【Advanced Chapter】Advanced Web Crawler Project Practice: Large-scale Data Collection: Implementing a Distributed Crawler System
发布时间: 2024-09-15 12:53:12 阅读量: 26 订阅数: 36 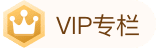
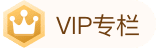
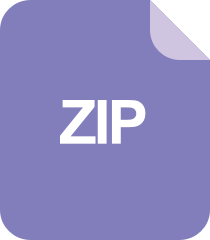
leetcode有效期-python-beginner-webcrawler-infographic:python-初学者-webcrawle
# Advanced Web Crawler Project Implementation: Large-scale Data Collection - Building a Distributed Crawler System
## 1. Overview of Distributed Crawler Systems
A distributed crawler is a type of crawler system that leverages distributed computing technologies to accomplish large-scale web crawling tasks through the collaborative work of multiple nodes. It offers advantages such as high concurrency, efficiency, and reliability, and is widely used in areas such as e-commerce data collection, public opinion monitoring, and search engine optimization.
Distributed crawler systems typically consist of several components, including a crawler scheduler, crawler distributor, crawler executor, data storage system, and monitoring system. The crawler scheduler manages crawling tasks and assigns them to the crawler distributor; the crawler distributor then assigns tasks to the crawler executors; the crawler executors are responsible for executing the crawling tasks to retrieve web page content; the data storage system is responsible for storing the retrieved web page content; and the monitoring system is responsible for monitoring the operation status of the crawler system, promptly detecting and handling faults.
## 2. Distributed Crawler Architecture Design
### 2.1 Advantages and Challenges of Distributed Crawlers
**Advantages:**
***Scalability:** Distributed architectures allow for easy expansion of the crawler system to handle vast amounts of data and concurrent requests.
***High Availability:** Components within the distributed system can be redundant, enhancing system availability and fault tolerance.
***Parallel Processing:** Distributed crawlers can simultaneously crawl data across multiple nodes, significantly improving crawling efficiency.
***Data Consistency:** Distributed storage systems can ensure data consistency, even in the event of node failures or network outages.
**Challenges:**
***System Complexity:** Distributed architectures increase system complexity, requiring consideration of communication, coordination, and fault tolerance between components.
***Data Consistency:** Maintaining data consistency in a distributed environment requires additional mechanisms, such as distributed transactions or eventual consistency.
***Network Latency:** Network latency between distributed components can affect system performance and stability.
***Resource Management:** Distributed systems need to manage a large number of resources, such as computing, storage, and networking, to ensure smooth system operation.
### 2.2 Common Patterns in Distributed Crawler Architectures
**Master-Slave Pattern:**
* A master node coordinates crawling tasks and assigns them to slave nodes for execution.
* Slave nodes return crawling results to the master node for aggregation and storage.
* Advantages: Simple and easy to use, good scalability.
* Disadvantages: A master node failure can cause the system to collapse.
**Cluster Pattern:**
* Multiple nodes execute crawling tasks simultaneously without a master-slave relationship.
* Nodes communicate and coordinate through message queues or other mechanisms.
* Advantages: High availability, good scalability.
* Disadvantages: Complex coordination, difficulty in maintaining data consistency.
**Hybrid Pattern:**
* Combines the advantages of both master-slave and cluster patterns.
* The master node is responsible for task assignment and coordination, while slave nodes form clusters for parallel data crawling.
* Advantages: Balances high availability, scalability, and data consistency.
* Disadvantages: High complexity in implementation.
### 2.3 Selection and Design of Distributed Crawler Architectures
The selection of the architecture needs to consider the following factors:
***Crawling Scale:** The amount of data and concurrent requests to be processed.
***Data Consistency Requirements:** Whether strong or eventual consistency is required.
***System Availability Requirements:** The system's tolerance for faults.
***Resource Constraints:** Available computing, storage, and network resources.
When designing, consider the following aspects:
***Component Division:** Divide the crawler system into different components, such as scheduler, distributor, executor, and storage.
***Communication Mechanism:** Choose an appropriate communication mechanism, such as message queues, RPC, or HTTP.
***Fault Handling:** Design fault handling mechanisms to ensure the system continues to run in the event of component failures.
***Load Balancing:** Implement load balancing strategies to optimize resource utilization and system performance.
**Code Example:**
```python
# Master node code in the master-slave pattern
import time
import requests
# Creating a task queue
task_queue = []
# Crawling task
def crawl_task(url):
# Sending the crawl request
response = requests.get(url)
# Parsing and saving the crawl results
# Master node loop
while True:
# Getting tasks from the task queue
url = task_queue.pop(0)
# Assigning tasks to slave nodes
requests.post("***", json={"url": url})
# Waiting for results from slave nodes
# Saving crawl results
```
```python
# Slave node code in the master-slave pattern
import requests
# Receiving tasks assigned by the master node
url = requests.get("*
```
0
0