[Practical Exercise] DSP Basic Application Development with MATLAB
发布时间: 2024-09-14 07:07:25 阅读量: 96 订阅数: 83 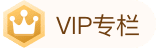
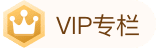
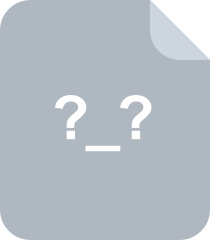
Practical Azure Application Development epub
# 1. MATLAB Basics
MATLAB (Matrix Laboratory) is a high-level programming language and interactive environment for technical computing. It is renowned for its powerful matrix manipulation capabilities, making it a widely used tool in the fields of science, engineering, and mathematics. MATLAB offers an interactive command-line interface that allows users to input commands, perform calculations, and view results. It also includes a Graphical User Interface (GUI) that provides access to various tools and features such as the variable browser, editor, and debugger.
# 2. DSP Theory Fundamentals
### 2.1 Concept of Digital Signal Processing
**Definition:**
Digital Signal Processing (DSP) is a technique for processing discrete-time signals and systems, where both the signal and the system are represented in digital form.
**Characteristics:**
***Discreteness:** DSP processes signals that are discretized in time and amplitude.
***Digital Representation:** Signals and systems are represented using binary digits.
***Algorithmic Processing:** DSP algorithms are used for processing and analyzing digital signals.
### 2.2 Discrete-Time Signals and Systems
#### 2.2.1 Time-Domain and Frequency-Domain Analysis of Discrete-Time Signals
**Time-Domain Analysis:**
***Sampling:** Converts continuous-time signals into discrete-time signals.
***Discrete-Time Signals:** Signals defined at discrete time points.
***Difference Equations:** Equations describing the time-domain behavior of discrete-time signals.
**Frequency-Domain Analysis:**
***Discrete Fourier Transform (DFT):** Converts discrete-time signals into frequency-domain representations.
***Magnitude Spectrum:** Displays the magnitudes of the signal's frequency components.
***Phase Spectrum:** Displays the phases of the signal's frequency components.
#### 2.2.2 Time-Domain and Frequency-Domain Analysis of Discrete-Time Systems
**Time-Domain Analysis:**
***Impulse Response:** Output of the system in response to a unit impulse.
***Convolution:** Describes the relationship between the system's output and input signals.
***Difference Equations:** Describes the time-domain behavior of discrete-time systems.
**Frequency-Domain Analysis:**
***Frequency Response:** Function describing how the system's output magnitude and phase vary with frequency.
***Transfer Function:** Mathematical expression describing the system's frequency response.
***Zero-Pole Plot:** Displays the zeros and poles of the system's transfer function.
**Code Block:**
```matlab
% Sampling a continuous-time signal
fs = 1000; % Sampling rate
t = 0:1/fs:1; % Time vector
x = sin(2*pi*100*t); % Sine signal
% Compute DFT
X = fft(x);
% Plot magnitude spectrum
figure;
plot(abs(X));
title('Magnitude Spectrum');
```
**Logical Analysis:**
* The `fft` function computes the Discrete Fourier Transform.
* The `abs` function takes the absolute value of a complex number, obtaining the magnitude spectrum.
* Plotting the magnitude spectrum displays the frequency components of the signal.
**Parameter Explanation:**
* `fs`: Sampling rate.
* `t`: Time vector.
* `x`: Continuous-time signal.
* `X`: Result of DFT.
# 3. Basic DSP Operations in MATLAB
### 3.1 Signal Generation and Processing
#### 3.1.1 Signal Generation Functions
MATLAB provides a rich set of signal generation functions capable of producing various types of signals, including sine waves, square waves, sawtooth waves, Gaussian noise, and white noise. These functions are very convenient for quickly generating the necessary signals for analysis and processing.
**Common Signal Generation Functions:**
| Function | Description |
|---|---|
| `sin` | Generates sine waves |
| `cos` | Generates cosine waves |
| `square` | Generates square waves |
| `sawtooth` | Generates sawtooth waves |
| `randn` | Generates Gaussian noise |
| `rand` | Generates white noise |
**Code Example:**
```matlab
% Generate a sine wave with a frequency of 100Hz
t = 0:0.01:1; % Time vector
y = sin(2*pi*100*t); % Sine wave signal
% Plot the sine wave
plot(t, y);
xlabel('Time (s)');
ylabel('Amplitude');
title('Sine Wave Signal');
```
#### 3.1.2 Signal Processing Functions
MATLAB also offers a multitude of signal processing functions that can perform various operations on signals, such as filtering, convolution, correlation, Fourier transforms, etc. These functions can help users process signals efficiently and extract useful information.
**Common Signal Processing Functions:**
| Function | Description |
|---|---|
| `filter` | Filters a signal |
| `conv` | Performs convolution on a signal |
| `xcorr` | Correlates a signal |
| `fft` | Performs a Fourier transform on a signal |
| `ifft` | Performs an inverse Fourier transform on a signal |
**Code Example:**
```matlab
% Apply low-pass filtering to a sine wave signal
Fs = 1000; % Sampling frequency
Fpass = 100; % Passband cutoff frequency
Fstop = 200; % Stopband cutoff frequency
Wn = [Fpass Fstop] / (Fs
```
0
0
相关推荐







