【Advanced Section】Advanced Selenium Techniques: Page Interaction and Element Location
发布时间: 2024-09-15 12:25:23 阅读量: 28 订阅数: 48 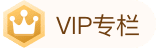
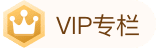
# 1. Advanced Page Interactions with Selenium
Selenium is a powerful automated testing framework that enables developers to interact with web pages just as a real user would. With Selenium, we can automate a variety of tasks, such as:
* Navigating web pages
* Entering data
* Clicking buttons
* Verifying the presence of elements
Selenium provides a multitude of methods for interacting with web page elements, including:
* `find_element()`: Finds and returns a single element
* `find_elements()`: Finds and returns a list of all matching elements
* `click()`: Clicks on an element
* `send_keys()`: Enters text into an element
* `get_attribute()`: Gets the value of an element's attribute
* `set_attribute()`: Sets the value of an element's attribute
* `remove_attribute()`: Removes an element's attribute
# 2. Selenium Element Locating Tips
### 2.1 Basic Methods for Locating Elements
#### 2.1.1 By.id()
The `By.id()` method locates elements by their `id` attribute. The `id` attribute is a unique identifier, thus making this method a quick and accurate way to find elements.
```python
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("***")
element = driver.find_element(By.ID, "element_id")
```
**Parameters:**
* `id`: The value of the `id` attribute of the element to be located.
**Code Logic:**
1. Create a Chrome browser driver using `webdriver.Chrome()`.
2. Load the specified URL using the `get()` method.
3. Locate the element by its `id` attribute using the `find_element()` method and store it in the `element` variable.
#### 2.1.2 By.name()
The `By.name()` method locates elements by their `name` attribute. The `name` attribute is commonly used for form elements, such as input fields and buttons.
```python
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("***")
element = driver.find_element(By.NAME, "element_name")
```
**Parameters:**
* `name`: The value of the `name` attribute of the element to be located.
**Code Logic:**
1. Create a Chrome browser driver using `webdriver.Chrome()`.
2. Load the specified URL using the `get()` method.
3. Locate the element by its `name` attribute using the `find_element()` method and store it in the `element` variable.
#### 2.1.3 By.className()
The `By.className()` method locates elements by their `class` attribute. The `class` attribute can contain multiple values, separated by spaces.
```python
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("***")
element = driver.find_element(By.CLASS_NAME, "element_class")
```
**Parameters:**
* `class`: The value of the `class` attribute of the element to be located.
**Code Logic:**
1. Create a Chrome browser driver using `webdriver.Chrome()`.
2. Load the specified URL using the `get()` method.
3. Locate the element by its `class` attribute using the `find_element()` method and store it in the `element` variable.
# 3.1 Element Retrieval and Verification
**3.1.1 find_element()**
The `find_element()` method is used to find a single element on a page. It takes a locator as a parameter and returns the first element that matches the locator.
```python
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("***")
element = driver.find_element(By.ID, "username")
```
**Parameters:**
* `By.ID`: A locator used to find an element by its ID attribute.
* `"username"`: The ID attribute value of the element.
**Code Logic Analysis:**
1. `driver.get("***")`: Opens the web page of the specified URL.
2. `driver.find_element(By.ID, "username")`: Finds the element with the ID attribute "username" and stores it in the `element` variable.
**3.1.2 find_elements()**
The `find_elements()` method is used to find multiple
0
0
相关推荐








