Tips for Using Table Widgets in QT: Displaying CSV Data
发布时间: 2024-09-15 10:50:22 阅读量: 22 订阅数: 23 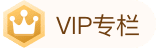
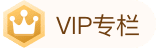
# Introduction
- **1.1 What is a QT Table Widget?**
The QT Table Widget is a control provided within the QT framework for displaying tabular data. It presents data in a table format and supports a rich set of styling options and interactive features, making it suitable for scenarios requiring the display of structured data.
- **1.2 Characteristics of CSV Data and Its Value in QT:**
CSV (Comma-Separated Values) is a common text file format where data is delimited by commas, with each line representing a record and each column representing a different field. In QT, by loading CSV data into a table widget, we can conveniently display and process large amounts of data, offering users a more intuitive and effective way of interacting with data. CSV is highly versatile, easy to generate and parse, and is often used for data exchange and storage.
# Preparations
In this chapter, we will introduce the preparations required before using the QT Table Widget to display CSV data, including importing the QT Table Widget and preparing sample data for展示 CSV data. Let's proceed step by step.
# Loading CSV Data
In this chapter, we will discuss how to load and display CSV data in QT. Loading and displaying CSV data is a common requirement in many practical applications, and it can be conveniently implemented using QT.
#### 3.1 Reading CSV Files
Firstly, we need to read the data from the CSV file. The csv module in Python or file operation libraries in other corresponding languages can be used to read CSV files. When reading CSV files, it's usually necessary to handle issues such as file encoding and delimiters.
```python
import csv
data = []
with open('sample.csv', 'r') as ***
***
***
***
```
#### 3.2 Converting CSV Data into a Format Suitable for Display in Table Widgets
Data in CSV files is typically in the form of a two-dimensional table. To display it in QT, we need to convert it into a data structure suitable for table widgets, such as a two-dimensional list or a dictionary.
```python
table_data = []
for row in data:
table_data.append(row)
```
#### 3.3 Basic Steps for Displaying CSV Data in QT
Next, we can display CSV data using the table widgets in QT. First, we need to create a table widget and set the headers and row contents.
```python
from PyQt5.QtWidgets import QApplication, QTableWidget, QTableWidgetItem, QVBoxLayout, QWidget
app = QApplication([])
widget = QWidget()
layout = QVBoxLayout()
table = QTableWidget()
table.setRowCount(len(table_data))
table.setColumnCount(len(table_data[0]))
for i, row in enumerate(table_data):
for j, col in enumerate(row):
item = QTableWidgetItem(col)
table.setItem(i, j, item)
layout.addWidget(table)
widget.setLayout(layout)
widget.show()
```
With these steps, we can display and load CSV data in QT. We will discuss how to optimize the display effect of the table in the next chapter.
# Optimizing Table Display
In this chapter, we will discuss how to optimize the display effect of table widgets, making the displayed data more intuitive and easier to understand.
#### 4.1 Setting Table Headers and Row Headers
In QT, we can increase the readability of data by setting the table widget's column headers and row headers. The following code can be used to set headers:
```python
# Setting column headers
for index, column_name in enumerate(column_names):
model.setHorizontalHeaderItem(index, QtWidgets.QTableWidgetItem(column_name))
# Setting row headers
for index, row_name in enumerate(row_names):
model.setVerticalHeaderItem(index, QtWidgets.QTableWidgetItem(row_name))
```
This allows users to clearly understand what each column and row represents when viewing the table.
#### 4.2 Adding Sorting Functionality
When displaying large amounts of data, users may need to sort based on the numerical value or alphabetical order of a column. The following code can be used to implement sorting functionality:
```python
def sort_table_column(table, col):
table.sortByColumn(col, QtCore.Qt.AscendingOrder)
```
This way, users can click on the header to sort in ascending order, and click again to sort in descending order, making it easier to view the data.
#### 4.3 Customizing Cell Styles
To give the table more aesthetic appeal and information density, we can customize the cell styles, such as setting different background colors and text colors. The following code can be used to achieve this:
```python
def set_cell_style(table, row, col, bg_color, text_color):
item = table.item(row, col)
item.setBackground(QtGui.QColor(bg_color))
item.setTextColor(QtGui.QColor(text_color))
```
By appropriately setting cell styles, we can make important data or information stand out, enhancing the user experience.
In this chapter, we introduced how to optimize the display effect of table widgets, including setting table headers and row headers, adding sorting functionality, and customizing cell styles. These techniques can make the displayed data clearer and more readable, enhancing the user experience.
# Data Processing and Interaction
In this chapter, we will discuss how to implement data processing and user interaction functions in QT to enhance the user experience of displaying CSV data in table widgets.
