【Advanced】Analysis and Countermeasures of Anti-Crawler Mechanisms
发布时间: 2024-09-15 12:10:24 阅读量: 31 订阅数: 32 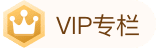
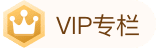
# 2.1 IP Address-Based Restrictions
IP address-based restrictions are the simplest and most direct form of anti-crawling mechanisms. They work by limiting access to the website for specific IP addresses or ranges of IP addresses. This method can effectively block crawlers when they utilize a large number of IP addresses.
**Principle:**
The website server records the visitor's IP address and compares it with a blacklist or whitelist. If the visitor's IP address is on the blacklist, access to the website is denied. If the visitor's IP address is on the whitelist, access to the website is permitted.
**Implementation:**
IP address-based restrictions can be implemented by adding the following rules to the configuration file of the website server:
```
Deny from ***.***.*.*
Allow from ***.***.*.*
```
Here, `Deny` signifies access refusal, `Allow` signifies access permission, and `***.***.*.*` and `***.***.*.*` represent the IP addresses to be restricted or permitted.
# 2. Principles and Implementations of Anti-Crawling Mechanisms
Anti-crawling mechanisms can be implemented in a variety of ways, with the following primary methods:
### 2.1 IP Address-Based Restrictions
#### Principle
IP address-based restrictions are the simplest form of an anti-crawling mechanism, which operates by recording the IP address of a crawler visiting the website and adding it to a blacklist, thereby preventing that IP address from accessing the website again.
#### Implementation
```python
# Import necessary libraries
import ipaddress
# Create a blacklist of IP addresses
blacklist = set()
# Check if the request's IP address is in the blacklist
def check_ip_address(request):
ip_address = request.remote_addr
if ipaddress.ip_address(ip_address) in blacklist:
return True
else:
return False
```
### 2.2 Based on Cookie and Session Restrictions
#### Principle
Cookies and sessions are used by websites to track user states, and anti-crawling mechanisms can leverage this to restrict crawler access. For instance, a website can set a cookie to record the time of the user's last visit. If a crawler accesses the website frequently within a short period, it can be assumed to be a crawler behavior, and appropriate restrictions can be imposed.
#### Implementation
```python
# Import necessary libraries
from datetime import datetime
# Set the cookie's validity period to 1 hour
cookie_max_age = 60 * 60
# Check if the cookie is valid
def check_cookie(request):
cookie = request.cookies.get('last_visit')
if cookie is None:
return False
else:
last_visit = datetime.strptime(cookie, '%Y-%m-%d %H:%M:%S')
if (datetime.now() - last_visit).seconds > cookie_max_age:
return False
else:
return True
```
### 2.3 Based on User-Agent Restrictions
#### Principle
The User-Agent is part of the HTTP request header sent by the browser to the server, which includes information about the browser's type and version. Anti-crawling mechanisms can use User-Agent information to identify crawlers and take appropriate restrictive measures. For example, a website can set a whitelist that only allows access from specific types of browsers.
#### Implementation
```python
# Import necessary libraries
import re
# Create a User-Agent whitelist
whitelist = ['Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/***.*.*.* Safari/537.36']
# Check if the User-Agent is in the whitelist
def check_user_agent(request):
user_agent = request.headers.get('User-Agent')
if user_agent in whitelist:
return True
else:
return False
```
### 2.4 Based on CAPTCHA Restrictions
#### Principle
CAPTCHA is a graphical or textual challenge designed to differentiate humans from machines. Anti-crawling mechanisms can use CAPTCHAs to limit crawler access. For example, a website can use CAPTCHAs on login or registration pages, preventing crawlers that cannot recognize CAPTCHAs from accessing the website.
#### Implementation
```python
# Import necessary libraries
from captcha.models import CaptchaStore
# Generate a CAPTCHA
def generate_captcha():
store = CaptchaStore.objects.create()
return store.image_url, store.hashkey
# Verify a CAPTCHA
def check_captcha(hashkey, answer):
store = CaptchaStore.objects.get(hashkey=hashkey)
return store.answer == answer
```
### 2.5 Based on Behavioral Features Restrictions
#### Principle
Behavioral fe
0
0
相关推荐
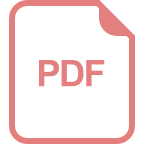
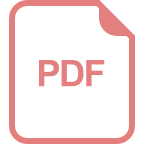
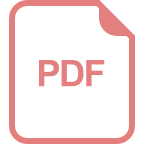



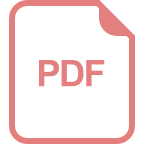
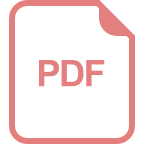
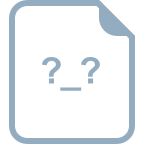