[Practical Exercise] Simulation of BCH Coding and Decoding Using MATLAB
发布时间: 2024-09-14 07:10:38 阅读量: 44 订阅数: 66 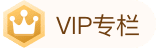
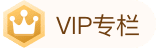
# 1. Fundamental Theory of BCH Coding
BCH coding is a non-binary cyclic error-correcting code with high error correction capability and low encoding complexity, widely used in communication, storage, and data transmission fields. The theoretical foundation of BCH coding is built upon finite field algebra and cyclic code theory.
A BCH code is a type of cyclic code based on the finite field GF(2^m). The finite field GF(2^m) is a field containing 2^m elements, where each element can be represented as an m-bit binary vector. The generator polynomial of a BCH code is an irreducible polynomial over GF(2^m) with degree n. The encoder performs a convolution operation between the information sequence and the generator polynomial to obtain an n-bit codeword.
# 2. BCH Coding Simulation in MATLAB
### 2.1 Design of BCH Encoder
**2.1.1 Selection of Generator Polynomial**
The first step in designing a BCH encoder is to choose a generator polynomial. The generator polynomial is a binary polynomial with degree n-k, where n is the length of the codeword and k is the number of information bits.
The choice of generator polynomial is crucial as it determines the error correction capability of the BCH code. Typically, an irreducible polynomial is chosen as the generator polynomial to ensure good error correction performance of the BCH code.
In MATLAB, the `gfprim` function can be used to generate an irreducible polynomial. This function takes an integer parameter representing the order of the polynomial and returns an irreducible polynomial.
```matlab
% Generate a 7th order irreducible polynomial
p = gfprim(7);
```
**2.1.2 Implementation of Encoder**
Once the generator polynomial has been chosen, the BCH encoder can be implemented. The BCH encoder is a convolutional encoder that performs a convolution operation between the information bit sequence and the generator polynomial to produce a codeword.
In MATLAB, the `conv` function can be used to implement the BCH encoder. This function takes two polynomials as inputs and returns their convolution result.
```matlab
% Information bit sequence
u = [1 0 1 1 0 1];
% Encoder implementation
v = conv(u, p);
```
### 2.2 Design of BCH Decoder
**2.2.1 Selection of Decoding Algorithm**
The first step in designing a BCH decoder is to choose a decoding algorithm. There are two main BCH decoding algorithms: the Berlekamp-Massey algorithm and the Euclidean algorithm.
The Berlekamp-Massey algorithm is an iterative algorithm that uses feedback shift registers (FSRs) to compute the error locator polynomial required for error correction. The Euclidean algorithm is a recursive algorithm that uses Euclidean division to compute the error locator polynomial.
In MATLAB, the `bchdec` function can be used to implement the Berlekamp-Massey algorithm. This function takes the codeword and the generator polynomial as inputs and returns the information bit sequence after error correction.
```matlab
% Decoding algorithm implementation
u_hat = bchdec(v, p);
```
**2.2.2 Implementation of Decoder**
Once the decoding algorithm has been chosen, the BCH decoder can be implemented. The BCH decoder is an iterative process that uses the decoding algorithm to compute the error locator polynomial required for error correction and then uses this polynomial to correct errors in the codeword.
In MATLAB, the `bchdec` function can be used to implement the BCH decoder. This function takes the codeword and the generator polynomial as inputs and returns the information bit sequence after error correction.
```matlab
% Decoder implementation
u_hat = bchdec(v, p);
```
# 3.1 Analysis of Coding Efficiency
Coding efficiency is an important metric for evaluating the performance of BCH coding, indicating the ratio of the length of the codeword after coding to the original information length. The higher the coding efficiency, the shorter the length of the codeword after coding, and the higher the transmission efficiency.
**Formula for calculating coding efficiency:**
```
Coding efficiency = Original information length / Codeword length
```
**Factors affecting coding efficiency:**
***Codeword length:** The longer the codeword length, the lower the coding efficiency.
***Error correction capability:** The stronger the error correction capability, the longer the codeword length, and the lower the coding efficiency.
***Generator polynomial:** Different generator polynomials result in different codeword lengths, thereby affecting coding efficiency.
**Methods to improve coding efficiency:**
***Choose an appropriate codeword length:** Select the shortest codeword length that can meet the error correction requirements based on the actual application scenario.
***Optimize generator polynomials:** Choose generator polynomials that can produce shorter codeword lengths.
***Use Low-Density Parity-Check (LDPC) codes:** LDPC codes have high coding efficiency and can approach the Shannon limit.
### 3.2 Analysis of Bit Error Rate (BER)
Bit Error Rate (BE
0
0
相关推荐
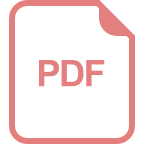
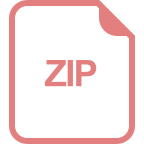
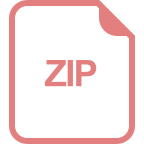
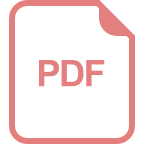
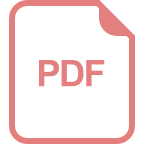
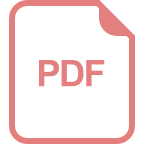
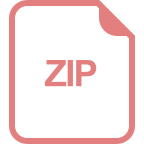
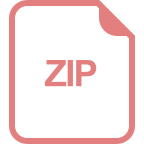
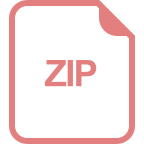