#### 5.1 Implementing Data Filtering Functionality
To allow users to find and filter data more conveniently, it is necessary to implement data filtering functionality in the table widget. This can be achieved by adding interactive controls such as search boxes and dropdown options above the table.
The following is an example code snippet demonstrating how to implement basic data filtering functionality in QT:
```python
# Implementing data filtering functionality
def filter_data(self):
search_text = self.search_input.text()
for row in range(self.table.rowCount()):
for col in range(self.table.columnCount()):
item = self.table.item(row, col)
if search_text.lower() in item.text().lower():
self.table.setRowHidden(row, False)
break
else:
self.table.setRowHidden(row, True)
```
#### 5.2 Responding to User Interactions: Selecting Rows, Viewing Detailed Information, etc.
In addition to data filtering functionality, it's also possible to implement features that respond to user interactions, such as displaying detailed information when a row is selected or allowing editing by double-clicking on a cell in the table.
The following is an example code snippet demonstrating how to respond to user row selection in QT:
```python
# Responding to user row selection
def on_item_clicked(self, item):
row = item.row()
col = item.column()
selected_data = []
for c in range(self.table.columnCount()):
cell_data = self.table.item(row, c).text()
selected_data.append(cell_data)
print("Selected Data:", selected_data)
```
#### 5.3 Data Export Functionality
In real-world applications, ***rovide a better user experience, data export functionality can be added to the table widget, supporting exports to CSV, Excel, and other formats.
The following is an example code snippet demonstrating how to implement data export functionality in QT:
```python
# Exporting data to a CSV file
def export_data(self):
file_name, _ = QFileDialog.getSaveFileName(self, "Save File", "", "CSV Files (*.csv)")
if file_name:
with open(file_name, 'w', newline='') as ***
***
***
*** []
for col in range(self.table.columnCount()):
item = self.table.item(row, col)
row_data.append(item.text())
writer.writerow(row_data)
```
By implementing these functions, users can more flexibly manipulate the displayed data, enhancing the interactive experience of the table widget when displaying CSV data.
This concludes the fifth chapter, which introduced methods for implementing data processing and user interaction in QT. We hope this has been helpful to you!
# Performance Optimization and Expansion
When displaying large amounts of data, to enhance the performance of the table widget and the user experience, we can adopt certain optimization strategies and expand functionalities. The following will introduce some methods:
#### 6.1 Performance Optimization Strategies for Large Data Volumes
- **Pagination Display:** When dealing with a large amount of data, consider using a paginated approach to load data, only displaying the data for the current page, reducing memory consumption and rendering time.
- **Lazy Loading:** Implement lazy loading, loading data only when the user scrolls into the visible area, reducing the performance pressure caused by loading a large amount of data at once.
- **Using Indexes:** Indexing the data can speed up the lookup and sorting processes, improving the responsiveness of the table.
#### 6.2 Expanding Functionality: Supporting Import and Export of Excel and Other Formats
In addition to supporting CSV format, the functionality of the table widget can be expanded to include the import and export of Excel and other formats.
- **Excel Import:** Data from Excel files can be imported into the table widget for display using libraries that support Excel formats.
- **Excel Export:** Provide functionality for users to export the data from the table into Excel files, making it convenient for users to view and process data in other software.
#### 6.3 Other Possible Expansions and Tips
Beyond the aforementioned functionality expansions, consider other possible features and tips:
- **Data Linkage:** Implement data linkage between table data and other controls, enhancing the user experience.
- **Custom Table Styles:** Provide users with the ability to customize table styles, allowing them to beautify the interface according to their needs.
- **Data Statistics and Analysis:** Integrate data statistics and analysis functions within the table to help users better understand and utilize the data.
By applying these performance optimizations and functional expansions, the table widget can more flexibly and efficiently handle large amounts of data and improve the user experience, meeting requirements in different scenarios.
0
0
相关推荐
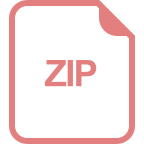
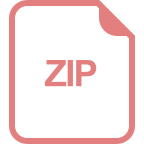
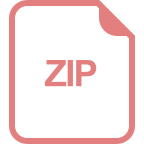
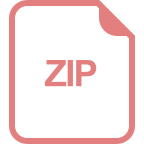
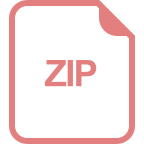
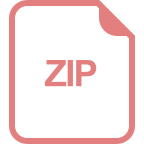
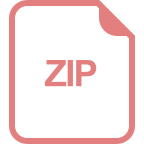
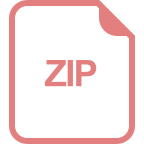