【Practical Exercise】Design and Simulation of a Basic QPSK Modulation System in MATLAB
发布时间: 2024-09-14 06:47:14 阅读量: 46 订阅数: 71 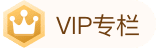
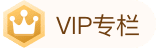
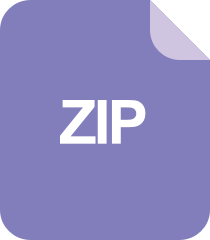
matlab Basic simulation of IEEE802.11a.zip
# 2.1 The Structure and Operation Principle of the QPSK Modulator
The QPSK modulator consists of the following main modules:
- **Data Source:** Produces the binary data stream to be modulated.
- **Serial-to-Parallel Converter:** Converts the serial binary data stream into a parallel binary data stream.
- **Scrambler:** Scrambles the data to reduce the correlation between adjacent symbols.
- **I/Q Modulator:** Modulates the parallel binary data stream onto orthogonal carriers (I and Q).
The basic operating principle of the QPSK modulator is as follows:
1. The data source generates a binary data stream.
2. The serial-to-parallel converter converts the serial data stream into a parallel data stream, where each parallel bit corresponds to two bits of a symbol.
3. The scrambler scrambles the data to improve noise immunity.
4. The I/Q modulator modulates the parallel data stream onto orthogonal carriers. Specifically, for each symbol, the I carrier modulates the first bit, and the Q carrier modulates the second bit.
# 2. The QPSK Modulation Principle
### 2.1 The Structure and Operation Principle of the QPSK Modulator
The QPSK modulator consists of the following components:
- **Data Source:** Produces the data bit stream to be modulated.
- **Binary-to-Quaternary Converter:** Converts the binary data bit stream into a quaternary symbol sequence.
- **Quaternary-to-Phase Converter:** Converts the quaternary symbol sequence into phase shifts.
- **Carrier Oscillator:** Produces a sinusoidal carrier signal.
- **Phase Modulator:** Modulates the phase shifts onto the carrier signal.
The operating principle of the QPSK modulator is as follows:
1. The data source generates a binary data bit stream.
2. The binary-to-quaternary converter converts the binary data bit stream into a quaternary symbol sequence. Each quaternary symbol represents two binary bits.
3. The quaternary-to-phase converter converts the quaternary symbol sequence into phase shifts. Each quaternary symbol corresponds to a specific phase shift (0°, 90°, 180°, 270°).
4. The carrier oscillator generates a sinusoidal carrier signal.
5. The phase modulator modulates the phase shifts onto the carrier signal.
### 2.2 The Mathematical Model of the QPSK Modulated Signal
The mathematical model for the QPSK modulated signal is:
```
s(t) = A cos(2πf_c t + θ(t))
```
Where:
- `s(t)` is the modulated signal
- `A` is the carrier amplitude
- `f_c` is the carrier frequency
- `θ(t)` is the phase shift
The phase shift `θ(t)` is determined by the following formula:
```
θ(t) = 2πk/4, k = 0, 1, 2, 3
```
Where:
- `k` is the quaternary symbol
Therefore, the QPSK modulated signal has four phase states: 0°, 90°, 180°, 270°.
**Code Block:**
```python
import numpy as np
import matplotlib.pyplot as plt
# Carrier frequency
f_c = 1000
# Sampling frequency
fs = 10000
# Data bit stream
data = np.random.randint(0, 2, 1000)
# Binary-to-quaternary conversion
symbols = np.array([int(''.join(map(str, data[i:i+2])), 2) for i in range(0, len(data), 2)])
# Quaternary-to-phase conversion
phases = np.array([2*np.pi*k/4 for k in symbols])
# Carrier signal
carrier = np.cos(2*np.pi*f_c*np.arange(0, 1, 1/fs))
# Phase modulation
modulated_signal = carrier * np.exp(1j*phases)
# Visualization
plt.plot(np.real(modulated_signal))
plt.xlabel('Time (s)')
plt.ylabel('Amplitude')
plt.title('QPSK Modulated Signal')
plt.show()
```
**Code Logic Analysis:**
1. Generate a random binary data bit stream.
2. Convert the binary data bit stream into a quaternary symbol sequence.
3. Convert the quaternary symbol sequence into phase shifts.
4. Generate a sinusoidal carrier signal.
5. Modulate the phase shifts onto the carrier signal.
6. Visualize the modulated signal.
**Parameter Description:**
- `f_c`: Carrier frequency
- `fs`: Sampling frequency
- `data`: Binary data bit stream
- `symbols`: Quaternary symbol sequence
- `phases`: Phase shifts
- `carrier`: Carrier signal
- `modulated_signal`: Modulated signal
# 3. Implementing QPSK Modulation in MATLAB
### 3.1 Setting Up the MATLAB Environment and Data Generation
**Setting Up the MATLAB Environment**
1. Install the MATLAB software.
2. Set the MATLAB working path.
**Data Generation**
1. Generate binary data: Use the `randi` function to generate a random binary sequence.
2. Convert to symbol
0
0
相关推荐
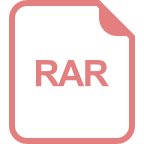
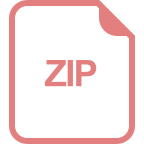
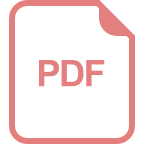
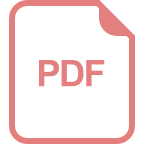
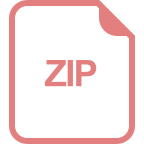
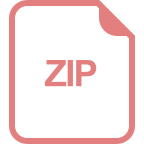
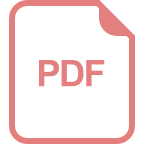
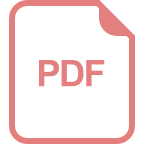