【Fundamentals】Optimizing Crawler Speed: Multithreading and Asynchronous Request Techniques
发布时间: 2024-09-15 12:00:41 阅读量: 23 订阅数: 38 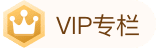
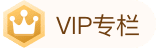
# [Fundamentals] Optimizing Crawler Speed: Multi-threading and Asynchronous Request Tips
## 2.1 Basic Principles of Multi-threading
Multi-threading is a concurrency programming technique that allows a program to execute multiple tasks simultaneously. In multi-threaded programs, each task is executed by an independent thread, and these threads share the same memory space and resources.
The advantage of multi-threading lies in its ability to improve program efficiency and responsiveness. By executing multiple tasks simultaneously, multi-threaded programs can fully utilize multi-core processors, reducing execution time. Additionally, multi-threaded programs can handle user interactions more effectively, such as responding to user input or updating graphical user interfaces.
## 2. Multi-threading Optimization Tips
### 2.1 Basic Principles of Multi-threading
Multi-threading is a concurrency programming technique that allows multiple tasks to be executed simultaneously within a process. By creating and managing multiple threads, a program can process multiple requests or tasks concurrently, thus improving overall performance.
### 2.2 Python Multi-threading Programming
#### 2.2.1 Thread Creation and Management
In Python, the `threading` module can be used to create and manage threads. The `Thread` class provides methods to create and manage threads:
```python
import threading
# Creating a thread
thread = threading.Thread(target=my_function, args=(args,))
# Starting the thread
thread.start()
# Waiting for the thread to complete
thread.join()
```
#### 2.2.2 Thread Synchronization and Communication
When multiple threads access shared resources simultaneously, ***revent this, synchronization mechanisms such as locks and semaphores are needed to coordinate access between threads.
**Lock:** A lock is a synchronization mechanism that allows only one thread to access a shared resource at a time.
```python
import threading
# Creating a lock
lock = threading.Lock()
# Acquiring the lock
lock.acquire()
# Accessing the shared resource
# Releasing the lock
lock.release()
```
**Semaphore:** A semaphore is a synchronization mechanism that limits the number of threads that can access a shared resource simultaneously.
```python
import threading
# Creating a semaphore to limit the number of threads accessing the resource to 5
semaphore = threading.Semaphore(5)
# Acquiring the semaphore
semaphore.acquire()
# Accessing the shared resource
# Releasing the semaphore
semaphore.release()
```
## 2.3 Multi-threading Optimization Practices
#### 2.3.1 Thread Pool Usage
A thread pool is a pre-created and managed collection of threads. Using a thread pool can avoid the overhead of frequent thread creation and destruction, thus improving performance.
```python
import concurrent.futures
# Creating a thread pool
with concurrent.futures.ThreadPoolExecutor(max_workers=5) as executor:
# Submitting tasks to the thread pool
executor.submit(my_function, args=(args,))
```
#### 2.3.2 Implementing Concurrent Requests
In web crawlers, concurrent requests refer to the simultaneous sending of multiple HTTP requests. Multi-threading can implement concurrent requests, thereby increasing the efficiency of crawling.
```python
import threading
# Creating a list
```
0
0
相关推荐
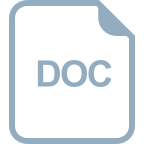
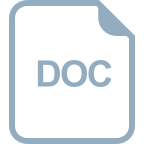
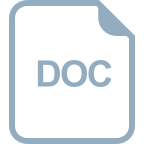
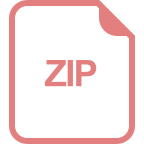
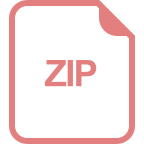
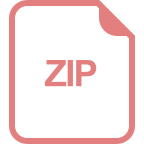
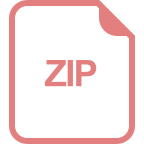
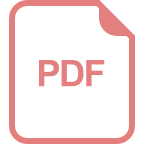
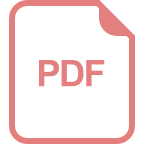