【Basics】Crawler Practice: Scraping Dynamic Webpage Data (AJAX)
发布时间: 2024-09-15 12:07:05 阅读量: 21 订阅数: 37 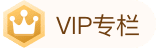
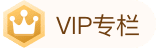
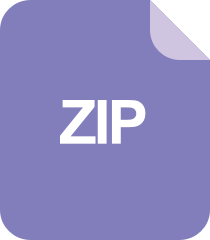
JS-ruby-basics-practice:Ruby vs Javascript基础实践
# 2.1 Fundamentals of AJAX Technology
AJAX (Asynchronous JavaScript and XML) is a web development technology used to create dynamic web pages. It allows web pages to communicate with the server without reloading the entire page, resulting in a smoother and more interactive user experience.
The basic principle of AJAX technology is the use of the XMLHttpRequest object to send and receive data between the client and the server. The XMLHttpRequest object is an object built into web browsers that allows JavaScript code to communicate with the server asynchronously. When a user triggers an event (e.g., clicking a button) on an AJAX-enabled web page, the JavaScript code uses the XMLHttpRequest object to send a request to the server. The server processes the request and returns a response that contains data for updating part of the webpage. Then, the JavaScript code updates the webpage without reloading the entire page.
AJAX technology has several advantages, including:
***Enhanced responsiveness:** AJAX-enabled web pages can respond faster to user interactions as they do not need to reload the entire page.
***Improved user experience:** AJAX web pages can provide a more seamless and interactive user experience by updating content in real-time.
***Reduced server load:** AJAX requests only send and receive the necessary data, which reduces server load.
# 2. AJAX Technology Principles and Crawling Strategies
### 2.1 Fundamentals of AJAX Technology
AJAX (Asynchronous JavaScript and XML) is an asynchronous communication technology that enables web pages to communicate with the server without reloading the entire page. Using AJAX, it's possible to dynamically update webpage content, perform form validation, and enable real-time chat, among other functions.
The core of AJAX technology is the XMLHttpRequest object, which allows web pages to asynchronously communicate with the server through HTTP requests. The XMLHttpRequest object can send and receive data without interrupting the rendering of the web page.
### 2.2 Challenges and Solutions in AJAX Crawling
For traditional web crawlers, the crawling of AJAX web pages presents several challenges:
- **Asynchronous loading:** The content of AJAX web pages is loaded asynchronously, meaning that crawlers cannot directly obtain all content.
- **Dynamically generated content:** The content of AJAX web pages is dynamically generated, meaning that crawlers cannot use traditional HTML parsers to extract content.
- **Cross-domain restrictions:** AJAX requests are usually subject to the same-origin policy, meaning that crawlers cannot cross domains to crawl AJAX web pages.
To overcome these challenges, the following strategies are employed for AJAX crawling:
- **Simulating browser behavior:** Tools like Selenium, PhantomJS, or Puppeteer can be used to simulate browser behavior, triggering AJAX requests and obtaining dynamically loaded content.
- **Handling asynchronous requests:** Asynchronous programming techniques such as JavaScript's Promise or async/await are used to manage AJAX requests and wait for responses.
- **Bypassing cross-domain restrictions:** Techniques such as CORS (Cross-Origin Resource Sharing) or JSONP (JSON with Padding) are used to bypass the limitations of the same-origin policy.
### 2.3 A Practical Example of AJAX Crawling
Here is a practical example of using Selenium to crawl an AJAX web page:
```python
from selenium import webdriver
# Create a Selenium WebDriver instance
driver = webdriver.Chrome()
# Access the AJAX web page
driver.get("***")
# Wait for AJAX requests to complete
driver.implicitly_wait(10)
# Get the dynamically loaded content
content = driver.find_element_by_id("dynamic-content").text
# Print the dynamically loaded content
print(content)
# Close the Selenium WebDriver
driver.quit()
```
In this example, the Selenium WebDriver simulates browser behavior, triggers an AJAX request, and obtains the dynamically loaded content.
# 3. Chapter 3: A Practical Guide to Crawling AJAX Web Pages with Selenium
### 3.1 Introduction and Installation of Selenium
**Selenium** is an open-source automation testing framework that can simulate browser actions to crawl AJAX web pages. Selenium supports multiple programming languages, including Python, Java, C#, and others.
**Installing Selenium**
Taking Python as an example, the steps to install Selenium are as follows:
```
pip install selenium
`
```
0
0
相关推荐
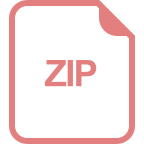
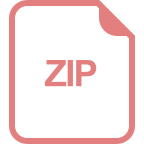
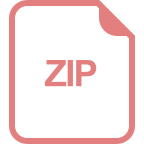
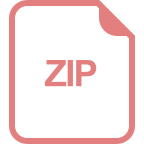
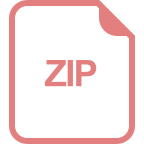
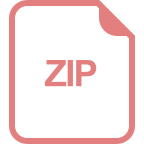
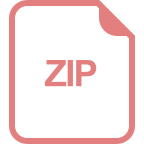
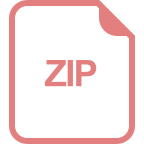