[Advanced Chapter] Efficient Spider Scheduling and Task Queue: Implementing Scheduled Tasks with Celery
发布时间: 2024-09-15 12:35:38 阅读量: 24 订阅数: 32 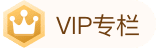
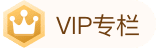
# [Advanced篇] Efficient Crawler Scheduling and Task Queue: Implementing Timed Task Scheduling with Celery
## 1. Overview of Crawler Scheduling
Crawler scheduling is a crucial aspect of managing and coordinating crawler tasks; it handles task distribution, execution, and monitoring. An efficient crawler scheduler can significantly enhance the efficiency and reliability of crawlers.
Crawler schedulers typically implement a task queue approach. A task queue is a data structure used to store tasks waiting to be processed. The crawler scheduler breaks down the crawler tasks into individual tasks and places them into the task queue. The task queue manages the sequence and priority of tasks and assigns them to the crawler processes for execution.
## 2. Celery Task Queue
### 2.1 Basic Principles of Celery
#### 2.1.1 The Concept and Role of Task Queues
A task queue is a distributed system that manages and executes asynchronous tasks. It allows applications to offload time-consuming tasks from the main process, thereby improving the application's responsiveness and throughput. Celery is a popular Python task queue that offers powerful features and scalability, making it highly suitable for crawler scheduling.
#### 2.1.2 Celery's Architecture and Components
Celery's architecture consists of the following components:
***Broker:** Responsible for receiving and storing task messages.
***Worker:** Responsible for executing tasks.
***Backend:** Responsible for persisting task states and results.
Celery uses a message passing mechanism to communicate between the Broker and the Worker. When a task is created, it is sent to the Broker. Workers fetch tasks from the Broker and execute them. Task states and results are stored in the Backend.
### 2.2 Celery Task Scheduling
#### 2.2.1 Task Creation and Execution
In Celery, tasks are defined as Python functions or classes. To create a task, the `@task` decorator can be used. For example:
```python
@task
def crawl_page(url):
# Crawl and parse the page content
pass
```
To execute a task, the `apply_async()` method can be used. This method accepts the task name and parameters as arguments. For example:
```python
crawl_page.apply_async(args=[url])
```
#### 2.2.2 Timed Task Scheduling
Celery supports timed task scheduling. The `schedule_recurring()` method can be used to schedule a task to be executed repeatedly at specified intervals. For example:
```python
crawl_page.schedule_recurring(interval=600) # Crawl a page every 10 minutes
```
#### 2.2.3 Task Monitoring and Management
Celery provides powerful task monitoring and management features. The `celery inspect` command can be used to view task status, retry counts, execution times, and more. The `celery control` command can be used to stop, start, or terminate Workers.
### Code Example
The following code example demonstrates how to use Celery to create a crawler task:
```python
from celery import Celery
# Create a Celery instance
app = Celery('crawler')
# Define a crawler task
@app.task
def crawl_page(url):
# Crawl and parse the page content
pass
# Execute the crawler task
crawl_page.apply_async(args=[url])
```
### Code Logic Analysis
* `Celery('crawler')`: Create a Celery instance, specifying the name as 'crawler'.
* `@app.task`: Decorate the `crawl_page` function as a Celery task.
* `crawl_page.apply_async(args=[url])`: Execute the crawler task, passing `url` as a parameter.
## 3. Applications of Celery in Crawler Scheduling
### 3.1 Splitting and Managing Craw
0
0
相关推荐
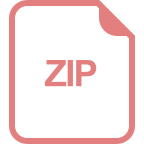
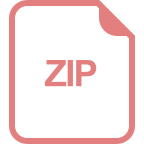
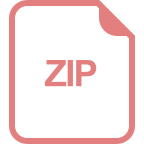
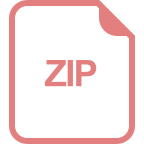
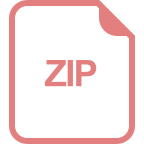
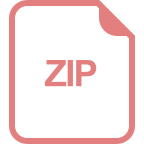
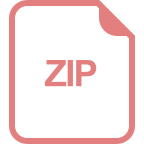
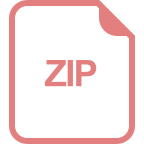
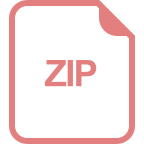