[Advanced Chapter] Advanced Scrapy Practices: Customizing Middleware and Pipelines: Writing Custom Middleware to Handle Requests and Responses
发布时间: 2024-09-15 12:49:53 阅读量: 18 订阅数: 30 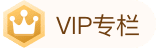
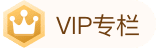
# Advanced Scrapy Practices: Customizing Middlewares and Pipelines
Scrapy is a popular web crawling framework that offers powerful middleware and pipeline mechanisms, allowing users to customize and extend the behavior of crawlers. Middleware and pipelines are key components in the Scrapy architecture, playing crucial roles at various stages of the crawling process.
Middleware primarily deals with requests and responses, allowing users to intercept and modify data during the crawling process. Pipelines are responsible for handling the crawling results, enabling users to further process, filter, and persist the data.
# Customizing Middleware
## 2.1 Types and Functions of Middleware
Middleware in the Scrapy framework is used to process requests and responses. Scrapy provides three types of middleware:
- **Request Middleware**: Processes requests before they are sent to the website.
- **Response Middleware**: Processes responses after the website has returned them.
- **Downloader Middleware**: Handles requests and responses during the processing by Scrapy's downloader.
## 2.2 Writing Custom Middleware
### 2.2.1 Creating Middleware Class
To write custom middleware, create a Python class that inherits from Scrapy's middleware base class:
```python
from scrapy.middlewares import MiddlewareManager
class CustomMiddleware(MiddlewareManager):
# Code for custom middleware
```
### 2.2.2 Implementing Middleware Methods
Custom middleware classes need to implement the following methods:
- `process_request(request, spider)`: Process the request before it is sent to the website.
- `process_response(request, response, spider)`: Process the response after it is returned by the website.
- `process_exception(request, exception, spider)`: Handle exceptions that occur during request processing.
## 2.3 Middleware Configuration and Usage
### 2.3.1 Configuring Middleware in settings.py
To use custom middleware, it needs to be configured in Scrapy's `settings.py` file. Add the path of the custom middleware class to the `MIDDLEWARE_CLASSES` setting:
```python
MIDDLEWARE_CLASSES = {
'scrapy.downloadermiddlewares.httpauth.HttpAuthMiddleware': 800,
'scrapy.downloadermiddlewares.downloadtimeout.DownloadTimeoutMiddleware': 850,
'scrapy.downloadermiddlewares.useragent.UserAgentMiddleware': 400,
'scrapy.downloadermiddlewares.retry.RetryMiddleware': 500,
'scrapy.downloadermiddlewares.redirect.MetaRefreshMiddleware': 800,
'scrapy.downloadermiddlewares.httpcompression.HttpCompressionMiddleware': 810,
'scrapy.downloadermiddlewares.redirect.RedirectMiddleware': 900,
'scrapy.downloadermiddlewares.cookies.CookiesMiddleware': 700,
'scrapy.downloadermiddlewares.httpproxy.HttpProxyMiddleware': 750,
'scrapy.downloadermiddlewares.stats.DownloaderStats': 800,
'scrapy.downloadermiddlewares.httpcache.HttpCacheMiddleware': 900,
'scrapy.spidermiddlewares.offsite.OffsiteMiddleware': 500,
'scrapy.spidermiddlewares.referer.RefererMiddleware': 700,
'scrapy.spidermiddlewares.urllength.UrlLengthMiddleware': 800,
'scrapy.spidermiddlewares.depth.DepthMiddleware': 900,
'scrapy.spidermiddlewares.httperror.HttpErrorMiddleware': 500,
# ... (The list continues with custom middleware configurations)
}
```
# Customizing Pipelines
## 3.1 Types and Functions of Pipelines
Pipelines in Scrapy are components used to process crawled results. They provide a mechanism to handle and modify the results for storage in a database, sending to a message queue, or performing other operations. Scrapy offers two types of pipelines:
- **Item Pipeline**: Processes the entire Item object. They are used for validation, cleaning, transformation, or persistence of Item data.
- **Item Processor**: Processes individual fields of an Item object. They are used for extraction, transformation, or validation of specific field data.
## 3.2 Writing Custom Pipelines
To write a custom pipeline, create a pipeline class and implement the following methods:
- **open_spider(spider)**: Called when the spider starts, used for initializing the pipeline.
- **process_item(item, spider)**: Called after each Item is crawled, used for processing Item data.
- **close_spider(spider)**: Called when the spider is closed, used for cleaning up the pipeline.
## 3.3 Configuring and Using Pipelines
To configure and
0
0
相关推荐
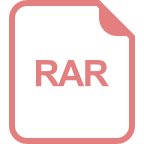
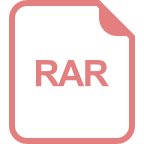
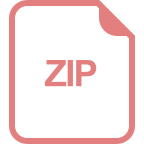
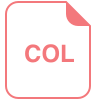
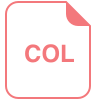
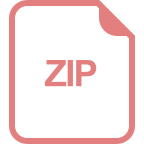
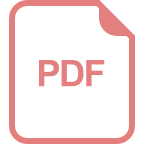
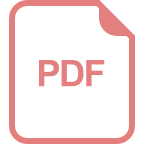
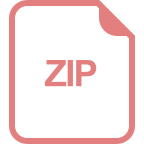