【Practical Exercise】Data Storage and Analysis: Storing Scraped Data into MongoDB and Conducting Statistical Analysis
发布时间: 2024-09-15 12:59:34 阅读量: 20 订阅数: 32 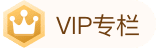
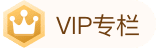
# Data Storage and Analysis: Storing Web Scraped Data in MongoDB and Conducting Statistical Analysis
## 1. Overview of Data Storage and Analysis
Data storage and analysis are indispensable key technologies in modern enterprise operations and decision-making. Data storage is responsible for storing data safely and efficiently, while data analysis extracts valuable insights and information by processing, analyzing, and visualizing the stored data.
The combination of data storage and analysis enables enterprises to fully utilize their data assets, gaining the following advantages:
- **Improved Decision-Making:** By analyzing data, enterprises can gain an in-depth understanding of customer behavior, market trends, and operational efficiency, leading to smarter decisions.
- **Increased Operational Efficiency:** Data analysis can help identify bottlenecks in processes and optimize resource allocation, thereby improving operational efficiency and reducing costs.
- **Identification of New Opportunities:** Data analysis can reveal hidden patterns and trends, aiding enterprises in identifying new business opportunities and growth areas.
- **Enhanced Customer Experience:** By analyzing customer data, enterprises can understand customer needs and preferences, offering personalized experiences and improving customer satisfaction.
## 2. Data Storage Practices
### 2.1 Introduction to MongoDB and Installation
**Introduction to MongoDB**
MongoDB is a document-oriented NoSQL database known for its flexible data model and high performance. It stores data as JSON documents, allowing users to store data in structured or unstructured formats.
**Installation of MongoDB**
**Linux**
```bash
sudo apt-get update
sudo apt-get install mongodb
```
**Windows**
1. Download the MongoDB installer.
2. Run the installer and follow the on-screen instructions.
**macOS**
```bash
brew install mongodb-community
```
### 2.2 Data Modeling and Document Operations
**Data Modeling**
MongoDB uses a document model, where documents are JSON objects containing key-value pairs. Each document belongs to a collection, similar to tables in traditional databases.
**Document Operations**
MongoDB provides a rich API for document operations, including:
***Insertion:** `db.collection.insertOne()`
***Update:** `db.collection.updateOne()`
***Deletion:** `db.collection.deleteOne()`
***Search:** `db.collection.find()`
### 2.3 Data Queries and Aggregation
**Data Queries**
MongoDB uses a query language (similar to SQL) to query data. The query language supports various operators and conditions, allowing users to retrieve data flexibly.
**Aggregation**
The aggregation pipeline enables users to perform complex operations on data, such as grouping, sorting, and computing. The aggregation pipeline is a multi-stage process, where each stage performs a specific operation.
**Code Example**
The following code example demonstrates how to query and aggregate MongoDB data:
```javascript
// Query all documents
db.collection.find();
// Example of an aggregation pipeline
db.collection.aggregate([
{
$group: {
_id: "$category",
count: { $sum: 1 }
}
},
{
$sort: { count: -1 }
}
]);
```
**Logical Analysis**
The first query returns all documents in the collection. The second aggregation pipeline groups documents by the `category` field and calculates the number of documents in each group. Then, the pipeline sorts the results in descending order by the `count` field.
## 3 Data Analysis Practices
### 3.1 Data Preprocessing and Exploration
Data preprocessing is a crucial step in the data analysis process, involving cleaning, transforming, and normalizing raw data to make it suitable for subsequent analysis.
**Data Cleaning**
Data cleaning includes removing missing values, handling outliers, correcting data type errors, and standardizing data formats.
```python
import pandas as pd
# Reading data
df = pd.read_csv('data.csv')
# Removing missing values
df = df.dropna()
# Handling outliers
df['age'] = df['age'].replace(-1, np.nan)
# Correcting data type errors
df['gender'] = df['gender'].astype('category')
# Standardizing data formats
df['date'] = pd.to_datetime(df['date'])
```
**Data Transformation**
Data transformation includes creating new features, merging datasets, and splitting data.
```python
# Creating new features
df['age_group'] = pd.cut(df['age'], bins=[0, 18, 30, 45, 60, np.inf], labels=
```
0
0
相关推荐
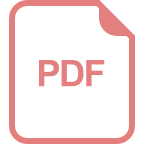
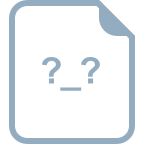
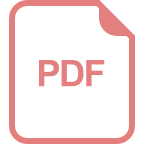
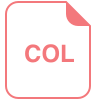
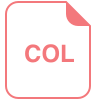
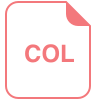
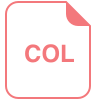
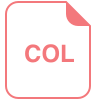
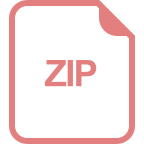