【Basic】Page Parsing Tool Beautiful Soup: Basic Usage and Selectors
发布时间: 2024-09-15 11:52:40 阅读量: 26 订阅数: 37 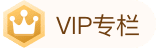
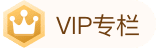
## Introduction to Beautiful Soup: Basic Usage and Selectors
Beautiful Soup is a Python library designed for parsing HTML and XML documents. It provides a suite of simple and powerful methods to help developers extract and manipulate data from web pages. Beautiful Soup is widely used in web scraping, data analysis, and automating web operations.
## Basic Usage of Beautiful Soup
### Creating a Beautiful Soup Object
The Beautiful Soup object is the core of the Beautiful Soup library, representing an HTML or XML document. To create a Beautiful Soup object, the `BeautifulSoup` function is used, which accepts parameters such as:
- `html`: A string of the HTML or XML document to be parsed.
- `features`: Specifies the parser to use. By default, Beautiful Soup uses the `html.parser` parser, but other parsers such as `lxml` or `html5lib` can also be specified.
```python
from bs4 import BeautifulSoup
# Creating a Beautiful Soup object with the default parser
html = '<html><body><h1>Hello, world!</h1></body></html>'
soup = BeautifulSoup(html, 'html.parser')
# Creating a Beautiful Soup object with the lxml parser
soup = BeautifulSoup(html, 'lxml')
```
### Finding and Extracting HTML Elements
After creating a Beautiful Soup object, various methods can be used to find and extract HTML elements.
#### Using Tag Names to Find Elements
The `find_all()` method can find HTML elements by tag name. This method returns a list containing all matching elements.
```python
# Finding all h1 elements
h1_tags = soup.find_all('h1')
# Printing the text content of h1 elements
for h1 in h1_tags:
print(h1.text)
```
#### Using CSS Selectors to Find Elements
The `select()` method can find HTML elements using CSS selectors. CSS selectors are a powerful syntax for precisely selecting HTML elements.
```python
# Finding all elements with class="example"
example_elements = soup.select('.example')
# Printing the text content of example elements
for element in example_elements:
print(element.text)
```
#### Using Regular Expressions to Find Elements
The `find_all()` method can also find HTML elements using regular expressions. Regular expressions are a pattern-matching language used to find strings that match a particular pattern.
```python
# Finding all elements containing the text "example"
example_elements = soup.find_all(text=***pile('example'))
# Printing the text content of example elements
for element in example_elements:
print(element.text)
```
### Extracting HTML Element Content
Once HTML elements are found, their content can be extracted using various methods provided by Beautiful Soup.
#### Getting the Element's Text Content
The `text` attribute contains the text content of an element.
```python
# Getting the text content of the first h1 element
h1_text = h1_tags[0].text
# Printing the text content of the first h1 element
print(h1_text)
```
#### Getting the Element's Attribute Values
The `attrs` attribute contains the attribute values of an element.
```python
# Getting the 'id' attribute value of the first h1 element
h1_id = h1_tags[0].attrs['id']
# Printing the 'id' attribute value of the first h1 element
print(h1_id)
```
## Advanced Usage of Beautiful Soup
### Traversi
0
0
相关推荐
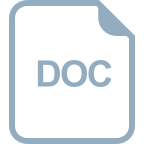
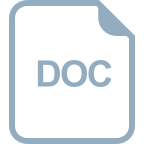
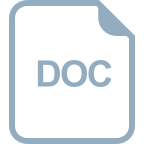
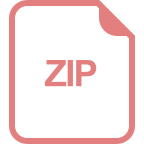
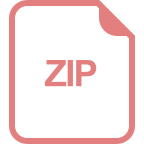
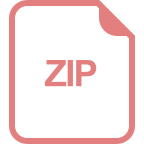
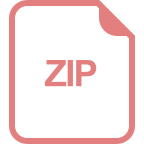
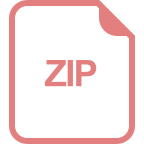
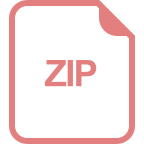