【Basic】Signal Filtering in MATLAB: Implementing Low-pass, High-pass, and Band-pass Filters
发布时间: 2024-09-14 05:47:40 阅读量: 19 订阅数: 41 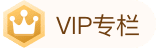
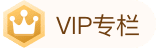
# 2.1 Low-Pass Filter Design
Low-pass filters allow low-frequency components to pass through while attenuating high-frequency components. In MATLAB, the `butter` function can be used to design low-pass filters. The `butter` function takes three parameters:
- **N:** The order of the filter
- **Wn:** The cutoff frequency (normalized to the unit circle)
- **type:** The type of filter ('low', 'high', 'bandpass', 'bandstop')
For a low-pass filter, the `type` parameter is set to 'low'. For example, the following code designs a low-pass filter with an order of 5 and a cutoff frequency of 0.2:
```
N = 5;
Wn = 0.2;
[b, a] = butter(N, Wn, 'low');
```
Here, `b` and `a` are the numerator and denominator polynomial coefficients of the filter, respectively.
# 2. MATLAB Filter Design
### 2.1 Low-Pass Filter Design
Low-pass filters permit low-frequency signals to pass while attenuating high-frequency signals. MATLAB provides two common methods for designing low-pass filters: Butterworth filters and Chebyshev filters.
#### 2.1.1 Butterworth Low-Pass Filter
The transfer function of a Butterworth low-pass filter is:
```
H(s) = 1 / (1 + (s/ωc)^2n)
```
Where:
* `ωc` is the cutoff angular frequency
* `n` is the order of the filter
**Parameter Description:**
| Parameter | Description |
|---|---|
| `ωc` | Cutoff angular frequency, in rad/s |
| `n` | Order of the filter, which determines the filter's steepness |
**Code Block:**
```
% Designing a Butterworth low-pass filter
[b, a] = butter(n, ωc);
```
**Logical Analysis:**
The `butter` function is used to design a Butterworth filter. It requires two parameters: the order of the filter `n` and the cutoff angular frequency `ωc`. The function returns two coefficient vectors `b` and `a`, which are used to represent the filter's transfer function.
#### 2.1.2 Chebyshev Low-Pass Filter
The transfer function of a Chebyshev low-pass filter is:
```
H(s) = 1 / (1 + ε^2 * (s/ωc)^2n * C_n(s/ωc))
```
Where:
* `ωc` is the cutoff angular frequency
* `n` is the order of the filter
* `ε` is the passband ripple
* `C_n` is the nth-order Chebyshev polynomial
**Parameter Description:**
| Parameter | Description |
|---|---|
| `ωc` | Cutoff angular frequency, in rad/s |
| `n` | Order of the filter, which determines the filter's steepness |
| `ε` | Passband ripple, indicating the maximum permissible gain deviation within the passband |
**Code Block:**
```
% Designing a Chebyshev low-pass filter
[b, a] = cheby1(n, ε, ωc);
```
**Logical Analysis:**
The `cheby1` function is used to design a Chebyshev filter. It requires three parameters: the order of the filter `n`, the passband ripple `ε`, and the cutoff angular frequency `ωc`. The function returns two coefficient vectors `b` and `a`, which are used to represent the filter's transfer function.
# 3. MATLAB Filter Implementation
### 3.1 Filters Applied to Time-Domain Signals
#### 3.1.1 Low-Pass Filter Application
**Code Block:**
```
% Importing time-domain signal
signal = load('signal.mat');
signal = signal.signal;
% Designing a low-pass filter
Fs = 1000; % Sampling frequency
Fpass = 100; % Passband cutoff frequency
Fstop = 200; % Stopband cutoff frequency
Apass = 1; % Passband attenuation
Astop = 60; % Stopband attenuation
N = 6; % Filter order
[b, a] = butter(N, Fpass/(Fs/2), 'low');
% Applying
```
0
0
相关推荐





