【Practical Exercise】 Simulation Design of Direct Sequence Spread Spectrum Communication System Based on MATLAB
发布时间: 2024-09-14 07:17:26 阅读量: 43 订阅数: 62 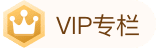
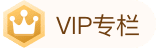
# 2.1 System Parameter Configuration and Initialization
The first step in modeling a DS-CDMA system in MATLAB is to configure and initialize the system parameters. This includes setting the following parameters:
- **Carrier Frequency (fc)**: The frequency of the signal's carrier.
- **Chip Rate (Rc)**: The rate of the pseudo-random code.
- **Spreading Factor (SF)**: The ratio of the bandwidth of the spread signal to the original signal bandwidth.
- **Number of Users (K)**: The number of users in the system.
- **Signal-to-Noise Ratio (SNR)**: The signal-to-noise ratio of the channel.
These parameters can be configured using the following code:
```
% Set system parameters
fc = 1e9; % Carrier frequency (Hz)
Rc = 1e6; % Chip rate (Hz)
SF = 128; % Spreading factor
K = 10; % Number of users
SNR = 10; % Signal-to-noise ratio (dB)
% Initialize system
system = struct('fc', fc, 'Rc', Rc, 'SF', SF, 'K', K, 'SNR', SNR);
```
# 2. MATLAB Modeling of DS-CDMA Systems
### 2.1 System Parameter Configuration and Initialization
Modeling a DS-CDMA system in MATLAB requires first configuring and initializing the system parameters. This includes setting the following parameters:
- **Carrier Frequency (fc)**: The carrier frequency used by the system, in Hertz (Hz).
- **Chip Rate (Rc)**: The chip rate of the spreading sequence, in chips per second.
- **Spreading Factor (SF)**: The length of the spreading sequence, equal to the ratio of chip rate to symbol rate.
- **Number of Users (K)**: The number of users communicating simultaneously in the system.
- **Signal-to-Noise Ratio (SNR)**: The channel signal-to-noise ratio, in decibels (dB).
The following code example demonstrates how to configure and initialize system parameters:
```matlab
% System parameter configuration
fc = 2e9; % Carrier frequency, 2 GHz
Rc = 1e6; % Chip rate, 1 Mbps
SF = 10; % Spreading factor
K = 10; % Number of users
SNR = 10; % Signal-to-noise ratio, 10 dB
```
### 2.2 Pseudo-Random Code Generation and Spreading
In a DS-CDMA system, each user uses a unique pseudo-random noise (PN) sequence for spreading. A PN sequence is a binary sequence with good auto-correlation and cross-correlation properties.
In MATLAB, the `pnseq` function can be used to generate PN sequences. This function requires two parameters:
- **Length (n)**: The length of the PN sequence.
- **Initial State (s)**: The initial state of the PN sequence.
The following code example demonstrates how to generate a PN sequence of length 1023:
```matlab
% PN sequence generation
n = 1023; % PN sequence length
s = 1; % Initial state
pn_sequence = pnseq(n, s);
```
The spreading process involves performing an XOR operation between the PN sequence and the data signal to be transmitted. This extends the bandwidth of the data signal to the chip rate bandwidth.
The following code example demonstrates how to spread a data signal:
```matlab
% Spreading
data_signal = [1, 0, 1, 1, 0, 1]; % Data signal
spread_signal = xor(data_signal, pn_sequence); % Spread signal
```
### 2.3 Signal Modulation and Demodulation
The spread signal needs to be modulated to transmit it into the channel. The `modulate` function can be used for modulation in MATLAB. This function requires three parameters:
- **Signal**: The signal to be modulated.
- **Modulation Type**: The type of modulation, such as `pskmod` (Phase Shift Keying Modulation).
- **Modulation Order**: The order of modulation, such as 2 (Binary Phase Shift Keying).
The following code example demonstrates how to perform Binary Phase Shift Keying modulation on a spread signal:
```matlab
% Modulation
modulated_signal = modulate(spread_signal, 'pskmod', 2);
```
The demodulation process involves performing a correlation operation between the received signal and the known PN sequence. This restores the original data signal.
The `correlate` function can be used for correlation in MATLAB. This function requires t
0
0