
- **Advantages**:
- Improves user viewing efficiency, quickly comparing different content.
- Enhances user interaction experience, increasing user satisfaction.
- Can better display complex information, providing more comprehensive data presentation.
- **Disadvantages**:
- Multi-view display may increase system load, with high performance requirements.
- Design complexity is high, requiring consideration of interaction details and interface layout.
- Some users may not be used to viewing multiple views simultaneously, raising the usage threshold.
# 4. Implementing Multi-View Display with the Graphics View Framework
In this chapter, we will delve into how to utilize the Graphics View framework to implement multi-view display capabilities. By creating a multi-view window with Graphics View, adding and managing different views, and controlling the interactive functions of multi-view display, we can construct more flexible and powerful multi-view display applications.
###
0
0
相关推荐
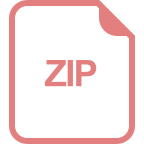
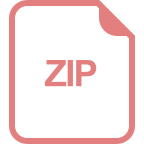





