Advanced Techniques: Optimizing Crawler Performance and Concurrency Control with Asynchronous Frameworks for Enhanced Efficiency
发布时间: 2024-09-15 12:40:55 阅读量: 26 订阅数: 37 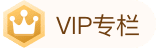
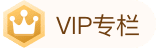
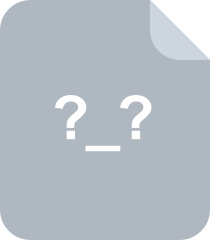
Optimizing Java: Practical Techniques for Improving JVM Application Performance

# [Advanced篇] Optimizing Crawler Performance and Concurrency Control: Improving Crawler Efficiency with Asynchronous Frameworks
## 1. Overview of Crawler Performance Optimization
Crawler performance optimization refers to enhancing the efficiency and speed of a crawler through various techniques and methods, thereby improving the quality and efficiency of the data being scraped. Crawler performance optimization encompasses multiple aspects, including the application of asynchronous frameworks, concurrency control, performance bottleneck analysis, and optimization techniques.
The necessity for optimizing crawler performance lies in:
***Increasing Scraping Efficiency:** An optimized crawler can scrape data more quickly, thus enhancing overall scraping efficiency.
***Enhancing Data Quality:** An optimized crawler can reduce scraping errors and data loss, thereby improving the quality of the scraped data.
***Reducing Resource Consumption:** An optimized crawler can decrease the consumption of server and network resources, thus lowering costs and increasing stability.
## 2. The Application of Asynchronous Frameworks in Crawlers
### 2.1 Principles and Advantages of Asynchronous Frameworks
An asynchronous framework is a software library that allows tasks to be executed without blocking. It achieves this by scheduling tasks to separate threads or processes, allowing the program to continue executing other tasks while waiting for tasks to complete.
The advantages of asynchronous frameworks include:
- **Higher Throughput:** By allowing the program to handle multiple tasks simultaneously, asynchronous frameworks can increase throughput.
- **Lower Latency:** Asynchronous frameworks can reduce latency since the program does not have to wait for a task to complete before continuing execution.
- **Better Scalability:** Asynchronous frameworks can be easily scaled to handle larger loads, as threads or processes can be added or removed as needed.
### 2.2 Introduction and Comparison of Common Asynchronous Frameworks
There are many different asynchronous frameworks available, each with its own set of advantages and disadvantages. Here are some of the most commonly used asynchronous frameworks:
| Framework | Language | Advantages | Disadvantages |
|---|---|---|---|
| asyncio | Python | Easy to use | Limited to Python only |
| Tornado | Python | High performance | Complexity |
| gevent | Python | Lightweight | Stability |
| Node.js | JavaScript | High performance | Single-threaded |
| Go | Go | High concurrency | Steep learning curve |
### 2.3 Practice of Asynchronous Frameworks in Crawlers
Asynchronous frameworks are highly useful in crawlers as they can increase throughput, reduce latency, and enhance scalability. Here are some examples of how asynchronous frameworks are used in crawlers:
- **Concurrent Requests:** Asynchronous frameworks can be used to concurrently send requests, which can improve the throughput of the crawler.
- **Non-blocking Parsing:** Asynchronous frameworks can be used for non-blocking parsing of responses, which can reduce the latency of the crawler.
- **Scalability:** Asynchronous frameworks can be easily scaled as needed to handle larger loads.
#### Code Example
Below is an example of using the asyncio framework in Python to implement concurrent requests:
```python
import asyncio
async def fetch(url):
response = await asyncio.get(url)
return response.text
async def main():
tasks = [fetch(url) for url in urls]
responses = await asyncio.gather(*tasks)
if __name__ == "__main__":
asyncio.run(main())
```
In this example, the `fetch()` function is an asynchronous function that uses `asyncio.get()` to concurrently send requests. The `main()` function uses `asyncio.gather()` to wait for all tasks to complete.
## 3. Crawler Concurrency Control
### 3.1 Necessity and Challenges of Concurrency Control
**Necessity of Concurrency Control**
Concurrency control is crucial in crawler systems because it can:
* Improve crawler efficiency: By executing multiple requests simultaneously, the time required to complete tasks can be reduced.
* Prevent server overload: By limiting the number of requests sent to the server at the same time, server crashes due to overload can be prevented.
* Adhere to website scraping rules: Many websites have scraping rules that limit the number of requests that can be sent simultaneously. If these rules are not followed, the crawler may be blocked.
**Challenges of Concurrency Control**
Implementing effective concurrency control faces the following challenges:
***Resource Limitations:** The degree of concurrency in a crawler is limited by available resources such as memory, CPU, and network bandwidth.
***Server Response Time:** Server response times are unpredictable, which can lead to request backlogs and reduced crawler efficiency.
***Deadlocks:** When two or more requests are waiting for each other, deadlocks c
0
0