【Practical Exercise】Basic Data Compression and Encoding with MATLAB
发布时间: 2024-09-14 06:59:25 阅读量: 26 订阅数: 61 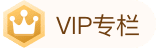
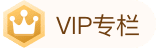
# 2.1 Fundamental Principles of Data Compression
Data compression is a technique that reduces the size of data files to save space when storing or transmitting. It achieves this by eliminating redundancy and unnecessary information in the data. Data compression algorithms are divided into two major categories:
### 2.1.1 Lossless Compression and Lossy Compression
***Lossless Compression:** Data can be fully restored to its original state after compression without losing any information. Examples include Huffman coding and Lempel-Ziv coding.
***Lossy Compression:** Data cannot be fully restored after compression, resulting in the loss of some information. However, lossy compression can achieve higher compression rates. Examples include JPEG image compression.
### 2.1.2 Classification of Compression Algorithms
Data compression algorithms can be further classified based on their operational methods:
***Statistical Coding:** Coding based on the statistical characteristics of the data, such as Huffman coding.
***Dictionary Coding:** Using a dictionary to replace repeated sequences in the data with shorter codes, such as Lempel-Ziv coding.
***Arithmetic Coding:** Representing data as a fraction and then encoding it using arithmetic operations.
# 2. MATLAB Data Compression Theory and Algorithms
### 2.1 Fundamental Principles of Data Compression
**2.1.1 Lossless Compression and Lossy Compression**
Data compression is divided into lossless and lossy compression. Lossless compression refers to the ability to completely restore compressed data to its original state, while lossy compression means that some information will be lost after compression, but more storage space can be saved.
**2.1.2 Classification of Compression Algorithms**
Compression algorithms can be classified into the following categories:
- **Statistical Coding:** Coding based on the statistical characteristics of the data, such as Huffman coding and Lempel-Ziv coding.
- **Dictionary Coding:** Coding using predefined dictionaries, such as arithmetic coding.
- **Transform Coding:** Transforming data into another domain and then encoding it in that domain, such as JPEG and MPEG.
### 2.2 Common Data Compression Algorithms
#### 2.2.1 Huffman Coding
Huffman coding is a lossless compression algorithm that constructs a binary tree based on the frequency of data, assigning shorter codes to symbols that appear more frequently and longer codes to those that appear less frequently.
**Code Block:**
```matlab
% Original data
data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
% Frequency table
freq = [0.1, 0.2, 0.3, 0.4, 0.5, 0.6, 0.7, 0.8, 0.9, 1.0];
% Construct Huffman tree
tree = huffmantree(freq);
% Encode data
encodedData = huffmanenco(data, tree);
```
**Logical Analysis:**
* The `huffmantree` function constructs a Huffman tree based on the frequency table.
* The `huffmanenco` function uses the Huffman tree to encode data.
**Parameter Description:**
* `freq`: Frequency table, representing the probability of each symbol's occurrence.
* `tree`: Huffman tree, representing the encoding rules.
* `data`: Data to be encoded.
* `encodedData`: Data after encoding.
#### 2.2.2 Lempel-Ziv Coding
Lempel-Ziv coding is a lossless compression algorithm that compresses data by finding and replacing repeated substrings.
**Code Block:**
```matlab
% Original data
data = 'abracadabra';
% Lempel-Ziv encoding
encodedData = lzw(data);
```
**Logical Analysis:**
* The `lz
0
0
相关推荐
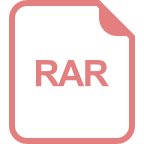
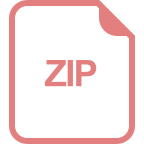
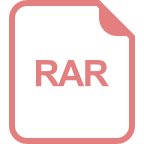
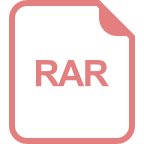
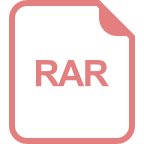
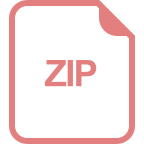
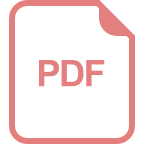
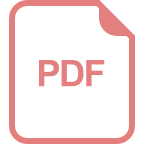