Qt Drawing Animation Effect: Implementation Method for Color Gradient Animation
发布时间: 2024-09-15 11:05:48 阅读量: 45 订阅数: 24 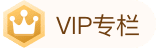
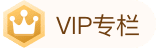
# 1. Introduction
## 1.1 What is QT Drawing Animation Effect
In QT, drawing animation effect refers to the smooth transition and dynamic effects achieved by dynamically changing the properties of graphics (such as position, color, size, etc.). QT offers a rich set of drawing and animation functionalities, making it easy to realize various cool animation effects.
## 1.2 The Role and Advantages of Color Gradient Animation
Color gradient animation is a common type of animation effect that involves changing the color gradient process of graphics, making them more vivid and appealing visually. Color gradient animation is widely used in UI design, data presentation, and other areas, enhancing the user experience and interface aesthetics. QT provides a rich set of APIs and methods, making the implementation of color gradient animation simple and flexible.
# 2. QT Drawing Fundamentals
Qt is a cross-platform C++ application development framework that offers a rich set of drawing functionalities, enabling the easy realization of various drawing effects. In QT, drawing primarily relies on components such as drawing devices (QPainter), drawing engines (QPaintEngine), and drawing systems (QPainterPath).
### 2.1 Overview of QT Drawing Framework
- **QPainter**: The core class of QT drawing, providing various drawing operation functions, such as drawing lines, rectangles, circles, etc.
- **QPaintEngine**: The QT drawing engine class, responsible for translating drawing operations into operations of the underlying graphics system.
- **QPainterPath**: The QT drawing system class, used to describe complex drawing paths, supporting functions such as Bézier curves and path combinations.
### 2.2 Methods for Drawing Colors and Gradients
In QT, the QColor class represents colors, and color objects can be created by setting RGB values or predefined color names. Simple color drawing, gradient effects, etc., can be achieved through the QBrush class, specifically including:
```java
// Drawing a red rectangle
QColor redColor = QColor(Qt::red);
QBrush redBrush(redColor);
painter.fillRect(rect, redBrush);
// Creating a linear gradient
QLinearGradient linearGrad(QPointF(0,0), QPointF(100, 100));
linearGrad.setColorAt(0, Qt::red);
linearGrad.setColorAt(0.5, Qt::green);
linearGrad.setColorAt(1, Qt::blue);
QBrush gradientBrush(linearGrad);
painter.fillRect(150, 0, 100, 100, gradientBrush);
```
The above code demonstrates how to draw red rectangles and linear gradient colored rectangles in QT. By setting different colors and gradient points, a variety of colorful drawing effects can be achieved.
# 3. Implementing Animation Effects
In this chapter, we will introduce how to use the QT animation framework to realize color gradient animation effects.
#### 3.1 Introduction to QT Animation Framework
The QT framework offers a rich set of animation classes to realize various animation effects, including QPropertyAnimation, QVariantAnimation, etc. These classes can be used to dynamically change property values
0
0
相关推荐
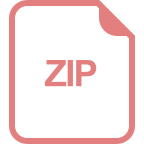
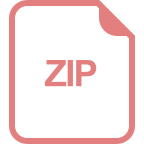
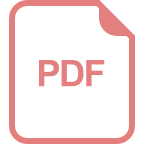
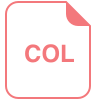
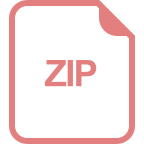
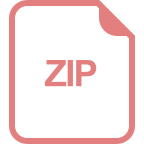
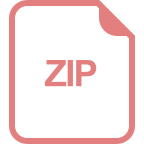
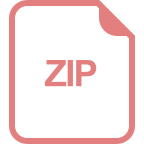
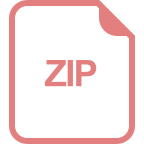