【Advanced Section】Advanced Data Parsing: XPath and Regular Expressions Advanced
发布时间: 2024-09-15 12:23:00 阅读量: 7 订阅数: 27 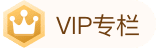
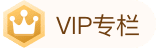
# 2.1 XPath Syntax and Functions
### 2.1.1 Basic XPath Syntax
XPath is a language based on paths, used for locating elements and attributes in XML documents. Its basic syntax is as follows:
```
/root-element/child-element/grandchild-element/...
```
Where:
* `/` signifies starting from the root element.
* `root-element` is the root element of the XML document.
* `child-element` is a child element of the root element.
* `grandchild-element` is a child element of the child element.
* `...` indicates that the path can continue further.
For example, the following XPath expression locates all child elements named `title` under the root element named `book`:
```
/book/title
```
# 2. Advanced Applications of XPath
### 2.1 XPath Syntax and Functions
#### 2.1.1 Basic XPath Syntax
XPath (XML Path Language) is a language used for navigating and querying data in XML documents. Its syntax is based on path expressions, similar to paths in a file system.
An XPath expression consists of:
- **Axis:** Specifies the type of node to traverse, such as `child::`, `parent::`, `following-sibling::`, etc.
- **Node Test:** Specifies the type of node to match, such as `element()`, `text()`, `attribute()`, etc.
- **Predicate:** Used to further filter the matched nodes, like `[condition]`.
For example, the following XPath expression locates all child elements of the `book` element:
```xml
/book/*
```
#### 2.1.2 XPath Functions and Operators
XPath provides a rich set of functions and operators for processing and transforming data.
**Functions:**
- `string()`: Converts a node into a string.
- `number()`: Converts a node into a number.
- `boolean()`: Converts a node into a boolean value.
- `concat()`: Joins strings.
- `substring()`: Extracts a part of a string.
**Operators:**
- `+`: String concatenation.
- `-`: Numeric subtraction.
- `*`: Numeric multiplication.
- `/`: Numeric division.
- `=`: Equality comparison.
- `!=`: Inequality comparison.
For example, the following XPath expression uses the `substring()` function to extract the title of the `book` element:
```xml
/book/title/substring(1, 10)
```
### 2.2 Application of XPath in XML Processing
#### 2.2.1 Structure and Parsing of XML Documents
XML (Extensible Markup Language) is a markup language used for representing and storing data. It has a tree-like structure, consisting of elements, attributes, and text.
XPath can be used to parse XML documents and extract specific information. For example, the following code block uses XPath to parse an XML document and extract the titles of all `book` elements:
```python
import xml.etree.ElementTree as ET
tree = ET.parse('books.xml')
root = tree.getroot()
for book in root.findall('book'):
print(book.find('title').text)
```
#### 2.2.2 Use of XPath in XML Querying and Extraction
XPath can be used to perform various XML querying and extraction operations, including:
- **Finding elements:** Using axes and node tests to locate specific elements.
- **Extracting attributes:** Using the `@` symbol to extract element attributes.
- **Filtering nodes:** Using predicates to filter matched nodes.
- **Navigating the document:** Using axes to traverse nodes in the document.
For example, the following XPath expression locates all `book` elements with an `author` attribute of `"John Doe"`:
```xml
/book[@author="John Doe"]
```
# 3.1 Regular Expression Syntax and Metacharacters
#### 3.1.1 Basic Syntax of Regular Expressions
Regular expressions are a special syntax used for matching text patterns. They use a series of metacharacters and syntactic rules to define the text patterns to be matched. The basic syntax of regular expressions is as follows:
```
pattern = (expression)
expression = term | expression operator term
term = factor | term quantifier
factor = character | char
```
0
0
相关推荐
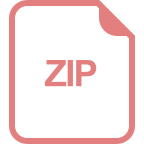
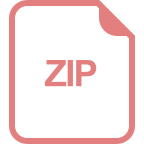
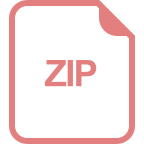





