Palette Application in QT: Achieving Dynamic Color Selection
发布时间: 2024-09-15 10:59:00 阅读量: 22 订阅数: 21 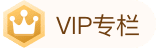
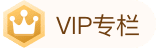
# 1. Understanding the Palette
The palette is an essential tool in QT for managing and selecting colors. In this chapter, we will introduce what a palette is, its role in QT, and its fundamental principles. Let's delve into this together.
# 2. Color Selection Controls in QT
#### 2.1 Setting the Color for QLabel
In QT, you can set the text color of a QLabel by configuring its StyleSheet property. Here is a simple example code:
```python
from PyQt5.QtWidgets import QApplication, QLabel
app = QApplication([])
label = QLabel("This is a text")
label.setStyleSheet("color: red;")
label.show()
app.exec_()
```
**Code Explanation:**
- A QLabel is created with the text content "This is a text".
- The text color is set to red using the setStyleSheet method.
- Finally, the label is displayed using the show method.
**Result of the Code:**
The text will appear in red on the interface.
#### 2.2 Setting the Color for QPushButton
For QPushButton controls, the button's background color can also be set through the StyleSheet property. Here is an example code:
```python
from PyQt5.QtWidgets import QApplication, QPushButton
app = QApplication([])
button = QPushButton("Click Me")
button.setStyleSheet("background-color: blue; color: white;")
button.show()
app.exec_()
```
**Code Explanation:**
- A QPushButton is created with the text content "Click Me".
- The button's background color is set to blue and the text color to white using the setStyleSheet method.
- Finally, the button is displayed.
**Result of the Code:**
The button's background color is blue, and the text color is white.
#### 2.3 Setting the Color for QComboBox
For QComboBox controls, you can set the background and text colors for the dropdown list. Here is a simple example code:
```python
from PyQt5.QtWidgets import QApplication, QComboBox
app = QApplication([])
combo = QComboBox()
combo.addItem("Option 1")
combo.addItem("Option 2")
combo.addItem("Option 3")
combo.addItem("Option 4")
combo.setStyleSheet("background-color: lightgrey; color: black;")
combo.show()
app.exec_()
```
**Code Explanation:**
- A QComboBox is created and several options are added.
- The dropdown list's background color is set to light grey and the text color to black using the setStyleSheet method.
- Finally, the dropdown list is displayed.
**Result of the Code:**
The background of the dropdown list is light grey, and the text color is black.
# 3. Dynamic Palettes
Palettes play a crucial role in graphical interface applications, providing users with a rich color selection functionality. In QT, we can implement real-time updates to the UI interface's color display through dynamic palettes, enhancing user experience and interactivity.
#### 3.1 Creating an Instance of a Dynamic Palette
Firstly, we need to create an instance of a dynamic palette and apply it to our interface components. In QT, this can be achieved through the QPalette class:
```python
# Python example code
# Creating a dynamic palette instance
palette = self.palette()
# Setting the palette's colors
palette.setColor(QPalette.Background, QColor(255, 0, 0)) # Sets the background color to red
self.setPalette(palette)
```
#### 3.2 Implementing Color Selection Functionality
To implement color selection functionality, we can combine QPushButton and QColorDialog to create a color selection button, allowing users to choose their preferred colors by clicking the button:
```python
# Python example code
# Creating a color selection button
color_btn = QPushButton('Choose Color',
```
0
0
相关推荐
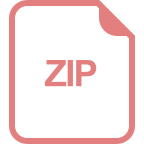
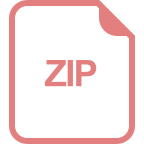
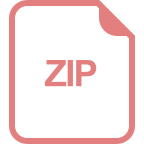
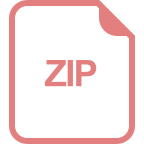
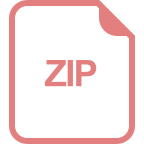
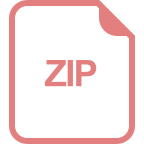
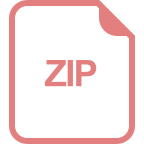
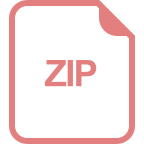
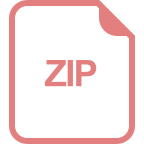