[Advanced Chapter] Implementation and Simulation of Turbo Codes and LDPC Codes in MATLAB
发布时间: 2024-09-14 06:14:01 阅读量: 14 订阅数: 40 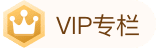
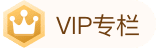
# 1. Basics of Turbo Codes and LDPC Codes
Turbo codes and LDPC (Low-Density Parity-Check) codes are both widely-used error correction coding technologies in modern communication systems. They possess robust error correction capabilities, allowing for the reliable transmission of data even under poor channel conditions.
**Turbo Codes** are a type of Serial-Serial Parallel-Serial (SSCC) encoder, consisting of two parallel concatenated convolutional codes. The encoding process of Turbo codes involves:
1. Data bits are inputted into the first convolutional code for encoding.
2. The encoded bits are then interleaved to break the correlation between data bits.
3. The interleaved bits are fed into the second convolutional code for further encoding.
4. The output bits from both convolutional codes are combined to form the Turbo code codeword.
# 2. Implementing Turbo Codes in MATLAB
Turbo codes are a powerful error correction coding technology that has been widely applied in various communication systems. MATLAB provides a rich set of toolboxes and functions to easily implement encoding and decoding of Turbo codes.
### 2.1 Turbo Code Encoder
A Turbo code encoder is composed of two parallel concatenated convolutional encoders, known as constituent encoders. Each constituent encoder is defined by a generator polynomial and a puncturing pattern.
In MATLAB, the `comm.TurboEncoder` class can be used to create a Turbo code encoder. This class has the following parameters:
* `ConstituentEncoders`: A cell array containing two convolutional encoder objects that define the constituent encoders.
* `Interleaver`: An interleaver object used to interleave the input data.
* `PuncturingPattern`: A vector specifying the puncturing pattern.
The following code example demonstrates how to create a Turbo code encoder using the `comm.TurboEncoder` class:
```
% Define constituent encoders
constituentEncoders = {comm.ConvolutionalEncoder('polynomial', [133 171], 'trellisStructure', poly2trellis(7, [133 171]));
comm.ConvolutionalEncoder('polynomial', [133 171], 'trellisStructure', poly2trellis(7, [133 171]))};
% Define interleaver
interleaver = comm.BlockInterleaver('BlockSize', 256);
% Define puncturing pattern
puncturingPattern = [1 1 0 1];
% Create Turbo code encoder
turboEncoder = comm.TurboEncoder('ConstituentEncoders', constituentEncoders, 'Interleaver', interleaver, 'PuncturingPattern', puncturingPattern);
```
### 2.2 Turbo Code Decoder
The Turbo code decoder employs an iterative decoding algorithm known as the Log-MAP algorithm to estimate the encoded data. MATLAB provides the `comm.TurboDecoder` class for Turbo code decoding.
The `comm.TurboDecoder` class has the following parameters:
* `ConstituentDecoders`: A cell array containing two convolutional decoder objects that define the constituent decoders.
* `Interleaver`: An interleaver object used to interleave the input data.
* `DecisionType`: Specifies the type of decision used by the decoder (hard decision or soft decision).
* `NumIterations`: Specifies the number of iterations for the decoder.
The following code example demonstrates how to create a Turbo code decoder using the `comm.TurboDecoder` class:
```
% Define constituent decoders
constituentDecoders = {comm.ViterbiDecoder('polynomial', [133 171], 'trellisStructure', poly2trellis(7, [133 171]));
comm.ViterbiDecoder('polynomial', [133 171], 'trellisStructure', poly2trellis(7, [133 171]))};
% Define interleaver
interleaver = comm.BlockInterleaver('BlockSize', 256);
% Create Turbo code decoder
turboDecoder = comm.TurboDecoder('ConstituentDecoders', constituentDecoders, 'Interleaver', interleaver, 'DecisionType', 'hard', 'NumIterations', 10);
```
### 2.3 Turbo Code Performance Evaluation
The performance of Turbo codes can be evaluated through Bit Error Rate (BER) and Frame Error Rate (FER). MATLAB provides the `comm.BERTool` and `comm.FERTool` classes for calculating BER and FER.
The
0
0
相关推荐
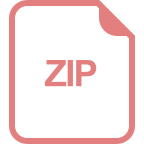
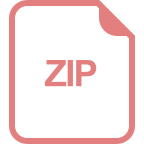
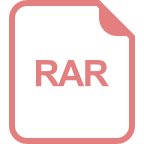
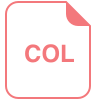
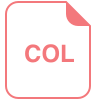
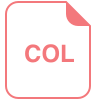
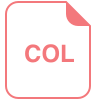

