Usage and Precautions of QPainter in QT
发布时间: 2024-09-15 11:01:40 阅读量: 32 订阅数: 35 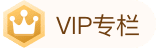
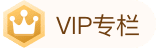
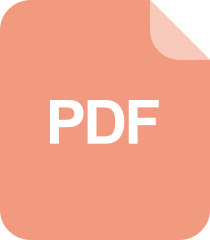
ITU-T Recommendations:Precautions at crossings
# Introduction
In QT, QPainter is an essential tool for performing drawing operations on QWidget and its subclasses. With QPainter, we can implement the drawing of various graphics, text display, and advanced drawing techniques. In this article, we will introduce the usage and precautions of QPainter in QT, to help readers better master this drawing tool.
# Initialization and Basic Drawing of QPainter
When drawing with QT, a QPainter object must first be created, and various graphics are drawn through this object. The following will introduce how to initialize the QPainter object and draw some basic graphics.
### Creating QPainter Object
Before drawing, you need to obtain a drawing device, usually a QWidget or QPixmap. Then, create a QPainter object in the paintEvent() function to perform the drawing operation.
```python
# Creating QPainter object in paintEvent() function
def paintEvent(self, event):
painter = QPainter(self)
painter.begin(self)
# Drawing content
painter.end()
```
### Drawing Basic Shapes
With the created QPainter object, it is very convenient to draw various basic shapes, such as lines, rectangles, and ellipses.
```python
# Drawing a line
painter.drawLine(10, 10, 100, 100)
# Drawing a rectangle
rect = QRect(50, 50, 100, 100)
painter.drawRect(rect)
# Drawing an ellipse
ellipse = QRect(150, 150, 100, 50)
painter.drawEllipse(ellipse)
```
Through the above code examples, we can achieve the drawing of basic shapes with the QPainter object in QT.
# Setting Up Brushes and Pens in QPainter
When using QPainter for drawing, we often need to set the properties of the brush (fill) and the pen (outline) to achieve different graphical effects. The following will introduce how to set up the relevant properties of brushes and pens.
1. **Setting the brush color and fill pattern**
```python
brush = QBrush(Qt.red, Qt.SolidPattern) # Creating a red solid brush
painter.setBrush(brush) # Setting the brush
```
The above code creates a red solid brush and sets it as the current painter's brush. Here, `Qt.SolidPattern` represents the solid fill pattern, and you can choose different fill patterns according to your needs.
2. **Setting the pen color, width, and style**
```python
pen = QPen(Qt.blue, 2, Qt.DashLine) # Creating a blue dashed pen with a width of 2
painter.setPen(pen) # Setting the pen
```
The above code creates a blue dashed pen with a width of 2 and sets it as the current painter's pen. You can set pens with different colors, widths, and styles to draw various border
0
0
相关推荐







