[Practical Exercise] Simulation of Frequency Hopping Communication Fundamental Principles Based on MATLAB
发布时间: 2024-09-14 07:16:10 阅读量: 84 订阅数: 71 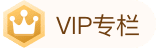
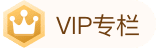
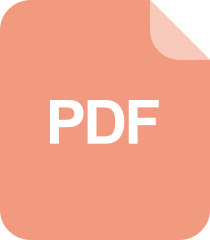
Simulation of Screening Process Based on MATLAB_Simulink.pdf
# 1. Frequency Hopping Communication Fundamental Principles
Frequency hopping communication is a spread spectrum communication technology that improves the resistance to interference by rapidly changing the carrier frequency. The basic principles are as follows:
***Rapid Carrier Frequency Hopping:** Frequency hopping communication uses multiple carrier frequencies and switches between these frequencies at a certain rate. This hopping makes it difficult for interference signals to concentrate on a specific frequency.
***Spread Spectrum:** By using multiple carrier frequencies, frequency hopping communication expands the signal bandwidth, thus reducing the energy density of interference signals.
***Interference Resistance:** Since interference signals find it hard to concentrate on a particular frequency, frequency hopping communication possesses strong resistance to interference.
# 2. MATLAB Frequency Hopping Communication Simulation Basics
### 2.1 Signal Generation and Processing in MATLAB
MATLAB is a powerful numerical computing and visualization environment, widely used in signal processing, image processing, and communication fields. In frequency hopping communication simulations, MATLAB offers a rich set of functions for signal generation and processing, including generating frequency-hopping signals, adding noise and interference, and analyzing and visualizing simulation results.
**Signal Generation**
* `randn(m, n)`: Generates an m by n matrix of normal distribution random values with a mean of 0 and variance of 1.
* `randi(n, m, p)`: Generates an m by p matrix of random integers within the range [1, n].
* `sin(x)`: Calculates the sine of x.
* `cos(x)`: Calculates the cosine of x.
**Signal Processing**
* `fft(x)`: Computes the discrete Fourier transform of x.
* `ifft(x)`: Computes the inverse discrete Fourier transform of x.
* `filter(b, a, x)`: Filters the signal x using the transfer function b/a.
* `conv(x, h)`: Computes the convolution of x and h.
### 2.2 Generation of Frequency Hopping Communication Signals
A frequency hopping communication signal is one that transmits information by rapidly changing the carrier frequency. In MATLAB, you can generate a frequency-hopping signal using the following steps:
**Code Block 1: Frequency Hopping Signal Generation**
```matlab
% Carrier frequency range
carrier_freq_range = [1e6, 10e6];
% Hopping rate
hop_rate = 1000;
% Sampling rate
fs = 100e3;
% Duration
duration = 1;
% Generate carrier frequency sequence
carrier_freq_sequence = randi(carrier_freq_range, 1, duration * fs / hop_rate);
% Generate sine wave carrier
carrier = sin(2 * pi * carrier_freq_sequence / fs * (0:duration * fs - 1));
% Generate data sequence
data = randi([0, 1], 1, duration * fs / hop_rate);
% Modulate the data
modulated_signal = carrier .* data;
```
**Code Logic Analysis:**
* `carrier_freq_range` defines the carrier frequency range.
* `hop_rate` sets the hopping rate.
* `fs` is the sampling rate.
* `duration` is the signal duration.
* `carrier_freq_sequence` is the randomly generated carrier frequency sequence.
* `carrier` is the sine wave carrier.
* `data` is the randomly generated data sequence.
* `modulated_signal` is the modulated frequency-hopping signal.
### 2.3 Setting Up the Simulation Environment
Before conducting frequency hopping communication simulations, it is necessary to set up a simulation environment, including a channel model, a noise model, and an interference model.
**Channel Model**
***AWGN Channel:** An additive white Gaussian noise channel with a constant noise power spectral density.
***Rayleigh Fading Channel:** A channel model that simulates the fading effects of wireless channels.
**Noise Model**
***Gaussian Noise:** Normal distribution noise with a mean of 0 and variance of σ^2.
***Uniform Noise:** Noise uniformly distributed within the range [-A, A].
**Interference Model**
***Narrowband Interference:** An interference signal with a narrow frequency range and high power spectral density.
***Wideband Interference:** An interference signal with a wide frequency range and lower power spectral density.
**Code Block 2: Setting Up the Simulation Environment**
```matlab
% Channel model
channel_model = 'AWGN';
% Noise model
noise_model = 'Gaussian';
% Interference model
interference_model = 'Narrowband';
% Noise power spectral density
noise_psd = -100; % dBm/Hz
% Interference power
interference_power = -90; % dBm
% Channel parameters
channel_params = struct('noise_psd', noise_psd, 'interference_power', interference_power);
```
**Code Logic Analysis:**
* `channel_model` specifies the channel model.
* `noise_model` specifies the noise model.
* `interference_model` specifies the interference model.
* `noise_psd` is the noise power spectral density.
* `interference_power` is the interference power.
* `channel_params` is a structure containing channel parameters.
# 3. Practical Frequency Hopping Communication Simulation
### 3.1 Establishing a Frequency Hopping Communication System Model
Establishing a frequency hopping communication system model is the foundation of simulation practice. In MATLAB, the `comm.FHSS` class can be used to construct a frequency hopping communication system model. The `comm.FHSS` class provides common parameter settings for the frequency hopping communication system, including hopping mode, hopping rate, carrier frequency, modulation method, etc.
```matlab
% Create a frequency hopping communication system model
fhss = comm.FHSS(...
'FrequencyHoppingPattern', 'Random', ...
'HopPeriod', 0.01, ...
'CarrierFrequency', 100e6, ...
'Modulation', 'BPSK');
```
**Parameter Description:**
* `FrequencyHoppingPattern`: Hopping mode, which can be `Random` (random hopping), `Sequence` (sequential hopping), or `Custom` (custom hopping).
* `HopPeriod`: Hopping rate, in seconds.
* `CarrierFrequency`: Carrier frequency, in Hertz.
* `Modulation`: Modulation method, which can be `
0
0
相关推荐
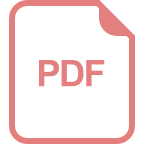
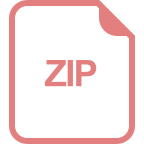
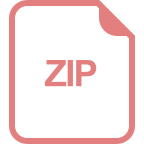
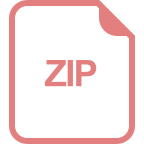
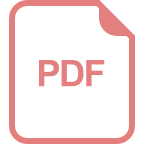
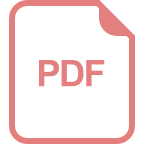
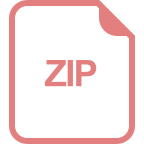