【Advanced篇】Methods for Captcha Recognition and Processing: Using Third-party Libraries for Graphical Captcha Recognition
发布时间: 2024-09-15 12:33:32 阅读量: 25 订阅数: 32 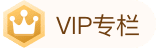
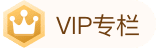
# 1. Overview of CAPTCHA Recognition
CAPTCHA recognition technology plays a crucial role in network security and automation fields. It prevents malicious software and automated programs from accessing protected systems by recognizing distorted characters or numbers in images. CAPTCHA recognition involves various disciplines, including image processing, pattern recognition, and machine learning. This article will delve into CAPTCHA recognition technology, from third-party library practices to algorithmic principles, to CAPTCHA processing and applications, and look forward to future development trends.
# 2. Third-Party Library Practices for CAPTCHA Recognition
### 2.1 Recognizing Graphic CAPTCHAs with Python Third-Party Libraries
#### 2.1.1 OpenCV-Python
OpenCV-Python is a computer vision library widely used for image processing and analysis. It provides a wealth of functions that can be used for CAPTCHA recognition.
```python
import cv2
# Load the CAPTCHA image
image = cv2.imread('captcha.png')
# Convert to grayscale image
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Binarization processing
thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY_INV)[1]
# Find contours
cnts = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
cnts = cnts[0] if len(cnts) == 2 else cnts[1]
# Recognize characters
for c in cnts:
x, y, w, h = cv2.boundingRect(c)
roi = thresh[y:y+h, x:x+w]
cv2.imshow('ROI', roi)
cv2.waitKey(0)
```
**Code Logic Analysis:**
* Load the CAPTCHA image and convert it to a grayscale image.
* Use binarization processing to convert the image to a black and white image.
* Find the contours in the image, where contours represent characters in the CAPTCHA.
* Iterate through each contour, extract the bounding box of the character, and crop the region of interest (ROI).
* Display the ROI image for manual character recognition.
#### 2.1.2 Tesseract-OCR
Tesseract-OCR is an open-source optical character recognition (OCR) engine that can recognize text in images.
```python
import pytesseract
# Load the CAPTCHA image
image = cv2.imread('captcha.png')
# Use Tesseract to recognize text
text = pytesseract.image_to_string(image)
# Print recognition results
print(text)
```
**Code Logic Analysis:**
* Load the CAPTCHA image.
* Use the Tesseract engine to recognize the text in the image.
* Print the recognition results.
### 2.2 Recognizing Graphic CAPTCHAs with Java Third-Party Libraries
#### 2.2.1 ImageJ
ImageJ is an open-source image processing software that provides a wide range of image processing functions, including CAPTCHA recognition.
```java
import ij.ImageJ;
import ij.process.ImageProcessor;
public class ImageJCaptcha {
public static void main(String[] args) {
// Load the CAPTCHA image
ImageJ ij = new ImageJ();
ImageProcessor ip = ij.openImage("captcha.jpg");
// Convert to grayscale image
ip.convertToGray8();
// Binarization processing
ip.threshold(127);
// Find contours
ip.dilate();
ip.erode();
ip.findContours();
// Recognize characters
for (int i = 0; i < ip.getContourCount(); i++) {
ip.setRoi(ip.getContourPolygon(i));
String text = ip.getStringRoiText();
System.out.println(text);
}
}
}
```
**Code Logic Analysis:**
* Load the CAPTCHA image and convert it to a grayscale image.
* Use binarization processing to convert the image to a black and white image.
* Dilate and erode the image to enhance contours.
* Find the contours in the image, where contours represent characters in the CAPTCHA.
* Iterate through each contour, extract the bounding box of the character, and recognize the characters.
#### 2.2.2 AipOcr
AipOcr is an OCR service provided by Baidu that can recognize text in images.
```***
***pOcr;
public class AipOcrCaptcha {
public static void main(String[] args) {
// Set Baidu OCR's App ID, API Key, and Secret Key
String appId = "your_app_id";
String apiKey = "your_api_key";
String secretKey = "your_secret_key";
// Initi
```
0
0
相关推荐
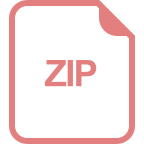
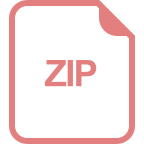
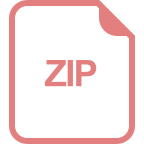
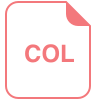
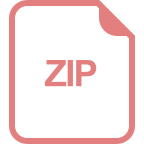
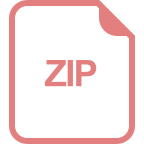
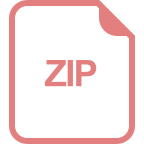
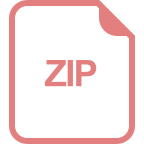
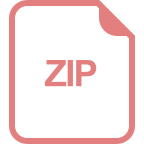