【Advanced】Advanced Techniques for Data Parsing and Extraction: Parsing XML Data with lxml
发布时间: 2024-09-15 12:29:57 阅读量: 30 订阅数: 38 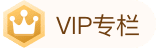
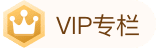
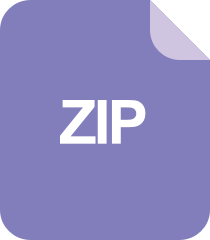
data-parsing-with-python:解析和简单的数据工作
# 1. Introduction to XML Parsing
XML (Extensible Markup Language) is a markup language extensively used for data representation and exchange. It organizes data in a tree-like structure and uses tags and attributes to describe the data. XML parsing is the process of transforming XML documents into a data structure that a computer can process.
# 2. Introduction to the lxml Library and Installation
### 2.1 Features and Advantages of the lxml Library
lxml is an open-source Python library used for parsing and manipulating XML and HTML documents. Its features and advantages include:
- **Efficient and Fast:** lxml is implemented in C, offering fast parsing and low memory consumption.
- **Rich Functionality:** lxml provides a wide range of APIs for easily loading, parsing, modifying, and transforming XML documents.
- **Strong Compatibility:** lxml supports various XML standards, including XML 1.0, XML 1.1, XPath 1.0, and XSLT 1.0.
- **Ease of Use:** The API of lxml is designed to be simple and clear, making it easy to learn and use.
- **Comprehensive Documentation:** lxml offers detailed documentation and tutorials to help users get started quickly.
### 2.2 Installation and Configuration of the lxml Library
#### 2.2.1 Installing the lxml Library
The lxml library can be installed using the following command:
```
pip install lxml
```
#### 2.2.2 Configuring the lxml Library
The lxml library requires the libxml2 and libxslt libraries, which are usually provided as system packages. These libraries may need to be installed manually on certain systems.
For Ubuntu and Debian systems:
```
sudo apt-get install libxml2-dev libxslt1-dev
```
For macOS systems:
```
brew install libxml2 libxslt
```
For Windows systems:
1. Download and install the Visual C++ Redistributable for Visual Studio 2015, 2017, and 2019.
2. Download and install the Windows binaries for libxml2 and libxslt.
3. Copy the DLL files for libxml2 and libxslt to the Lib folder under the Python installation directory.
#### 2.2.3 Verifying Installation of the lxml Library
After installation, you can verify if lxml has been successfully installed by running the following command:
```
python -c "import lxml"
```
If there is no error output, it means the lxml library has been installed successfully.
# 3.1 Loading and Parsing XML Documents
**Loading XML Documents**
The lxml library provides various ways to load XML documents, including:
- `etree.parse(file_name)`: Loads an XML document from a file.
- `etree.fromstring(xml_string)`: Loads an XML document from a string.
- `etree.XML(xml_string)`: Similar to `etree.fromstring`, but offers a more advanced API.
**Examples:**
```python
import lxml.etree as et
# Load XML document from a file
tree = et.parse('example.xml')
# Load XML document from a string
xml_string = '<root><child>Hello</child></root>'
tree = et.fromstring(xml_string)
```
**Parsing XML Documents**
After loading the XML document, you can parse it using the `etree.ElementTree` object `tree`. The `tree` object represents the entire XML document, which contains `etree.Element` objects representing elements in the document.
**Examples:**
```python
# Get the root element
root = tree.getroot()
# Get a child element
child = root.find('child')
# Get the text content of the child element
text = child.text
```
**Argument Descriptions:**
- `file_name`: The filename of the XML document to be loaded.
- `xml_string`: The string representation of the XML document to be loaded.
- `tree`: The `etree.El
0
0
相关推荐
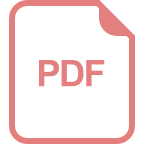
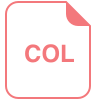
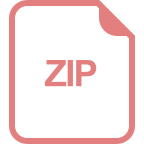
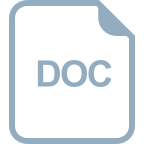
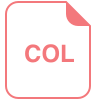
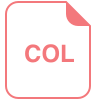
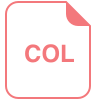