【Practical Exercise】Web Scraper Project: Scraping Product Information from E-commerce Websites and Conducting Price Comparisons
发布时间: 2024-09-15 12:58:29 阅读量: 24 订阅数: 38 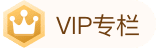
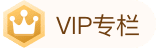
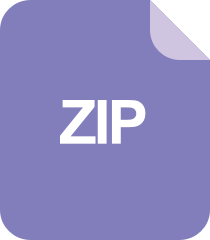
python-basic-web-scraper:python-basic-web-scraper
## Practical Exercise: Web Scraper Project - Harvesting E-commerce Product Information for Price Comparison
# 1. Overview of Web Scraper Project**
A web scraper, also known as a web spider or web crawler, is an automated tool designed to collect and extract data from the internet. Engaging in a web scraper project involves using scraper technology to obtain specific information from websites, process, and analyze it to fulfill particular needs.
This tutorial will guide you through every aspect of a web scraper project, from web parsing and data processing to price comparison and analysis. We will use real-world cases and sample code to walk you through the entire process step by step, helping you master the core concepts and practical skills of web scraper technology.
# 2. Harvesting Product Information from E-commerce Websites
### 2.1 Web Parsing Technology
#### 2.1.1 HTML and CSS Basics
HTML (HyperText Markup Language) and CSS (Cascading Style Sheets) are foundational technologies for web parsing. HTML is used to define the structure and content of web pages, while CSS is used to define the appearance and layout of web pages.
- **HTML Structure**: HTML uses tags to define the structure of web pages, such as `<head>`, `<body>`, `<div>`, `<p>`, etc. Each tag has a specific meaning and function, collectively building the framework of the web page.
- **CSS Styling**: CSS uses rules to define the appearance of web page elements, such as color, font, size, position, etc. With CSS, you can control the visual presentation of web pages, making them more readable and aesthetically pleasing.
#### 2.1.2 Web Parsing Tools and Libraries
Web parsing tools and libraries can help developers parse and extract web content with ease.
- **BeautifulSoup**: A popular Python library for parsing and processing HTML. It offers a variety of methods and attributes for conveniently extracting and manipulating web elements.
- **lxml**: Another Python library for parsing and processing HTML and XML. It is more powerful than BeautifulSoup but also more complex to use.
- **Requests**: A Python library for sending HTTP requests and retrieving web content. It provides a simple and user-friendly API for easily fetching and parsing web pages.
### 2.2 Scraper Frameworks and Tools
Scraper frameworks and tools provide more advanced features to help developers build and manage scraper projects.
#### 2.2.1 Introduction to Scrapy Framework
Scrapy is a powerful Python web scraper framework that offers the following features:
- **Built-in Parsers**: Scrapy has built-in HTML and CSS parsers that make it easy to extract web content.
- **Middleware**: Scrapy provides middleware mechanisms that allow developers to insert custom logic into the crawler's request and response processing.
- **Pipelines**: Scrapy provides pipeline mechanisms that allow developers to clean, process, and store the extracted data.
#### 2.2.2 Using the Requests Library
The Requests library is a Python library for sending HTTP requests and retrieving web content. It offers the following features:
- **Ease of Use**: The Requests library provides a clean and user-friendly API for sending HTTP requests and retrieving responses.
- **Support for Various Request Types**: The Requests library supports various HTTP request types, including GET, POST, PUT, DELETE, etc.
- **Session Management**: The Requests library can manage HTTP sessions, maintaining the state between requests.
**Code Example:**
```python
import requests
# Sending a GET request
response = requests.get("***")
# Retrieving response content
content = response.content
# Parsing HTML content
soup = BeautifulSoup(content, "html.parser")
# Extracting the web page title
title = soup.find("title").text
# Printing the web page title
print(title)
```
**Logical Analysis:**
This code example demonstrates how to use the Requests library to send HTTP requests and parse web content. It first uses the `requests.get()` method to send a GET request to a specified URL. Then, it retrieves the response content and uses BeautifulSoup to parse the HTML content. Finally, it extracts the web page title and prints it.
# 3. Product Information Data Processing
### 3.1 Data Cleaning and Preprocessing
**3.1.1 Data Cleaning Methods and Tools**
Data cleaning is a crucial step in the data processing process, aimed at removing errors, inconsistencies, ***mon cleaning methods include:
- **Removing incomplete or invalid data**: Records with too many missing values or obvious errors are deleted outright.
- **Filling in missing values**: For fields with fewer missing values, methods such as mean, median, or mode can be used to fill them in.
- **Data type conversion**: Convert data into appropriate data types, such as converting strings to numbers or dates.
- **Data formatting**: Standardize the data format, for example, by converting dates into a standard format.
- **Data normalization**: ***
***mon data cleaning tools include:
- Pandas: A powerful data processing library in Python, offering a wealth of cleaning functions.
- NumPy: A Python library for scientific computing, providing array operations and data cleaning features.
- OpenRefine: An interactive data cleaning tool supporting various data formats and custom scripts.
**Code Block: Using Pandas to Clean Data**
```python
import pandas as pd
# Reading data
df = pd.read_csv('product_info.csv')
# Deleting incomplete data
df = df.dropna()
# Filling in missing values
df['price'] = df['price'].fillna(df['price'].mean())
# Data type conversion
df['date'] = pd.to_datetime(df['date'])
# Data formatting
df['date'] = df['date'].dt.strftime('%Y-%m-%d')
```
**Logical Analysis:**
This code block uses Pandas to read a CSV file and then performs the following data cleaning operations:
- Deletes rows with missing values.
- Fills in missing price fields using the mean value.
- Converts the date field to datetime objects.
- Formats the date field to a standard date format.
### 3.1.2 Data Standardization and Normalization
Data standardization and normalization are two important steps in data preprocessing, aimed at converting data into a more suitable form for analysis and modeling.
**Data Standardization**
Data standardization refers to converting data to have ***mon standardization methods include:
- **Min-max scaling**: Scaling data between 0 and 1.
- **Mean normalization**: Subtracting the mean of the data and then dividing by its standard deviation.
- **Decimal scaling**: Multiplying data by the appropriate power of 10 to make the integer part of the data 1.
**Data Normalization**
Data normalization refers to converting data to hav***mon normalization methods include:
- **Normal distribution**: Converting data into a normal distribution.
- **Log transformation**: Taking the logarithm of the data, making its distribution closer to normal.
- **Box-Cox transformation**: A more flexible method that can transform data into various distributions.
**Code Block: Using Scikit-Learn to Standardize Data**
```python
from sklearn.preprocessing import StandardScaler
# Instantiating the scaler
scaler = StandardScaler()
# Standardizing the data
df_scaled = scaler.fit_transform(df)
```
**Logical Analysis:**
This code block uses Sci
0
0
相关推荐
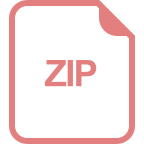
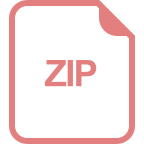
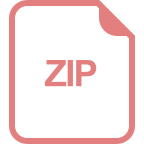
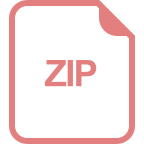
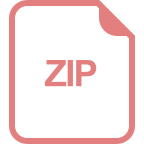
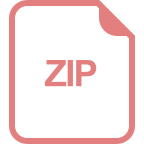
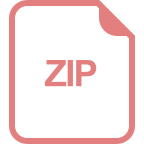
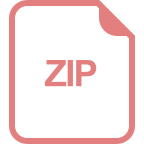