【Advanced】Advanced Applications of Selenium: Page Screenshot, Simulate Mouse and Keyboard Operations
发布时间: 2024-09-15 12:28:21 阅读量: 26 订阅数: 48 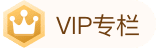
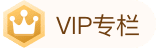
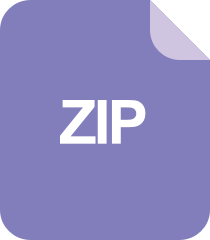
scrapy-selenium:Scrapy中间件使用Selenium处理javascript页面

# Advanced Selenium Applications: Page Screenshots and Simulated Mouse and Keyboard Operations
Selenium offers powerful screenshot capabilities, allowing developers to capture the visual representation of web pages during automated testing processes. The screenshot feature can serve multiple purposes, including:
- Verifying the visibility and correctness of page elements
- Documenting evidence of test failures
- Creating visual regression tests to ensure consistent page layout and content across different browsers and devices
## Page Screenshots
### 2.1 Selenium's Screenshot Functionality
Selenium provides a suite of robust screenshot functions that cater to the needs of various testing scenarios. These functions include:
#### 2.1.1 Types of Screenshots and Usage
| Screenshot Type | Usage |
|---|---|
| `take_screenshot()` | Captures a screenshot of the entire page |
| `get_screenshot_as_base64()` | Converts the screenshot to a base64-encoded string |
| `get_screenshot_as_file()` | Saves the screenshot as a file |
| `get_screenshot_as_png()` | Converts the screenshot to a PNG format byte array |
#### 2.1.2 Saving and Managing Screenshots
Selenium offers various methods to save and manage screenshots:
- **Saving to a *** `get_screenshot_as_file()` method to save the screenshot to a specified file path.
- **Saving to a byte array:** Use the `get_screenshot_as_png()` method to convert the screenshot into a byte array for further processing or storage.
- **Saving to base64 encoding:** Employ the `get_screenshot_as_base64()` method to convert the screenshot into a base64-encoded string, which can be embedded into HTML reports or other documents.
### 2.2 Practical Applications of Page Screenshots
Selenium's screenshot feature has a wide range of applications in automated testing, including:
#### 2.2.1 Screenshots of Page Elements
```python
# Import necessary libraries
from selenium import webdriver
# Create a WebDriver object
driver = webdriver.Chrome()
# Access the target page
driver.get("***")
# Find a page element
element = driver.find_element_by_id("my-element")
# Capture a screenshot of the element
element_screenshot = element.screenshot_as_png()
# Save the screenshot to a file
with open("element_screenshot.png", "wb") as f:
f.write(element_screenshot)
```
#### 2.2.2 Screenshots of the Entire Page
```python
# Import necessary libraries
from selenium import webdriver
# Create a WebDriver object
driver = webdriver.Chrome()
# Access the target page
driver.get("***")
# Capture a screenshot of the entire page
page_screenshot = driver.get_screenshot_as_png()
# Save the screenshot to a file
with open("page_screenshot.png", "wb") as f:
f.write(page_screenshot)
```
#### 2.2.3 Screenshots of Scrolled Pages
```python
# Import necessary libraries
from selenium import webdriver
# Create a WebDriver object
driver = webdriver.Chrome()
# Access the target page
driver.get("***")
# Scroll the page to a specific location
driver.execute_script("window.scrollTo(0, document.body.scrollHeight);")
# Capture a screenshot of the scrolled page
page_screenshot = driver.get_screenshot_as_png()
# Save the screenshot to a file
with open("page_screenshot.png", "wb") as f:
f.write(page_screenshot)
```
## Simulating Mouse and Keyboard Operations
### 3.1 Selenium's Mouse Operations
Selenium provides a rich set of mouse operation methods that simulate user interactions with the mouse on web pages, including moving, clicking, dragging, and releasing.
#### 3.1.1 Mouse Movement and Clicking
- **moveToElement(element)**: Move the mouse to the specified element.
- **click()**: Click the left mouse button on the current element.
- **doubleClick()**: Double-click the left mouse button on the current element.
- **contextClick()**: Right-click the mouse on the current element.
```python
# Move the mouse to the text box
driver.find_element_by_id("username").move_to_element()
# Click on the text box
driver.find_element_by_id("username").click()
# Double-click on the text box
driver.find_element_by_id("userna
```
0
0
相关推荐







