【Practical Exercise】Design of Basic AM Modulation and Demodulation System Using MATLAB
发布时间: 2024-09-14 06:40:01 阅读量: 48 订阅数: 62 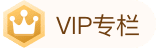
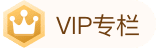
# 1. Introduction to MATLAB and Basic Knowledge
MATLAB (Matrix Laboratory) is an advanced programming language and interactive environment for technical computing. Developed by MathWorks, it is widely used in fields such as science, engineering, mathematics, and finance.
The features of MATLAB include:
- **Powerful matrix operations:** MATLAB excels in handling matrices and arrays and provides a rich set of matrix operation functions.
- **Extensive toolboxes:** MATLAB offers a wide range of toolboxes covering areas such as signal processing, image processing, control systems, and machine learning, providing rich functionality for specific applications.
- **Interactive environment:** MATLAB provides an interactive environment that allows users to input commands directly and receive immediate feedback, facilitating code development and debugging.
- **Visualization capabilities:** MATLAB has strong visualization tools that make it easy to create a variety of charts and graphs, aiding in data analysis and result presentation.
# 2. AM Modulation Principle and MATLAB Implementation
### 2.1 Basic Principles of AM Modulation
#### 2.1.1 Double-Sideband Modulation
Double-sideband modulation (DSB) is a modulation technique where the spectrum of the modulating signal is copied to both sides of the carrier signal. This modulation results in a modulation signal with a bandwidth that is twice the bandwidth of the modulating signal.
**Mathematical representation:**
```
s_AM(t) = s_m(t) * cos(2πf_c t)
```
Where:
* `s_AM(t)` is the modulation signal
* `s_m(t)` is the modulating signal
* `f_c` is the carrier frequency
**Spectrum diagram:**
[Image of DSB Spectrum]
#### 2.1.2 Single-Sideband Modulation
Single-sideband modulation (SSB) is a modulation technique where the spectrum of the modulating signal is copied to only one side of the carrier signal. This results in a modulation signal with a bandwidth that is half the bandwidth of the modulating signal.
**Mathematical representation:**
```
s_SSB(t) = s_m(t) * cos(2πf_c t + θ)
```
Where:
* `s_SSB(t)` is the modulation signal
* `s_m(t)` is the modulating signal
* `f_c` is the carrier frequency
* `θ` is the phase shift
**Spectrum diagram:**
[Image of SSB Spectrum]
### 2.2 AM Modulation Implementation in MATLAB
#### 2.2.1 Generation of the Modulating Signal
```
% Define modulating signal parameters
f_m = 100; % Modulating signal frequency (Hz)
A_m = 1; % Modulating signal amplitude
% Generate the modulating signal
t = 0:0.001:1; % Time vector
s_m = A_m * sin(2 * pi * f_m * t);
```
#### 2.2.2 Generation of the Carrier Signal
```
% Define carrier signal parameters
f_c = 1000; % Carrier frequency (Hz)
A_c = 1; % Carrier signal amplitude
% Generate the carrier signal
s_c = A_c * cos(2 * pi * f_c * t);
```
#### 2.2.3 Generation of the AM Modulation Signal
```
% Define modulation index
m = 0.5; % Modulation index
% Generate the AM modulation signal
s_AM = (1 + m * s_m) .* s_c;
```
**Code logic analysis:**
* `m` is the modulation index, which controls the effect of the modulating signal on the carrier signal.
* The term `(1 + m * s_m)` represents the modulation of the carrier signal by the modulating signal. When `m` is 0, there is no modulation, and the modulation signal is the carrier signal. As `m` increases, the effect of the modulating signal on the carrier signal also increases.
* The `.*` operator multiplies the modulating signal with the carrier signal to produce the AM modulation signal.
# 3. AM Demodulation Principle and MATLAB Implementation
### 3.1 Basic Principles of AM Demodulation
AM demodulation is the process of recovering the original modulating signal from the AM modulation signal. There are two main techniques for AM demodulation: envelope detection and coherent demodulation.
#### 3.1.1 Envelope Detection
Envelope detection is a simple and commonly used AM demodulation technique. It takes advantage of the fact that the envelope of the AM modulation signal contains information about the original modulating signal. The envelope detector works in the following steps:
1. **Rectification:** The AM modulation signal is rectified into a positive half-wave signal.
2. **Filtering:** A low-pass filter is used to remove high-frequency components, retaining the envelope.
3. **Amplification:** The envelope signal is amplified to restore the original modulating signal.
#### 3.1.2 Coherent Demodulation
Coherent demodulation is a more complex AM demodulation technique that requires knowledge of th
0
0
相关推荐
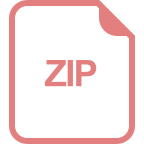
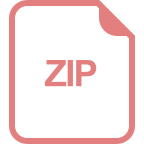
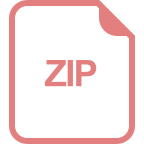
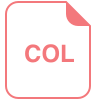
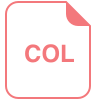
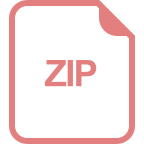
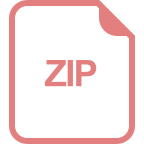
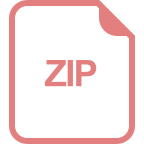
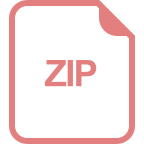