【Advanced】Using Selenium Library to Handle Dynamic Web Pages
发布时间: 2024-09-15 12:12:35 阅读量: 21 订阅数: 33 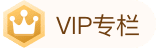
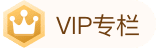
# **[Advanced Tutorial] Handling Dynamic Webpages with Selenium Library**
## 1. Introduction to Selenium Library**
Selenium is an open-source framework designed for automated testing of web applications. It enables testers to interact with browsers using programming languages such as Python, Java, and C#, executing various actions like clicking buttons, entering text, and verifying the presence of elements. The Selenium library offers a comprehensive set of tools and functionalities that empower testers to effectively automate complex web application tests.
## 2. Installation and Configuration of Selenium Library
### 2.1 Installation and Configuration of Selenium WebDriver
**1. Installing Python Selenium Library**
```python
pip install selenium
```
**2. Downloading Browser Drivers**
Depending on the browser used, download the corresponding driver from the Selenium official website. For example, for Chrome browser:
```
***
```
**3. Configuring the Driver Path**
Place the downloaded driver file into the system path, or specify the driver path in the code:
```python
from selenium import webdriver
driver = webdriver.Chrome(executable_path="/path/to/chromedriver")
```
### 2.2 Installation and Configuration of Browser Drivers
**1. Chrome Browser Driver**
* Download the latest version of ChromeDriver.
* Unzip the downloaded zip file and place the chromedriver executable file in the system path.
* Specify the chromedriver path in the code:
```python
from selenium import webdriver
driver = webdriver.Chrome(executable_path="/path/to/chromedriver")
```
**2. Firefox Browser Driver**
* Download the latest version of GeckoDriver.
* Unzip the downloaded zip file and place the geckodriver executable file in the system path.
* Specify the geckodriver path in the code:
```python
from selenium import webdriver
driver = webdriver.Firefox(executable_path="/path/to/geckodriver")
```
**3. Edge Browser Driver**
* Download the latest version of MicrosoftWebDriver.
* Unzip the downloaded zip file and place the msedgedriver executable file in the system path.
* Specify the msedgedriver path in the code:
```python
from selenium import webdriver
driver = webdriver.Edge(executable_path="/path/to/msedgedriver")
```
**4. Safari Browser Driver**
* The Safari browser driver is only compatible with MacOS systems.
* Install Safari Technology Preview.
* Specify the Safari driver in the code:
```python
from selenium import webdriver
driver = webdriver.Safari()
```
## 3.1 Browser Operations
**3.1.1 Creation and Management of Browser Instances**
Selenium WebDriver offers several methods to create and manage browser instances. The most commonly used methods are the browser driver classes such as `webdriver.Chrome()`, `webdriver.Firefox()`, and `webdriver.Edge()`. These classes provide specific methods for starting and controlling particular browsers.
```python
from selenium import webdriver
# Create a Chrome browser instance
driver = webdriver.Chrome()
# Open a web page
driver.get("***")
# Get the current page title
title = driver.title
# Close the browser instance
driver.close()
```
**Logical Analysis:**
* The `webdriver.Chrome()` method creates an instance of the Chrome browser.
* The `driver.get()` method opens a specified webpage.
* The `driver.title` attribute retrieves the title of the current page.
* The `driver.close()` method closes the browser instance.
**3.1.2 Locating and Operating Webpage Elements**
Selenium WebDriver offers multiple methods to locate and operate on webpage elements. The most frequently used methods are `find_element()` and `find_elements()`. These methods allow developers to find elements using various locating strategies such as ID, name, CSS selectors, and XPath.
```python
# Locating an element by ID
element = driver.find_element_by_id("my-element")
# Locating an element by name
element = driver.find_element_by_name("my-element")
# Locating an element by CSS selector
element = driver.find_element_by_css_selector("#my-element")
# Locating an element by XPath
element = driver.find_element_by_xpath("//input[@id='my-element']")
# Getting the text of an element
text = element.text
# Entering text into an element
element.send_keys("Hello, world!")
# Clicking an element
element.click()
```
**Logical Analysis:**
* The `find_element_by_id()` method locates an element by ID.
* The `find_element_by_name()` method locates an element by name.
* The `find_element_by_css_selector()` method locates an element by CSS selector.
* The `find_element_by_xpath()` method locates an element by XPath.
* The `element.text` attribute retrieves the text of an element.
* The `element.send_keys()` method enters text into an element.
* The `element.cl
0
0
相关推荐
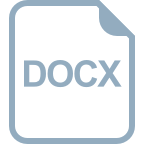
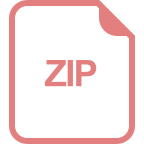
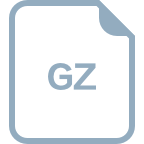
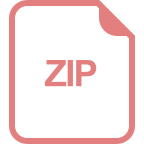
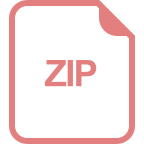
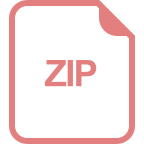
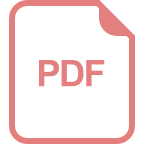