【Basic】Data Extraction Skills: The Application of Regular Expressions in Web Crawling
发布时间: 2024-09-15 11:53:50 阅读量: 21 订阅数: 32 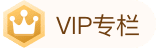
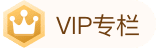
# **1. Fundamentals: Data Extraction Techniques - The Application of Regular Expressions in Web Scraping**
Regular Expressions (Regex) are a powerful tool for text pattern matching, utilizing a set of special characters and syntactic rules to define the text patterns to be matched. The basic syntax of regular expressions includes:
- **Matching Characters:** `.` matches any single character, `[abc]` matches any one of the characters within the square brackets, and `[^abc]` matches any character not in the square brackets.
- **Repetition Matching:** `*` matches the preceding character 0 or more times, `+` matches the preceding character 1 or more times, `?` matches the preceding character 0 or 1 time.
- **Grouping:** `()` groups expressions, allowing for operations to be performed on them, such as referencing or repeating.
- **Anchors:** `^` matches the start of a string, `$` matches the end of a string.
- **Escape Characters:** `\` escapes special characters, removing their special meaning.
# 2. The Application of Regular Expressions in Data Extraction
Regular Expressions (Regex) are a powerful pattern-matching language that allows us to match and extract complex data patterns using concise syntax. In the realm of data extraction, regular expressions play a crucial role as they help us quickly and accurately extract the required information from unstructured text.
### 2.1 Basic Syntax of Regular Expressions
A regular expression consists of a series of metacharacters and literal characters, where metacharacters have special meanings, and literal characters match themselves. Below are some commonly used regular expression metacharacters:
| Metacharacter | Meaning |
|---|---|
| `.` | Matches any single character |
| `*` | Matches the preceding character zero or more times |
| `+` | Matches the preceding character one or more times |
| `?` | Matches the preceding character zero or one time |
| `[]` | Matches any single character within the brackets |
| `^` | Matches the start of the string |
| `$` | Matches the end of the string |
For example, the following regular expression matches any word starting with the letter "a":
```
^a.*
```
### 2.2 Advanced Applications of Regular Expressions
Beyond basic syntax, regular expressions offer many advanced features, such as:
- **Grouping and Referencing:** Use parentheses `()` to group sub-expressions and `\n` to refer to the nth group.
- **Conditional Matching:** Use the `|` separator to match multiple options.
- **Backreferences:** Use `\b` to match word boundaries.
- **Greedy and Non-Greedy Matching:** Use `+?` and `*?` to control the greediness of the match.
### 2.3 The Practical Application of Regular Expressions in Data Extraction
In data extraction, regular expressions can be used for a variety of tasks, such as:
- **Extracting Email Addresses:**
```
[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,4}
```
- **Extracting Phone Numbers:**
```
(\d{3}[-.\s]??\d{3}[-.\s]??\d{4}|\(\d{3}\)\s*\d{3}[-.\s]??\d{4}|\d{3}[-.\s]??\d{4})
```
- **Extracting Dates:**
```
(0[1-9]|[12]\d|3[01])[- /.](0[1-9]|1[012])[- /.](19|20)\d\d
```
**Code Block:**
```python
import re
text = "John Doe, 123 Main Street, Anytown, CA 12345, john.***"
# Extracting Name
name = re.search(r"^(.*?),", text).group(1)
# Extracting Address
address = re.search(r"^(.*?), \d{5}", text).group(1)
# Extracting Email Address
email = re.search(r"[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,4}", text)
print(name)
print(address)
print(email)
```
**Logical Analysis:**
- The `re.search()` function is used to search for the first substring in the string that matches the regular expression.
- The `group(1)` method re
0
0
相关推荐
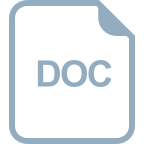
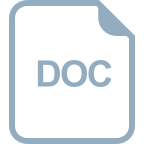
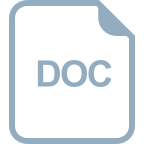
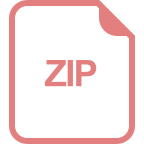
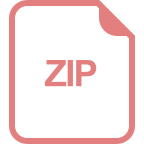
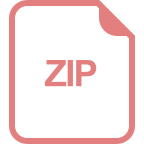
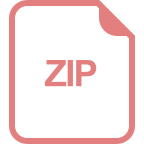
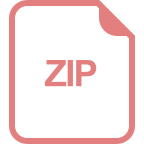
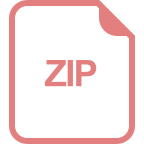