【Advanced】Advanced Selenium Techniques: Page Interaction and Element Location: Using ActionChains for Handling Page Interactions
发布时间: 2024-09-15 12:48:40 阅读量: 29 订阅数: 38 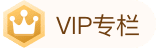
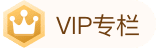
# 2.1 Basic Usage of ActionChains
ActionChains is a class in Selenium WebDriver designed for simulating complex user interactions. It enables developers to create a sequence of actions that can then be executed in one go.
### 2.1.1 Creating an ActionChains Object
To create an ActionChains object, you must first obtain a WebDriver object and then invoke its `action_chains` attribute:
```python
from selenium import webdriver
driver = webdriver.Chrome()
actions = webdriver.ActionChains(driver)
```
### 2.1.2 Adding Actions to ActionChains
Once the ActionChains object is created, various methods can be called to add actions. For example, to click on an element, you can use the `click()` method:
```python
actions.click(element)
```
To move the mouse over to an element, you can use the `move_to_element()` method:
```python
actions.move_to_element(element)
```
# 2. ActionChains Detailed Explanation and Practice
ActionChains is a class in Selenium that enables the simulation of complex user operations. It allows for the combination of a series of actions into a single command. This section will delve into the usage of ActionChains, covering everything from basic operations to advanced applications.
### 2.1 Basic Usage of ActionChains
#### 2.1.1 Creating an ActionChains Object
To start using ActionChains, you need to create an instance using the following syntax:
```***
***mon.action_chains import ActionChains
action_chains = ActionChains(driver)
```
Here, `driver` is the WebDriver instance you are using.
#### 2.1.2 Adding Actions to ActionChains
After creating the ActionChains object, you can add actions using the `perform()` method. The `perform()` method will execute all actions added to the ActionChains.
To add actions, you can use the following methods:
* `click()`: Click on an element
* `move_to_element()`: Move the mouse over to an element
* `drag_and_drop()`: Drag and drop an element onto another element
For example, to click on an element and drag and drop it onto another, you can use the following code:
```python
action_chains.click(element1).drag_and_drop(element1, element2).perform()
```
### 2.2 Common Actions of ActionChains
ActionChains provides a wide range of common actions, including:
#### 2.2.1 Mouse Operations
* `click()`: Click on an element
* `double_click()`: Double-click on an element
* `context_click()`: Right-click on an element
* `move_to_element()`: Move the mouse over to an element
#### 2.2.2 Keyboard Operations
* `key_down()`: Press a key on the keyboard
* `key_up()`: Release a key on the keyboard
* `send_keys()`: Send text to an element
#### 2.2.3 Drag-and-Drop Operations
* `drag_and_drop()`: Drag and drop an element onto another element
* `drag_and_drop_by_offset()`: Drag and drop an element to a specified offset
### 2.3 Advanced Applications of ActionChains
In addition to basic operations, ActionChains also offers the following advanced applications:
#### 2.3.1 Mouse Hover and Right-click
You can use the `move_to_element()` method to hover the mouse over an element and then use the `context_click()` method to right-click on the element.
```python
action_chains.move_to_element(element).context_click().perform()
```
#### 2.3.2 Dragging and Dropping Elements to a Specific Location
You can
0
0
相关推荐
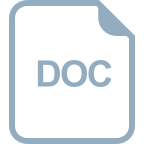
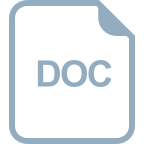
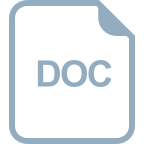
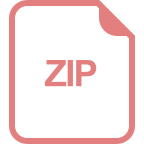
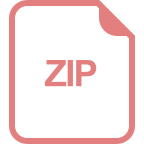
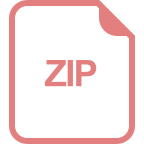
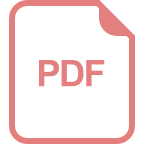
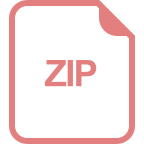
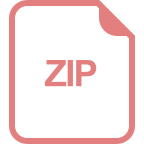